[Coding Exercise] Week 10 - Interactive image processor
pdf
keyboard_arrow_up
School
Simon Fraser University *
*We aren’t endorsed by this school
Course
120
Subject
Computer Science
Date
Oct 30, 2023
Type
Pages
6
Uploaded by xiaofanfan
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
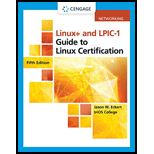
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L
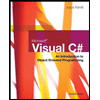
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
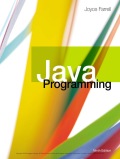
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
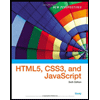
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
Recommended textbooks for you
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageLINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE LMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningMicrosoft Windows 10 Comprehensive 2019Computer ScienceISBN:9780357392607Author:FREUNDPublisher:Cengage
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
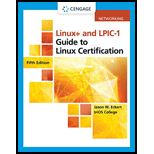
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L
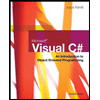
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
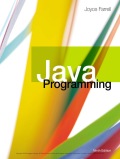
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
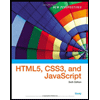
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
Browse Popular Homework Q&A
Q: Determine the intervals of the domain over which the function is continuous.
Select the correct…
Q: determine the forces of all members using the method of joints, indicating their forces in kips,…
Q: Like many structural elements, the rectangle has a strong axis (orientation) and a weak axis.
True…
Q: Consider the same population of mice with two alleles for a digestive enzyme, E
and e. Assume the…
Q: Find a counterexample for the statement.
N =
For every real number N > 0, there is some real number…
Q: Consider a loop branch that branches nine times
in a row, then is not taken once. What is the…
Q: Consider a loop branch that branches nine times
in a row, then is not taken once. What is the…
Q: 2.
# of Absences (in weeks) Exam Grade
1
2
3
4
5
23 014
90
85
95
92
80
Correlation analysis of the…
Q: 2. A ship travels due west for 80 miles. It then travels in a northern direction for 59 miles and…
Q: Set up integrals for both orders of integration. Use the more convenient order to evaluate the…
Q: x2 − 4
Q: The Venn diagram shows sets A, B, C, and the universal set U.
Shade Bn (C'UA) on the Venn diagram.
U…
Q: the original mass of the iron nails was 100 grams after one week the mass was 120
Q: How does decreasing the total energy of the system affect the stability of the pair of atoms in
the…
Q: winter day starting at sunrise.
5. The graph shows the outdoor temperature on a certain…
Q: Write a note on DOM manipulation.
Q: The domain for this problem is some unspecified collection of numbers. Consider the predicate
P(x,…
Q: In the western United States, there are many dry land wheat farms that depend on winter snow and…
Q: s
membrane
C. How does the diagram inform your answer to the question?
D. What is the answer to the…
Q: problem did the American independence leave them
Q: 2.
A system consists of 1 mole of ideal gas is taken through the cycle shown below
from (123). For…
Q: Write three statements to print the first three elements of array runTimes. Follow each statement…
Q: b. Writ
For 6-7, write the equation that models each linear relationship.
6.
ty
18
16
8642064NO
14…
Q: For the diagram shown below find the reactions at each support
Q: Upload a drawing of a graph showing the change in
potential energy (on the y-axis) as two molecules…