Lab_05-CS171
pdf
keyboard_arrow_up
School
Drexel University *
*We aren’t endorsed by this school
Course
171
Subject
Computer Science
Date
Jan 9, 2024
Type
Pages
16
Uploaded by DukeExplorationRhinoceros41
CS 171 - Lab 5
Professor Mark W. Boady and Professor Adelaida Medlock
Content by Professor Mark Boady
Detailed instructions to the lab assignment are found in the following pages.
•
Complete all the exercises and type your answers in the space provided. What to submit:
•
Lab sheet in PDF •
A screenshot of code and execution for questions 23, 24, 25 Submission must be done via Gradescope
•
Please make sure you have tagged all questions when you upload •
We only accept submissions via Gradescope. Student Name:
Aryan Dixit
User ID (abc123):
14479032
Possible Points: 88
Your score out of 88
:
Lab Grade on 100% scale:
Graded By (TA Signature):
CS 171
Lab 5
Week 5
1.
Critical Thinking Questions
Question 1: 7 points
Closely examine the Python program below.
FYI:
A
looping structure
allows a block of code to be repeated one or more times. A
while
loop is one of
the two looping structures available in Python.
(a)
(2 points) In the Python code, circle / highlight all the lines executed during each iteration of the loop.
•
name = input("Enter your name:")
•
x = 0
•
while (x < 20):
•
print(name)
•
x = x + 1
•
print("The End")
(b)
(1 point) In the IDE, enter and test the code. What does the line of code:
x = x + 1
do?
The line of code
x = x + 1
increments the value of the variable
x
by 1 in each iteration of the loop.
(c)
(1 point) How does the Python interpreter know what lines of code belong to the loop body?
The Python interpreter knows what lines of code belong to the loop body based on their level of
indentation. All the lines indented under the
while
statement with
while (x < 20):
are considered
part of the loop body.
(d)
Every loop structure requires three actions.
Identify the line of code in the Python program that
corresponds to each of the three actions.
(a)
(1 point) Initialize a variable used in the test condition:
Line of code:
x = 0
(b)
(1 point) Include a test condition that causes the loop to end when the condition is false:
# Description: This program prints # a person’s name 20 times
name = input
("Enter your name: ") x = 0 while
(x < 20): print
(name) x = x + 1 print
("The End")
CS 171
Lab 5
Week 5
•
Line of code:
while (x < 20):
(c)
(1 point) Within the loop body, update the variable used in the test condition:
Line of code:
x = x + 1
Question 2: 3 points
Enter and execute the following code. code does.
# Description: This program prints # number from 1 to the value entered by the user
number = int (
input
("Enter a number: ")) x = 1 while
(x <
= number ): if (x % 10 == 0): print
(x) else:
print
(x , end="") x = x + 1 Identify the line of code in the Python program that corresponds to each of the three actions.
(a)
(1 point) Initialize a variable used in the test condition:
Line of code: x = 1
(b)
(1 point) Include a test condition that causes the loop to end when the condition is false:
Line of code: while (x <= number):
(c)
(1 point) Within the loop body, update the variable used in the test condition:
x = x + 1
Question 3: 1 point
The following code should print the numbers from 1 to 10, but it does not print anything.
number = 12 while number <
= 10: print
(number) number = number + 1 Fix the logic error in this code. Edit the above code.
number = 1
while number <= 10:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171
Lab 5
Week 5
print(number)
number = number + 1
Question 4: 3 points
Enter and execute the following code:
number = 1 while number <
= 10: print
(number) number −
= 1
(a)
(1 point) Does the program end?
No, the program will not end because there is no logic to stop the loop. It will result in an infinite loop.
(b)
(2 points) How could you fix this problem?
number = 1
while number <= 10:
print(number)
number += 1
Question 5: 4 points
Enter and execute the following code:
number = 1 while number <
= 10: if number % 2 == 0: print
(number, end= " ") number = number + 1
(a)
(1 point) State the output
2 4 6 8 10
(b)
(1 point) What caused the output to display on one line?
The output is displayed on one line because of the
end=" "
argument in the
print
statement. This
argument specifies the character to be printed at the end of the printed text. In this case, it's a space
character, so the numbers are separated by spaces on the same line.
(c)
(2 points) What control structures are used in this code?
The control structures used in this code are:
•
while
loop: It controls the repetition of the code block as long as the condition
number <= 10
is true.
•
if
statement: It is used to check whether
number
is even (
number % 2 == 0
) and only then prints the
number.
Question 6: 7 points
CS 171
Lab 5
Week 5
The following directions will create a program that prompts the user to enter a number between 1 and 10. As
long as the number is out of range the program re-prompts the user for a valid number. Complete the
following steps to write this code.
(a)
(1 point) Write a line of code that prompts the user for a number between 1 and 10.
number = int(input("Enter a number between 1 and 10: "))
(b)
(1 point) Write a Boolean expression that tests the number the user entered by the code in step (a) to
determine if it is not in range.
out_of_range = (number < 1) or (number > 10)
(c)
(2 points) Use the Boolean expression created in step (b) to write a while loop that executes when the
user input is out of range. The body of the loop should tell the user that they entered an invalid number
and prompt them for a valid number again.
while out_of_range:
print("Invalid number. Please enter a number between 1 and 10.")
number = int(input("Enter a number between 1 and 10: "))
out_of_range = (number < 1) or (number > 10)
CS 171
Lab 5
Week 5
(d)
(1 point) Write the code that prints a message telling the user that they entered a valid number.
print("You entered a valid number.")
(e)
(1 point) Put the segments of code from steps (a) – (d) together. Enter and execute the code. Does it work
properly? If not, correct it and test it again.
number = int(input("Enter a number between 1 and 10: "))
out_of_range = (number < 1) or (number > 10)
while out_of_range:
print("Invalid number. Please enter a number between 1 and 10.")
number = int(input("Enter a number between 1 and 10: "))
out_of_range = (number < 1) or (number > 10)
print("You entered a valid number.")
(f)
(1 point) How many times does the loop execute?
The loop executes until the user enters a valid number, so it will execute at least once, and it may execute
multiple times if the user initially enters an invalid number. The exact number of times it executes
depends on the user's input behavior.
FYI:
A looping structure for which you know the number of times it will execute is known as a count-
controlled loop.
Question 7: 5 points
Sometimes a programmer does not know how many times data is to be entered. For example, suppose you
want to create a program that adds an unknown amount of positive numbers that will be entered by the user.
The program stops adding numbers when the user enters a zero or a negative number. Then the program
prints the total. Before creating this program, review the three actions required for all loops:
(a)
(1 point) Initialize a variable that will be used in the test condition: What will be tested to determine if the
loop is executed? Write a line of code that initializes a variable to be used in the test condition of the loop
for this program. The variable should contain a value entered by the user.
number = int(input("Enter a positive number (enter a negative number or zero to exit): "))
(b)
(1 point) Include a test condition that causes the loop to end when the condition is false: What is the test
condition for the while loop used in this program?
while number > 0:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171
Lab 5
Week 5
(c)
(1 point) Within the loop body, update the variable used in the test condition: Write the code for the loop
body.
number = int(input("Enter a positive number (enter a negative number or zero to exit): "))
(d)
(1 point) Is this a count-controlled loop?
This is not a count-controlled loop. It is condition-controlled because the loop continues until a certain
condition (input being greater than zero) is met.
(e)
(1 point) Complete the program. Enter your fully functioning code below.
total = 0
number = int(input("Enter a positive number (enter a negative number or zero to exit): "))
while number > 0:
total += number
number = int(input("Enter a positive number (enter a negative number or zero to exit): "))
print("The total is:", total)
FYI:
Short-cut operators provide a concise way of creating assignment statements when the variable on the
left-hand side of the assignment statement is also on the right-hand side. The addition short-cut operator (
+=
)
is usually used for incrementing a variable. These are also called compound operators.
Question 8: 5 points
Enter and execute the following code:
number = 1 number += 3 print
(number)
(a)
(1 point) What does the
+=
shortcut operator do?
The
+=
shortcut operator adds the value on the right-hand side to the variable on the left-hand side and
assigns the result back to the variable. In this specific code,
number += 3
adds 3 to the variable
number
,
so it's equivalent to
number = number + 3
. The value of
number
becomes 4.
(b)
(1 point) The code:
x += 5
is equivalent to which of the following lines of code?
x = 5 x = y + 5 x = x + 5 y = x + 5 The code x += 5
is equivalent to the line of code: x = x + 5
. It adds 5 to the variable x
. (c)
Replace the operator
+= with the following shortcut operators and execute the code. Explain what each
operator does.
CS 171
Lab 5
Week 5
(a)
(1 point)
-= The -= operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable. For example, x -= 2 is equivalent to x = x - 2.
(b)
(1 point)
*= The *= operator multiplies the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable. For example, y *= 4 is equivalent to y = y * 4.
(d)
(1 point) Is the following line of code valid: 23 += total
? Why or why not? The line of code
23 += total
is not valid. The
+=
operator can only be used with variables, not with
constant values like
23
. You can use
+=
to update the value of a variable by adding to it, but it can't be
used to modify a constant value.
Question 9: 2 points
Enter and execute the following code:
bonus = 25 salary += bonus print
("Total salary:", salary )
(a)
(1 point) What is the output of the preceding code?
The code as given will produce an error because it tries to add the value of
bonus
to a variable
salary
without first initializing
salary
. The code is attempting to perform an operation on an undefined variable.
(b)
(1 point) Rewrite the code so that it produces valid output.
salary = 1000 # Initialize salary to some value
bonus = 25
salary += bonus
print("Total salary:", salary)
Question 10: 1 point
The following code should print the numbers beginning with 100 and ending with 0. However, it is missing a
line of code. Add the missing code, using the shortcut operator. Draw an arrow to indicate where the code
belongs.
countdown = 100 while countdown >
= 0: print
(countdown) print
("Done!")
countdown = 100 while countdown >= 0:
CS 171
Lab 5
Week 5
print(countdown) countdown -= 1 # <- Missing line: decrement countdown by 1 print("Done!") Question 11: 7 points
Enter and execute the following code:
doAgain = "y" while doAgain == "y" : word = input
("Enter a word:") print
("First letter of " + word + " is " + word [0]) doAgain = input
("Type ’y’ to continue and anything else to quit.") print
("Done!")
(a)
(1 point) What does the program do?
The program repeatedly prompts the user to enter a word. It then prints the first letter of the word and
asks the user if they want to continue. If the user enters 'y', the loop continues, and if they enter anything
else, the program ends.
(b)
(1 point) What is the variable name used to store the user’s input?
The variable used to store the user's input is
word
.
(c)
(1 point) In the print statement, what does
word[0]
represent?
In the print statement,
word[0]
represents the first character (letter) of the word entered by the user.
(d)
(1 point) Change
word[0]
to
word[1]
in the print statement above. What is printed?
If you change
word[0]
to
word[1]
in the print statement, it will attempt to print the second character of
the word. However, this code may result in an "IndexError" if the user enters a word with only one
character. To avoid this error, you can add a check to ensure the word has more than one character before
trying to access
word[1]
.
(e)
(1 point) When does the program end?
The program ends when the user enters anything other than 'y' in response to the prompt "Type 'y' to
continue and anything else to quit."
FYI:
A
sentinel-controlled while loop
is a loop that repeats the loop body until the user enters a pre-specified
value.
(f)
(1 point) Why is the loop in this program an example of a sentinel control loop?
The loop in this program is an example of a sentinel-controlled loop because it uses the sentinel value 'y'
to control the repetition. The loop continues as long as the user enters 'y', and it stops when the user
enters something else.
