HW-4
docx
keyboard_arrow_up
School
The City College of New York, CUNY *
*We aren’t endorsed by this school
Course
103
Subject
Computer Science
Date
Jan 9, 2024
Type
docx
Pages
4
Uploaded by BaronPuppyPerson1126
HW-4 “Linked Lists”, “Stacks and Queues”, “Recursion”
1. Suppose cursor points to a node in a linked list (using the node definition with member functions called
data and link). What statement changes cursor so that it points to the next node?
A. cursor++;
B. cursor = link( );
C. cursor += link( );
D
. cursor = cursor->link( );
2. Suppose that f is a function with a prototype like this:
void f(________ head_ptr);
// Precondition: head_ptr is a head pointer for a linked list.
// Postcondition: The function f has done some manipulation of
// the linked list, and the list might now have a new head node.
What is the best data type for head_ptr in this function?
A. node
B. node&
C
. node*&
D. node*
3.
We need an expression that will give a random integer between -10 and 10. Select one.
A. (
rand() % 20) – 11; // [-11, 8]
B. (
rand() % 21) – 11; // [-11, 9]
C. (
rand() % 10) – 10; // [-10, -1]
D. (
rand() % 10) – 21;
// [-21, -12]
E
. (
rand() % 21) – 10; // (rand() % n) + k;
returns in range [k, k+(n-1)];
=> [-10, 10]
4. Which of the following stack operations could result in stack underflow?
A. is_empty
B
. pop
C. push
D. Two or more of the above answers
5. Consider the following pseudocode:
declare a stack of characters
while ( there are more characters in the word to read )
{
read a character
push the character on the stack
}
while ( the stack is not empty )
{
write the stack's top character to the screen
pop a character off the stack
}
What is written to the screen for the input "hamburger"?
A. ham
B. hamburger
C
. regrubmah
D. hhaammbbuurrggeerr
6. In the linked list implementation of the stack class, where does the push member function place the new entry on the linked list?
A
. At the head
B. At the tail
C. After all other entries that are greater than the new entry.
D. After all other entries that are smaller than the new entry.
7. One difference between a queue and a stack is:
A. Queues require dynamic memory, but stacks do not.
B. Stacks require dynamic memory, but queues do not.
C
. Queues use two ends of the structure; stacks use only one.
D. Stacks use two ends of the structure, queues use only one. 8. If the characters 'H', 'G', 'F', 'E' are placed in a queue (in that order), and then removed one at a time, in what order will they be removed?
A. EFGH
B. EFHG
C. FEGH
D
. HGFE
9. Suppose we have a circular array implementation of the queue class, with ten items in the queue stored at data[2] through data[11] (including). The CAPACITY is 42. Where does the push member function place the new entry in the array?
A. data[11]
B
. data[12]
C. data[1]
D. data[2]
10. What is the maximum depth of recursive calls a function may make?
A. 1
B. 2
C. n (where n is the argument)
D
. There is no fixed maximum
11. Consider the following function:
void super_write_vertical(int number)
// Postcondition: The digits of the number have been written, // stacked vertically. If number is less than -12, then a negative // sign appears on top. // Library facilities used: iostream.h, math.h
{
if (number < -12)
{
cout << '-' << endl;
super_write_vertical(abs(number));
}
else if (number < 12)
cout << number << endl;
else
{
super_write_vertical(number/10);
cout << number % 10 << endl;
}
}
What values of number are directly handled by the stopping case?
A. number < 0
B. number < 12
C
. number >= -12 && number < 12
D. number > 12
E. number >-10 && number < 12
12. Consider this function declaration:
void quiz(int i)
{
if (i > 1)
{
quiz(i / 2); [6, *][3, *][1, *]
quiz(i / 2); [6, *][3, *][1, *]
}
cout << "*";
}
How many asterisks are printed by the function call quiz(6)?
A. 3
B. 4
C
. 7
D. 8
E. Some other number
13. When the compiler compiles your program, how is a recursive call treated differently than a non-
recursive function call?
A. Parameters are all treated as reference arguments
B. Parameters are all treated as value arguments
C. There is no duplication of local variables
D
. None of the above
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
14. Consider the following function:
void test_b(int n)
{
if (n>0)
test_b(n-2);
cout << n << " ";
}
6 => [6]
4 => [6, 4]
2 => [6, 4, 2]
0 => [6, 4, 2, 0]
What is printed by the call test_b(6)?
A
. 0 2 4 6
B. 0 2 4
C. 2 4 6
D. 6 4 2 0
E. -1 4 2 0 15.
(Optional)
. Write a pseudocode (line by line, no more than 8 lines) for an algorithm that reads an even number of characters. The algorithm then prints the first character, third character, fifth character, and so on. On a second output line, the algorithm prints the second character, fourth character, sixth character, and so on. Use two queues to store the characters. Numerate your steps.
Example:
Your input: “spring”
Program output: “s r n”
“p i g”
SOLUTION:
1.
Read two characters at a time. 2.
Each pair of characters has the first character placed in queue # 1 and the second character placed in queue # 2. 3.
After all the reading is done, print all characters from queue # 1 on one line, and print
all characters from queue # 2 on a second line.
Related Documents
Recommended textbooks for you
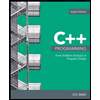
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
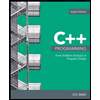
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning