CPSC_103_Midterm_2021W1_Solution-1
pdf
keyboard_arrow_up
School
University of British Columbia *
*We aren’t endorsed by this school
Course
103
Subject
Accounting
Date
Apr 3, 2024
Type
Pages
12
Uploaded by AgentBatPerson1076
2021W1 Midterm Answer Key
Question 1
numbers = [2, 3, 2, 7, 4, 3]
tot = 0
for i in numbers:
if is_even(i): # assume is_even(i) is a function that is
# complete and correct (and available for use)
# is_even(i) returns True if i is even
tot = tot + i
tot
8
Question 2
def foo1(x):
return x/2
def foo2(x, y):
y = 20
x == y
x = foo1(y)
y = 3
return x
foo2(6, 5)
10
Page 1 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
Question 3
The Beverage data below (similar to the one you saw in Worksheet 4) records information about
a beverage offered at the cafe, including:
●
Its name, as it appears in the menu
●
Its price
●
Whether or not it is dairy-free
Even though this data definition will run with no errors, there are some errors in the data design.
Identify the line number where each error is found and describe how you would fix each error.
For example, if you believe a line should be removed, write its number and SHOULD BE
REMOVED after it (capitalization does not matter) (i.e., Line 7 should be removed or LINE 7
SHOULD BE REMOVED).
01.
from
typing
import
NamedTuple
02.
Beverage
=
NamedTuple(
'Beverage'
, [(
'name'
,
str
),
03.
(
'price'
,
float
),
04.
(
'dairy_free'
,
str
)])
05.
06.
#interp. a beverage available in the cafe’s menu,
07.
# including its name, price, and whether or not
it is dairy free.
08.
09.
BLACK_COFFEE
=
Beverage(
"Black coffee"
,
2.50
,
"Yes"
)
10.
EARL_GRAY
=
Beverage(
"Earl Gray Tea"
,
3.25
,
"Yes"
)
11.
LONDON_FOG
=
Beverage(
"London Fog"
,
4.75
,
"No"
)
12.
13.
# template based on compound (3 fields)
14.
@typecheck
15.
def
fn_for_drink
(b: Beverage)
-> ...
:
16.
return
...
(b
.
name,
17.
b
.
price)
Line 3: an interval comment is needed to show that price cannot be a negative number
Line 4:
dairy_free
should be a bool, not a str
Lines 9-11: The
“Yes”
should be changed to
True
and the
“No”
should be
False
Line 15: It should be
fn_for_beverage
, not
fn_for_drink
Line 18: Missing a
b.dairy_free
in the data template.
Page 2 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
Question 4
Time to work on a classic RPG game! Design a data type for a character in a game. A
character’s information is comprised of:
●
Its name
●
Its race (one of: human, elf, dwarf, ogre)
●
Its health points (between 0 and 300)
●
Its mana points (between 0 and 300)
The data definition for race is already given to you:
from enum import Enum
Race = Enum("Race", ["HUMAN", "ELF", "DWARF", "OGRE"])
# interp. The possible races for a character in the game
# examples are redundant for enumerations
@typecheck
# Template based on Enumeration (4 cases)
def fn_for_race(r: race) -> ...:
if r == Race.HUMAN:
return …
elif r == Race.ELF:
return …
elif r == Race.DWARF:
return ...
elif r == Race.OGRE:
return ...
Page 3 of 12
No Unauthorized Distribution of Materials
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2021W1 Midterm Answer Key
from typing import NamedTuple
Character = NamedTuple(‘Character’, [(‘name’, str),
(‘race’, Race),
(‘health’, int) # in range [0,300]
(‘mana’, int) # in range [0,300]])
# interp. information about a character in the RPG game,
# including name (name), race (race), health points
# (health), and mana points (mana).
CH1 = Character(“Raghard”, Race.Human, 150, 100)
CH2 = Character(“Wargo”, Race.Ogre, 0, 300)
CH3 = Character(“Oggy”, Race.Dwarf, 300, 0)
# template based on compound (4 fields) and reference rule
@typecheck
def fn_for_character(c: Character) -> ...:
return ...(c.name,
fn_for_race(c.race),
c.health
c.mana)
Page 4 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
Data Definitions Used in Questions 5 and 6
Type = Enum("Type", ["APARTMENT", "HOUSE"])
# interp. Property type. It can be an apartment or a house.
# Examples are redundant for enumerations
# template based on Enumeration
@typecheck
def fn_for_type(t: Type) -> ...:
if t == Type.APARTMENT:
return ...
elif t == Type.HOUSE:
return ...