(g)
(1 point) Examine the print statement in this program:
print ("First letter of " + word + " is " + word[0])
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171
Lab 5
Week 5
What happens if you replace the
+
with a
,
?
print("First letter of " + word + " is " + word[0])
Question 12: 3 points
Examine the code below.
name = "Simone" cost = 3.56 numApples = 89
What type of data is stored in each variable? (integer, floating point, or string)
(a)
(1 point)
name
:
name
stores a string. (b)
(1 point)
cost
:
floating point
(c)
(1 point)
numApples
: stores an integer. FYI:
A variable that can store only the values
True
and
False
is called a
Boolean
variable.
Question 13: 1 point
Given the assignment statement:
foundCost = False
What type of data is stored in
foundCost
?
`foundCost` stores a Boolean data type, specifically the value `False`, which is a boolean literal in Python.
Question 14: 3 points
Enter and execute the following two Python programs.
WHILE LOOP
name = input
("Enter your name: ") x = 0 while x < 20: print
(name) x = x + 1
FOR LOOP
name = input
("Enter your name: ") for x in range (20): print
(name)
(a)
(2 points) What is the output for each program?
The output for both programs will be the same. They both print the name entered by the user 20
times. For example, if the user enters "John," the output will be "John" repeated 20 times.
(b)
(1 point) Both programs produce the same output. Which code fragment is more concise?
CS 171
Lab 5
Week 5
The "FOR LOOP" code is more concise. It accomplishes the same task as the "WHILE LOOP" code with
fewer lines of code and is generally considered more Pythonic and easier to read.
FYI:
The Python predefined function
range()
is used to define a series of numbers and can be used in a FOR
loop to determine the number of times the loop is executed.
Question 15: 5 points
Enter and execute the following code fragments and state the output:
(a)
(1 point)
for
x in range (5): print
(x , end=" ")
0 1 2 3 4
(b)
(1 point)
for
x in range (1 ,5): print
(x , end=" ")
1 2 3 4
(c)
(1 point)
for
x in range
(3 ,20 ,2): print
(x , end=" ")
3 5 7 9 11 13 15 17 19
(d)
(1 point)
numIterations = 6 for x in range
( numIterations ): print
(x , end=" ")
0 1 2 3 4 5
(e)
(1 point)
numIterations = 6 for x in range
(1 , numIterations +1): print
(x , end=" ")
1 2 3 4 5 6
Question 16: 3 points
After examining the five code fragments in question 15, explain how the
range()
function works. Include an
explanation of the arguments.
CS 171
Lab 5
Week 5
The `range()` function in Python is used to generate a sequence of numbers within a specified range. It's
often used in for loops to iterate through a sequence of numbers.
The `range()` function takes one to three arguments:
1. Start: This is the first number in the sequence. It's an optional argument, and if not provided, it defaults
to 0.
2. Stop: This is the number at which the sequence stops, but it is not included in the sequence. This
argument is required.
3. Step: This is an optional argument that specifies the increment between each number in the sequence. If
not provided, it defaults to 1.
For example:
- `range(5)` generates numbers from 0 to 4 (start defaults to 0, stop is 5-1).
- `range(1, 5)` generates numbers from 1 to 4 (start is 1, stop is 5-1).
- `range(3, 20, 2)` generates numbers from 3 to 19 with a step of 2.
So, the `range()` function helps create a sequence of numbers that you can use in loops to iterate through
or perform various operations on.
FYI:
In a
for
loop you can include a list of values in place of the
range()
function.
Question 17: 4 points
Enter and execute the following code.
for
x in [3 ,6 ,9 ,12 ,15 ,18]: print
(x , end=" ")
(a)
(2 points) Rewrite this code using the
range()
function.
for x in range(3, 19, 3):
print(x, end=" ")
(b)
(2 points) Why would you use the
range()
function when you could just list the numbers?
