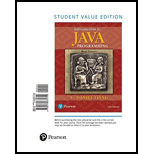
Show the output of the following
public class Test {
publ1c static void main(String[] args)
Circle circle1 =new Circle (1);
Circle circle2 =new Circle (2);
swap1(circle1, circle2);
System.out.println("After swap1: circle1 =" + circle1.radius+ " circle2 = " + circle2.radius);
swap2(circle1 , circle2);
System.out.println("After swap2 : circle1 = " + circle1.radius+ "circle2 =" + circle2.radius);
}
public static void swap1(Circle x, Circle y) {
Circle temp= x;
X = y;
y = temp;
public static void swap2(Circle x, Circle y) {
double temp = x.radius;
x.radius = y.radius;
y.radius = temp;
}
}
class Circle {
double radius;
Circle(double newRadius)
radius = newRadius;
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Modern Database Management
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- In C++ class rectangleType { public: void setLengthWidth(double x, double y); //Sets the length = x; width = y; void print() const; //Output length and width double area(); //Calculate and return the area of the rectangle (length*width) double perimeter(); //Calculate and return the perimeter (length of outside boundary of the rectangle) private: double length; double width; }; Print out the area of the rectangle.arrow_forwardusing System; class Program { publicstaticvoid Main(string[] args) { int number = 1,i; while (number <= 88) { Console.WriteLine(number); number = number + 2; { Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88) Console.WriteLine("Random Number between 1 and 88 is "+randNo); } } } } } how can this program be fixed to choose three random numbers in C# between 1-88 instead of just one random number?arrow_forward// Makes String comparisons public class DebugSeven1 { publicstaticvoidmain(String[] args) { String name1 ="Roger"; String name2 ="Roger"; String name3 ="Stacy"; if(name1.equals(name2)) System.out.println(name1 + " and " + name2 + " are the same"); if(name1.equals(name3)) System.out.println(name1 + " and " + name2 + " are the same"); if(name1.equals("roger)); System.out.println(name1 + " and 'roger' are the same"); if(name1.equals("Roger")); System.out.println(name1 + " and 'Roger' are the same"); } }arrow_forward
- // SuperMarket.java - This program creates a report that lists weekly hours worked // by employees of a supermarket. The report lists total hours for // each day of one week. // Input: Interactive // Output: Report. import java.util.Scanner; public class SuperMarket { public static void main(String args[]) { // Declare variables. final String HEAD1 = "WEEKLY HOURS WORKED"; final String DAY_FOOTER = " Day Total "; // Leading spaces are intentional. final String SENTINEL = "done"; // Named constant for sentinel value. double hoursWorked = 0; // Current record hours. String hoursWorkedString = ""; // String version of hours String dayOfWeek; // Current record day of week. double hoursTotal = 0; // Hours total for a day. String prevDay = ""; // Previous day of week. boolean done = false; // loop control Scanner input = new…arrow_forwardusing System; class main { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { int i; Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo[i]); } } } } This code is supposed to count from 1-88 and then select three random numbers from the list but instead it generates an infinite loop of random numers between 1-88. how can it be fixed?arrow_forwardpublic class Course{String name;String dept;int number;public Course (String n, String d, int n){name = n;dept = d;number = n;}public String toString(){return "Course " + name + " in " + dept + " #: " + number;}public static void main (String [] args){Scanner in = new Scanner(System.in);add 10 lines using the scanner and saving them as strings The input for each line will be entered a String, a space, another String, and an int. Parse the input string into those three items and make an instance of Course. Add the new Course instance to a generic ArrayList of Course. print out each Course in the ArrayList.arrow_forward
- in this code, the integer on each line shows the line number that is not part of code: what are the parameters of sum: ____ 1 public static int sum(int x, int y){ 2 int z = x +y; 3 return z; 4 } 5 public static void main(String[] args){ 6 … 7 sum(a+2, b); 8 … 9 }arrow_forwardQuestion public class MyProgram { if (h 18) { public static void main(String[] args) { int h=10, t-22; System.out.print ("Good "); } } else } } What is the output displayed by the following lines of code?" System.out.print ("Morning"); t = t + 2; if (t > 30) System.out.println(" Every one!"); System.out.println("The Your answer system.out.print("Evening"); t = t + 5; temperature is " System.out.println(" Brink water?"); Degree.");arrow_forward10. public static void methodl (int i, int num) { for (int j = 1; j <= i; j++) { " "); System.out.print (num + num *= 2; } System.out.println(); } public static void main(String[] args) { int i = 1; while (i <= 6) { methodl (i, 2); i++; }arrow_forward
- Print "userNum1 is negative." if userNum1 is less than 0. End with newline. Assign userNum2 with 4 if userNum2 is greater than 9. Otherwise, print "userNum2 is less than or equal to 9.". End with newline. 1 import java.util.Scanner; 2 public class UserNums { public static void main (String [] args) { int userNum1; int userNum2; 4 6. 7 Scanner input userNum1 = 8. = new Scanner(System.in); input.nextInt(); input.nextInt(); 9. 10 userNum2 11 12 V* Your code goes here */ 13 System.out.println("userNum2 is " } 14 + userNum2); 15 16 17 }arrow_forward1- public class MathF{ public static void main(String[] args) 3 {char[] grade%=new char[4]; grade[1]='B'; 5 grade[3]='C'; 6 Integer d1= new Integer(6); Integer d2= new Integer(10); 8 if(d1!= d2){ System.out.println("grades do not match "); System.out.println("Result: "+Math.sqrt(d1+d2)); 11 } 12 else 13- { System.out.println("Marks are same"); 14 for (int i-0;i<4;i++) 15 System.out.println(grade[i]); } 16 }} Answer:arrow_forwardFill in the blanks public class Test { public String name; public static void main(String[]args) { Test obj = new Test(); System.out.println(________.name); What should be the code in the blank part? Blank is ________arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
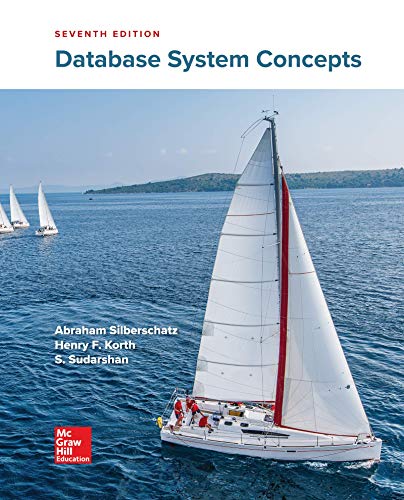
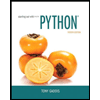
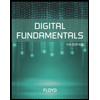
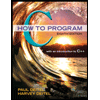
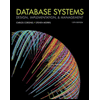
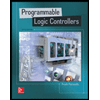