Property = NamedTuple("Property", [("type", Type),
("square_feet", int), # in range (0,...)
("year", int),
("price", int),
("pending", bool)])
# interp. information about a property, including its type #
(apartment or house), footage, the year it was built,
# price and whether or not it has a pending offer.
P1 = Property(Type.APARTMENT, 625, 2020, 450000, False)
P2 = Property(Type.HOUSE, 1100, 1980, 1350000, False)
P3 = Property(Type.HOUSE, 925, 1995, 1100000, True)
# template based on Compound and reference rule
@typecheck
def fn_for_property(p: Property) -> ...:
return ...(fn_for_type(p.type),
p.square_feet,
p.year,
p.price,
p.pending)
Page 5 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
# List[Property]
# interp. a list of properties in the agency’s inventory
L0 = []
L1 = [P1, P2, P3]
# template based on arbitrary-sized and reference rule
@typecheck
def fn_for_lop(lop: List[Property]) -> ...:
# description of the accumulator
acc = ... # type: …
for p in lop:
acc = ...(fn_for_property(p), acc)
return ...(acc)
Page 6 of 12
No Unauthorized Distribution of Materials
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2021W1 Midterm Answer Key
Question 5
The data definitions on the previous two pages are for a Real Estate agency that deals in
private homes. Each property has a type (apartment or house), square feet, year built, price,
and a status to indicate whether or not an offer is pending on it.
The following function examines a list of properties to determine whether or not it contains a
house that costs at most $1000000 and is of a given footage or greater. Complete the helper
functions and add new ones if needed. You can assume has_cheap_house_min_footage() is
complete and correct.
Be sure to follow the HtDF recipe. You are allowed to assume that the tests for all functions in
this question are present, complete, and correct.
from cs103 import *
@typecheck
def has_cheap_house_min_footage(lop: List[Property], sf: int) -> bool:
"""
Returns True if there is at least one house of footage
equal or greater than sf that costs at most one
million dollars in the list; False otherwise.
"""
# return True # stub
# template copied from List[Property] with 1
# additional parameter
for p in lop:
if is_property_house(p) and has_min_footage(p,
sf) and costs_at_most_one_million(p):
return True
return False
# Complete the helper functions below and add new functions if needed
# Be sure to follow the FULL HtDF recipe
Page 7 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
@typecheck
def is_property_house(p: Property) -> bool:
"""
Returns True if p is a house; False otherwise.
"""
#
return True # stub
# template based on Property
# return is_house(p.type)
@typecheck
def is_house(type: Type) -> bool:
"""
Returns True if type is Type.HOUSE; False otherwise.
"""
# return True # stub
# template based on Type
if type == Type.APARTMENT:
return False
elif type == Type.HOUSE:
return True
@typecheck
def has_min_footage(p: Property, square_footage: int) -> bool:
"""
Returns True if p's square footage (i.e., size) is at
least square_footage large; False otherwise.
"""
#
return True # stub
# template based on Property with additional parameter
return p.square_feet >= square_footage
Page 8 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
@typecheck
def costs_at_most_one_million(p: Property) -> bool:
"""
Returns True if p costs one million dollars or less;
False otherwise.
"""
#
return True # stub
# template based on Property
return p.cost <= 1000000
# You are allowed to assume that all tests required for the
# functions above are present, complete, and correct
Page 9 of 12
No Unauthorized Distribution of Materials
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2021W1 Midterm Answer Key
Question 6
Consider the Real Estate agency data definitions given earlier.
We want to design a function to return all apartments built after a given year and below a given
price.
Here are some functions which may or may not prove useful. It is possible that your answer will
not call every helper function listed in this question. You cannot write any other function for this
question. Only use what you are given.
You do NOT need to provide a complete implementation of the helpers. You only need to
complete find_apartments_by_year_and_price(). You do not need to provide tests for
find_apartments_by_year_and_price().
@typecheck
def find_all_apartments(lop: List[Property]) -> List[Property]:
"""
Returns all apartments in lop.
"""
return []
# stub
@typecheck
def is_property_apartment(p: Property) -> bool:
"""
Returns True if p is an apartment; False otherwise.
"""
# return True # stub
@typecheck
def is_apartment(t: Type) -> bool:
"""
Returns True if t represents an apartment; False
otherwise.
"""
# return True # stub
Page 10 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
@typecheck
def find_properties_in_budget(lop: List[Property], p: int) ->
List[Property]:
"""
Returns all properties with price equal to or below p.
"""
return []
# stub
@typecheck
def is_property_in_budget(p: Property, pr: int) -> bool:
"""
Returns True if the property p has price equal to or
below pr; False otherwise.
"""
# return True # stub
@typecheck
def find_properties_built_after_year(lop: List[Property], y: int) ->
List[Property]:
"""
Returns all properties built strictly after year y.
"""
return []
# stub
@typecheck
def is_property_built_after_year(p: Property, y: int) -> bool:
"""
Returns True if the property p was built strictly
after year y; False otherwise.
"""
# return True # stub
Page 11 of 12
No Unauthorized Distribution of Materials
2021W1 Midterm Answer Key
@typecheck
def find_apartments_by_year_and_price(lop: List[Property], y: int, p:
int) -> List[Property]:
"""
Returns a list of all apartments built after a given
year (y) and below a given price (p).
"""
# return [] # stub
# template based on composition
# 1. filter all apartments
# 2. filter all apartments built after year y
# 3. filter all apartments built after year y with
# price equal or less than price p
# 4. return final list
# Complete the rest of the
# find_apartments_by_year_and_price function
all_apartments = find_all_apartments(lop)
apartments_after_year =
find_properties_built_after_year(all_apartments, y)
apartments_at_most_price =
find_properties_in_budget(apartments_after_year, p)
return apartments_at_most_price
Page 12 of 12
No Unauthorized Distribution of Materials
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
No chatgpt used i will give 5 upvotes typing please
arrow_forward
No chatgpt used i will give 5 upvotes typing please I need 3 answers
arrow_forward
I need typing clear urjent no chatgpt use i will give 5 upvotes
arrow_forward
I need typing clear urjent no chatgpt used i will give 5 upvotes pls full explain
arrow_forward
I need typing clear urjent no chatgpt used i will give 5 upvotes pls full explain
arrow_forward
I need answer typing clear urjent no chatgpt used i will give upvotes
arrow_forward
I need do fast typing clear urjent no chatgpt used i will give 5 upvotes pls full explain
arrow_forward
myedio.com
Question 12
Listen
Use the function f(x)=2x-5
• Find the inverse of f(x).
.
.
Graph f(x) and f(x) and state the domain of each function.
Prove that f(x) and f¹(x) are inverses, both graphically and
algebraically.
ATTACHMENTS
W
Algebra2 U9 UnitTest_Q17
docx
146.32 KB
arrow_forward
typing clear no chatgpt used i will give 5 upvotes
arrow_forward
I need answer typing clear urjent no chatgpt used i will give upvotes all answers pls
arrow_forward
I need doo fast typing clear urjent no chatgpt used i will give 5 upvotes full explanations pls
arrow_forward
I need typing clear urjent no chatgpt use i will give 5 upvotes
full explanation
arrow_forward
Question 3
Listen
What are the values of r and r² for the below table of data?
Hint: Make sure your diagnostics are turned on. Enter the data into L1 and L2.
Click STAT, CALC, and choose option 8: Lin Reg(a+bx).
A
r = -0.862
r2=0.743
B
r=0.673
2=0.820
X
y
5
C
r=0.743
r2=-0.862
8
22
23.9
14
9
14
17
20
5.2
arrow_forward
I need answer typing clear urjent no chatgpt used pls i will give 5 Upvotes.
arrow_forward
I need answer typing clear urjent no chatgpt used i will give upvotes
arrow_forward
I need do fast typing clear urjent no chatgpt used i will give 5 upvotes pls full explain
arrow_forward
typing clear no chatgpt used i will give 5 upvotes
arrow_forward
Question 10
Listen
Based on the piecewise function given below, what is f(2)?
x², x≤ -5
f(x)=x+3, -52
A 5
B 2
C 4
arrow_forward
Topic: Uni x
U2_AS i
F1
Topic Uni X
(@
2
5 ezto.mheducation.com/ext/map/index.html?_con=con&external browser=0&launchUrl=https%253A%252F%252Flms.mheducation.com%252Fmghmiddleware%2
Required A Required B
Required:
a. What are the amount and character of the gain that Javens will recognize in year 0?
b. What amount and character of the gain will Javens recognize in years 1 through 6?
x
Complete this question by entering your answers in the tabs below.
Recognized Gain
Character of Recognized Gain:
Description
Ordinary Gain
§1231 gain
F2
M Question X M Question X
In year O, Javens Incorporated sold machinery with a fair market value of $500,000 to Chris. The machinery's original basis was
$394,000 and Javens's accumulated depreciation on the machinery was $60,000, so its adjusted basis to Javens was $334,000. Chris
paid Javens $50,000 immediately (in year O) and provided a note to Javens indicating that Chris would pay Javens $75,000 a year for
six years beginning in year 1.
What are the…
arrow_forward
Please Solve In 10mins
Both Questions
arrow_forward
Question 3
Listen
What are the values of r and r2 for the below table of data?
Hint: Make sure your diagnostics are turned on. Enter the data into L1 and L2.
Click STAT, CALC, and choose option 8: LinReg(a+bx).
A
r=-0.862
r²=0.743
B
r=0.673
2=0.820
C r=0.743
2=-0.862
X
5
22
8
23.9
9
14
14
17
77
20
20
52
5.2
arrow_forward
I need typing clear urjent no chatgpt used i will give 5 upvotes pls full explain
arrow_forward
I need do fast typing clear urjent no chatgpt used i will give 5 upvotes pls full explain
arrow_forward
I need answer typing clear urjent no chatgpt used i will give upvotes
arrow_forward
Q#2 Submit this question as one CPP files
Write a complete C++ program. In this program you have to write 3 functions in addition to the main function.
1. Function #1:
Write function throwDice that generates a random number between 1 and 6. The function should return the generated
number.
int throwDice();
1. Function #2:
Write function userGuess that asks the user to enter a valid number between 1 and 6. The function should ask the user to
re-enter as long as the input is not valid. The function should return the valid input of the user.
int userGuess();
1. Function #3:
Write function decide that receives as input parameters two integers, the first parameter dice represents the dice value
and the second parameter userG represents a user guess. This function should return true if userG and dice are equal;
otherwise, it should return false.
bool decide(int dice, int userG);
Write a complete program to do the following in the main:
1. Call the function dice.
2. Call the function…
arrow_forward
Only typed solution
arrow_forward
No chatgpt used i will give 5 upvotes typing please
arrow_forward
I need both answers pls explanation I need answer typing clear urjent no chatgpt used i will give upvotes
arrow_forward
myFunc = function (x, y = 2)
{z = 7
}
z+x^2+y
Assuming that this was the first thing entered in a new R session, if the next command entered is z+1, what is the output?
O 8.
● An error, z does not exist.
O 10.
O 7.
arrow_forward
What is the result of inputting lF(5>9,ok,error) into a cell?
#NAME?
ok
error
arrow_forward
No chatgpt used i will give 5 upvotes typing please
arrow_forward
I need do fast typing clear urjent no chatgpt use i will give 5 upvotes full explanation pls give
arrow_forward
M You x O File: xLA File x + Ass X
Q App x
Q Rev x
O Que X
202 X
C [Th X
O ISB X
E Can x
to.mheducation.com/ext/map/index.html?_con=con&external_browser=0&launchUrl=https%253A%252F%252Fnewconnect.mheducation.com%25
DuTube
A Maps
f Login - Rowan Uni.
A My Drive - Google.
ID ID.me | Simple, Se. A Division of Unemp..
plied C
Class Principles of..
omework- Assignment 1
Saved
Help
On June 30, Sharper Corporation's stockholders' equity section of its balance sheet appears as follows before any stock
dividend or split. Sharper declares and immediately distributes a 50% stock dividend.
Common stock-$10 par value, 60,000 shares issued and
outstanding
Paid-in capital in excess of par value, common stock
Retained earnings
$ 600,000
250,000
685,000
Total stockholders' equity
$ 1,535,000
(1) Prepare the updated stockholders' equity section after the distribution is made.
(2) Compute the number of shares outstanding after the distribution is made.
Complete this question by entering your answers in…
arrow_forward
supposed S= 9 + 9/1.07 + 9/1.07^2 + ...+ 9/1.07^961, Find Value of S. (please break down in step by step)
arrow_forward
hi im looking at the solution above and have a quuestion-im a littlepuzzled on exactly how i should be plugging in the numbersfor example, the chart liists Time (ln2/(ln(1+r)) but how exactly should i type this in my calculatorto get =l3/J3? maybe im overthinking it
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
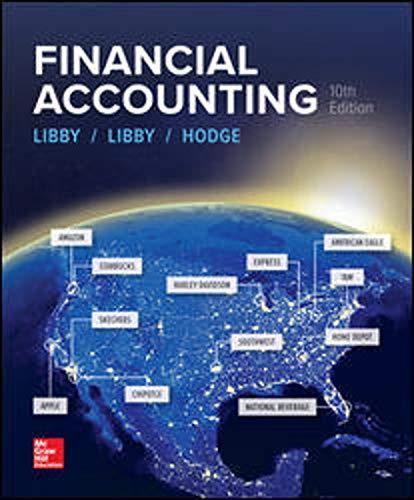
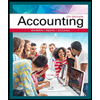
Accounting
Accounting
ISBN:9781337272094
Author:WARREN, Carl S., Reeve, James M., Duchac, Jonathan E.
Publisher:Cengage Learning,
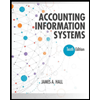
Accounting Information Systems
Accounting
ISBN:9781337619202
Author:Hall, James A.
Publisher:Cengage Learning,
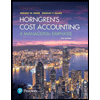
Horngren's Cost Accounting: A Managerial Emphasis...
Accounting
ISBN:9780134475585
Author:Srikant M. Datar, Madhav V. Rajan
Publisher:PEARSON
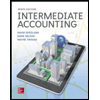
Intermediate Accounting
Accounting
ISBN:9781259722660
Author:J. David Spiceland, Mark W. Nelson, Wayne M Thomas
Publisher:McGraw-Hill Education
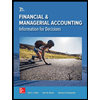
Financial and Managerial Accounting
Accounting
ISBN:9781259726705
Author:John J Wild, Ken W. Shaw, Barbara Chiappetta Fundamental Accounting Principles
Publisher:McGraw-Hill Education
Related Questions
- I need do fast typing clear urjent no chatgpt used i will give 5 upvotes pls full explainarrow_forwardmyedio.com Question 12 Listen Use the function f(x)=2x-5 • Find the inverse of f(x). . . Graph f(x) and f(x) and state the domain of each function. Prove that f(x) and f¹(x) are inverses, both graphically and algebraically. ATTACHMENTS W Algebra2 U9 UnitTest_Q17 docx 146.32 KBarrow_forwardtyping clear no chatgpt used i will give 5 upvotesarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- AccountingAccountingISBN:9781337272094Author:WARREN, Carl S., Reeve, James M., Duchac, Jonathan E.Publisher:Cengage Learning,Accounting Information SystemsAccountingISBN:9781337619202Author:Hall, James A.Publisher:Cengage Learning,
- Horngren's Cost Accounting: A Managerial Emphasis...AccountingISBN:9780134475585Author:Srikant M. Datar, Madhav V. RajanPublisher:PEARSONIntermediate AccountingAccountingISBN:9781259722660Author:J. David Spiceland, Mark W. Nelson, Wayne M ThomasPublisher:McGraw-Hill EducationFinancial and Managerial AccountingAccountingISBN:9781259726705Author:John J Wild, Ken W. Shaw, Barbara Chiappetta Fundamental Accounting PrinciplesPublisher:McGraw-Hill Education
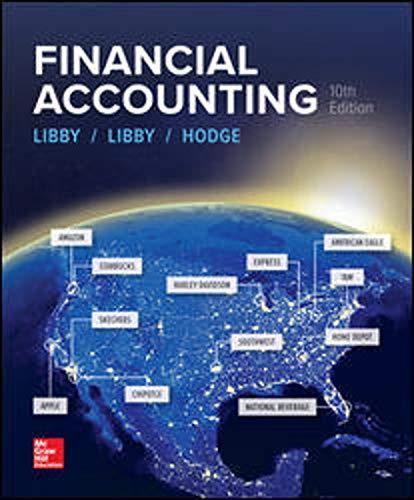
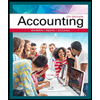
Accounting
Accounting
ISBN:9781337272094
Author:WARREN, Carl S., Reeve, James M., Duchac, Jonathan E.
Publisher:Cengage Learning,
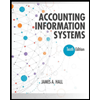
Accounting Information Systems
Accounting
ISBN:9781337619202
Author:Hall, James A.
Publisher:Cengage Learning,
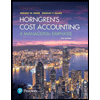
Horngren's Cost Accounting: A Managerial Emphasis...
Accounting
ISBN:9780134475585
Author:Srikant M. Datar, Madhav V. Rajan
Publisher:PEARSON
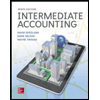
Intermediate Accounting
Accounting
ISBN:9781259722660
Author:J. David Spiceland, Mark W. Nelson, Wayne M Thomas
Publisher:McGraw-Hill Education
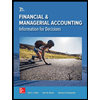
Financial and Managerial Accounting
Accounting
ISBN:9781259726705
Author:John J Wild, Ken W. Shaw, Barbara Chiappetta Fundamental Accounting Principles
Publisher:McGraw-Hill Education