The
range()
function is useful when you have a range of numbers that follows a pattern or has a regular
interval between them. It provides a more concise way to generate a sequence of numbers, especially
when the range is large or the numbers follow a consistent pattern. Using
range()
makes the code more
flexible and easier to maintain because you can easily change the range, start, stop, or step values without
having to manually list all the numbers. It also reduces the chance of errors and saves typing when
working with large ranges.
FYI:
The
str()
function converts its argument into a string.
Question 18: 2 points
Read through the code and determine what it does.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171
Lab 5
Week 5
favorite = input
("Enter your favorite ice cream flavor: ") for x in range (1 ,5): print
( str (x) + "." , favorite , end="\t")
(a)
(1 point) What does the program do?
The program prompts the user to enter their favorite ice cream flavor, and then it uses a for loop to
print the user's favorite ice cream flavor followed by the numbers from 1 to 4, all on the same line and
separated by tabs.
(b)
(1 point) Why is the
str()
function needed in the
print
statement?
The
str()
function is used to convert the number
x
to a string so that it can be concatenated with the
favorite ice cream flavor in the
print
statement. Without
str()
, you can't concatenate a number directly
with a string, so it's necessary to convert
x
to a string to include it in the output.
Question 19: 3 points
Complete the arguments in the following
range()
function so that the code prints the
even
numbers
between 100 and 200, inclusive.
for
x in range
( ??? ) print
(x)
Question 20: 3 points
Complete the arguments in the following
range()
function so that it prints:
5 4 3 2 1 0
.
for
x in range
( ??? ) print
(x)
for x in range(5, -1, -1):
print(x)
FYI:
An accumulator is a variable that stores the sum of a group of values.
Question 21: 5 points
Examine the following code segment.
1
2
3
4
5
total = 0 for x in range (5): number = int (
input
("Enter a number: ")) total += number print
("The total is:" , total )
(a)
(1 point) Why is the variable
total
initialized to
0
in the first line of code?
The variable
total
is initialized to 0 in the first line of code because it is used to
accumulate the sum of the numbers entered by the user. By initializing it to 0, we
start with a clean slate, and we can add the user's input to it in the subsequent
loop.
CS 171
Lab 5
Week 5
(b)
(1 point) Explain what line 3 of the code does.
Line 3 of the code prompts the user to enter a number using the
input()
function
and then converts the user's input to an integer using
int()
. It stores the entered
number in the variable
number
.
(c)
(1 point) Explain what line 4 of the code does.
Line 4 of the code adds the value of the
number
(the user's input) to the
total
variable. It accumulates the sum of the numbers entered by the user in each
iteration of the loop.
(d)
(1 point) How many numbers does the program prompt for?
The program prompts for numbers in the loop with
for x in range(5):
, which
means it prompts for numbers five times.
(e)
(1 point) What is the accumulator in the code segment?
The accumulator in the code segment is the variable
total
. It accumulates the sum
of the numbers entered by the user, and its value keeps increasing with each
input.
Question 22: 1 point
Is it better to use a
for
loop when you know the number of times the loop should be executed or when you
do not know?
It's better to use a for loop when you know the number of times the loop should be executed. For situations
where you have a fixed, predetermined number of iterations, a for loop provides a more concise and
straightforward way to manage the looping process.
Use the Python Interpreter for these questions. Submit one or more screenshots for each. Show your full source
code and an example of the code executing.
Question 23: 3 points
Write a code segment using a FOR loop that prints multiples of 5 from 5 to 500, one on a line.
CS 171
Lab 5
Week 5
Question 24: 4 points
Write code segment that prompts the user for a letter from ’a-z’. As long as the character is not between ’a-z’,
the user should be given a message and prompted again for a letter between ’a-z’. Make sure not to accept
words that start with letters. You should reject a word like robot.
Question 25: 3 points
Write a code segment using a FOR loop that prints all odd numbers between 1 and 100.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
CS 171
Lab 5
Week 5
Related Documents
Recommended textbooks for you
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
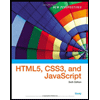
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Recommended textbooks for you
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
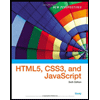
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning