Lab_01-AnswerSheet
pdf
keyboard_arrow_up
School
Drexel University *
*We aren’t endorsed by this school
Course
171
Subject
Computer Science
Date
Jan 9, 2024
Type
Pages
11
Uploaded by DukeExplorationRhinoceros41
CS 171 Computer Programming I
Lab 1
Content by Professor Lisa Olivieri of Chestnut Hill College
Modified for Drexel by Professors Mark Boady and Adelaida Medlock
Detailed instructions to the lab assignment are found in the following pages.
•
Complete all the exercises and type your answers in the space provided.
•
For the last question of this lab you must submit a screenshot your source code
(.py
file) running in Thonny
What to submit:
•
Lab sheet in PDF to Gradescope
•
Questions must be tagged in Gradescope
•
Your lab partner must be added to the submission
•
Only one submission per lab group
Submission must be done via Gradescope
Student Name(s):
Aryan Dixit
User ID(s) (abc123): 14479032
Possible Points:
80
Your score out of 80
:
Lab Grade on 100% scale:
Python Activity 1: Introduction to Python
Critical Thinking Questions (30 points): 1.
(2pts) Enter the Python program shown above. What does it do? The command will force the python above to appear on the screen as "Go!". 2.
(6pts) Type and execute following code. What output is produced? Indicate if there is a problem. a.
print(“Hello, my name is Pat!”)
This code has an issue since it doesn't use the right language. Python requires the standard single (') or double quotations ("). b.
print(Hello, my name is Pat)
Learning Objectives Students will be able to: Content: •
Explain how to display data in Python •
Explain how to create a comment in Python •
Determine the difference between a string literal
and a number •
Explain how to input data using Python •
Explain the meaning and purpose of a variable •
Determine if a variable name is valid •
Explain concatenation and the use of “+” Process: •
Create print statements in Python •
Create Python code that displays results to calculated addition facts •
Discuss problems and programs with all group members •
Create input statements in Python •
Create Python code that prompts the user for data and stores it in a variable •
Create valid and good variable names Prior Knowledge •
Be able to input and execute Python code using the Python IDE FYI: A "string literal"
is a sequence of characters surrounded by double quotation marks (" ").
The issue with this code is that the command's terms are not enclosed in quotes. For Python to recognize that you want to show a piece of code as text, it requires quotations around it. c.
print(“Hello.\nMy name is Pat”) The wrong sort of quotations are used around the text in this quotation, which is a concern. Python requires the standard single (') or double quotations ("). 3.
(2pts) What caused the different output format for samples “a” and “c” in question 2? 4. (8pts) What do you think the following Python statements output? Enter the statements in the interactive mode of the Python interpreter to verify your answers. a.
print(2+5)
b.
print(2*5)
c.
print(“2+5”)
d.
print(“Age:”,20)
a. The output of "print(2+5)" will be "7," which is the result of adding 2 and 5. b. "print(2*5)" will display the multiplication outcome, which is "10". c. Since the text is enclosed in quotes, "print("2+5")" will output the value "2+5". d. The command "print("Age:", 20)" displays the text "Age:" and the value 20. 5.
(8pts) Examine the output for each statement in question 4. a.
What is the difference in the output for the statements in “a” and “c” of question 4? The outcome of adding 2 and 5 is the number "7," which is the output for statement "a." Because statement "c" is enclosed in quotes and doesn't do any calculations, the result is the string "2+5". b.
What caused the difference? Quotes c.
Which statements contain a string literal
? Statement "c" contains a string literal, which is "2+5". Additionally, statement "d" contains a string literal, which is "Age:". d.
What does the comma (,) do in the print statement in part “d” of question 4? How does it affect the spacing of the output?
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
The string "Age:" and the number 20 are separated by a comma (,) in the print statement in component "d". The result will be "Age: 20" with a space between "Age:" and "20" since it automatically inserts a space between the two elements in the output. 6.
(2pts) Examine the following code and its output. What do the first three lines of the program do? The first three lines of the program are comments. They help explain what the program does, who wrote it, and when it was created, but they don't affect how the program runs. 7.
(2pts) In the program from question 6, what would happen if you placed a “#” in front of "Hi, Pat!" becomes a comment when you place a "#" in front of the line. When you execute the program, it won't output "Hi, Pat!" and will instead disregard that line. Application Questions: Use the Python program mode to design and check your work (4 points) 1.
(2 points) Create a Python program containing three statements to print the following output. Copy your program statements in the space below. print("Congratulations!") print("You just created") print("your first Python program.") Output
2.
(2pts) Create a Python program containing two statements that prints the output to the right. Have the program calculate the answers to the two arithmetic problems. Copy your program statements in the space below. print("34 + 123 =", 34 + 123) print("56 * 97 =", 56 * 97) When you run this program, you will see the following output: 34 + 123 = 157 56 * 97 = 5432 Critical Thinking Questions (36 points): 1.
(2pts) Enter and execute the Python program. What is printed on the screen when the Python program is executed? The Python application will initially prompt you with the question "What is your name?" when you launch it. Your name will appear behind "Your name is" and the name you just typed once you hit Enter. To ensure that the program runs properly, you must type "print" with a lowercase "p." FYI: input() and print() are functions
in Python. 2.
(4pts) Examine the first line of Python program: name = input
(
“What is your name? ”
) a.
What appears on the screen when this line of code is executed? The Python program's first line prompts you to enter your name by displaying "What is your name?" on the screen. In order for the software to utilize your name later, it then stores it in a variable named "name." FYI: The words that appear on the screen and tell the user what to enter are known as a prompt
. b.
What happens to the data the user entered? FYI: name = input
(
“What is your name? ”
) The word name in the Python code is a variable
– a name given to a memory location used to store data.
The data (the user's name) is saved in the variable named "name" when the user types their name and pushes Enter. The user's name may then be accessed and used by the application using this variable. 3.
(4pts) Explain the errors that occur when you execute each of the following lines of Python code. a.
name? = input(“What is your name?”) b.
your name = input(“What is your name?”) c.
1st_name = input(“What is your name?”) d.
from = input(“Where were you born?”) a.
question mark (?) is not permitted in variable names, therefore Python is confused and displays an error in the case of name? = input("What is your name?"). b. There is a space after "your" in your name = input("What is your name?"). Python will display an error if a space is present in a variable name. c. The variable name begins with a number (1) in 1st name = input("What is your name?"), which is not permitted. This will cause Python to display an error. d. The term "from" is a special word in Python known as a keyword in the expression from = input("Where were you born?"). Its usage as a variable name is prohibited, hence Python will display an error. 4. (2pts) Examine the errors that occurred when executing the lines of code in question 3. Then examine the following lines of valid code. name2 = input(“What is your name?”) your_name = input(“What is your name?”) yourName = input(“What is your name?”) List the rules that you need to follow to create a valid variable
name. To construct a Python variable name that is valid, follow these guidelines: 1. Uppercase or lowercase letters or the underscore (_) can be used to begin the names of variables.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2. Variable names may follow the initial character with any combination of letters, digits, or underscores. 3. Spaces and other special characters (such as?,!, etc.) are not permitted in variable names. 4. You are not permitted to use special terms that Python currently uses (known as keywords), such as "from," "if," or "while." By adhering to these guidelines, you may make legal variable names such as name2, your name, and yourName. 5. (8pts) Are the following variable names valid
? Are they good
names? Why or why not? 6. (2pts) Execute the following lines of code. Is the output what you would expect? Why or why not? If you run the provided lines of code, an error will be produced instead of the desired result. Python is case-sensitive, which means that it treats letters in uppercase and lowercase differently. The variable is created in the code as name (lowercase "n"), but when it's time to print it, Name (uppercase "N") is used instead. Use the same case for the variable to correct this issue: 7. (10pts) Use the following set of Python statements to answer the questions below. a.
State the output for each of line of code. Variable name Comments about variable name price Valid and good name. It's a simple, clear name that describes the value it represents. costoffirstitem Valid, but not a great name. It's too long and harder to read. A better alternative would be cost_of_first_item
or first_item_cost
. Ic Valid, but not a good name. It's unclear what "Ic" stands for, and it's better to have a more descriptive name. Also, it's recommended to use lowercase for the first character in variable names. firstName Valid and good name. It's clear and easy to understand that it represents someone's first name. In Python, it's common to use underscores instead of camel case, so first_name
would be an even better choice.
1.
‘Your name is Pat.’
2.
‘Your name is Pat.’ (single and double quotes both work for strings)
3.
‘Your name isPat.
’(no space between 'is' and 'Pat.') 4.
‘Your age is 20’
5.
will give an error, because you cannot add a string and a number directly. b. How are the first two print statements different? Does the difference affect the output? The first two print statements use different quotes (double quotes and single quotes) for the string 'Your name is', but this difference does not affect the output, as both types of quotes can be used to define strings in Python. b.
Notice that some statements include a comma (,) between the two literals being printed and some statements use a “+”. Do they produce the same output? Using a comma (,) between two things being printed adds a space between them, while using a "+" combines them directly with no space in between, so they don't always produce the same output. e.
Explain the purpose of the comma. The comma in a print statement is like a friendly helper that separates the things being printed and puts a space between them, making the output look nice and easy to read. e. Why does the last print statement crash the program? What would you do to correct it? The last print statement crashes because it tries to mix words and numbers like apples and oranges; to fix it, we can turn the number into words using str(20)
before combining them. 8. (2pts) State what is displayed on the screen when the following program is executed: FYI: “+” concatenates
two strings. The strings can be string literals or a variable containing string literals.
9.
(2pts) What caused the output in the print statement in question 8 to be printed on more than one line? Application Questions: Use the Python Interpreter to enter your code and check your work (10 points) 1.
(2pts) State a good variable name for an employee’s ID number: A good variable name for an employee's ID number would be employee_id
. 2.
(2pts) Write a line of Python code that prompts the user for the name of their favorite ice cream and stores it in a valid variable name. favorite_ice_cream = input("What is your favorite ice cream? ") 3.
(6pts) Crazy Sentence Program
. Create a program that prompts the user for the name of an animal, a color, the name of a vehicle, and the name of a city. Then print a sentence that contains the user input in the following order: color, animal, vehicle, city. Include the additional words in the sample output as part of your output. Example: Assume the user enters the words: tiger, green, motorcycle, and Wildwood. The output would be: The
green tiger drove the
motorcycle to
Wildwood. Once your program is complete, take a screenshot of the code and output of the program. You may need multiple screenshots to capture all your code. Paste the screenshot below. Your code must
include comments with the names of all group members and the date the code was written.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
# Group members: [list your group members' names here] # Date: [write the date the code was written here] creature = input("Give me an animal's name: ") shade = input("Tell me a color: ") transport = input("What's a vehicle's name? ") town = input("Name a city: ") phrase = "The " + shade + " " + creature + " took the " + transport + " to " + town + "." print(phrase)
Related Documents
Recommended textbooks for you
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
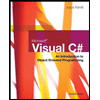
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
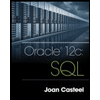
Recommended textbooks for you
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
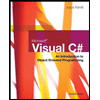
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
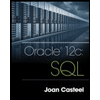