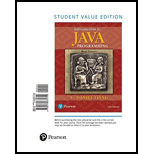
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 9.10, Problem 9.10.1CP
Explanation of Solution
Difference between Primitive type and Reference type:
Primitive type | Reference type |
These are the basic types of data like byte, short, int, long, float, double, boolean, char. | These are the instantiable class as well as arrays: string, scanner, random, die, int[], string[], and soon. |
Primitive variables store primitive values. | These variables store the address. |
Primitive type always contains a value. It can never be null | Reference type can contain null value. |
If primitive variable of type int is created but is not initialized with any value then by default it would be 0. | In this case the default value is null which means no reference is assigned to it. |
Program:
//class definition
public class Test {
// define main function
public static void main(String[] args) {
// create an object
Count myCount = new Count();
// data member
int times = 0;
for (int i = 0; i < 100; i++)
increment(myCount, times);
// accessing member through reference variable
System...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The parameters received by function are .called actual parameters True O False O n
int p =5 , q =6;
void foo ( int b , int c ) {
b = 2 * c ;
p = p + c ;
c = 1 + p ;
q = q * 2;
print ( b + c );
}
main () {
foo (p , q );
print p , q ;
}
Explain and print the output of the above code when the parameters to the foo function are passed by value. Explain and print the output of the above code when the parameters to the foo function are passed by reference. Explain and print the output of the above code when the parameters to the foo function are passed by value result. Explain and print the output of the above code when the parameters to the foo function are passed by name.
Write the functions with the following headers:# Return the reversal of an integer, e.g. reverse(456) returns# 654def reverse(number):# Return true if number is a palindromedef isPalindrome(number):
Use the reverse function to implement isPalindrome. A number is a palindrome if its reversal is the same as itself. Write a test program that prompts the user to enter an integer and reports whether the integer is a palindrome.
Chapter 9 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 9.3 - Prob. 9.3.1CPCh. 9.3 - How do you define a class?Ch. 9.3 - How do you declare an objects reference variable?Ch. 9.3 - How do you create an object?Ch. 9.4 - Prob. 9.4.5CPCh. 9.4 - When will a class have a default constructor?Ch. 9.5 - Which operator is used to access a data field or...Ch. 9.5 - What is an anonymous object?Ch. 9.5 - Prob. 9.5.3CPCh. 9.5 - Is an array an object or a primitive-type value?...
Ch. 9.5 - Prob. 9.5.5CPCh. 9.5 - What is wrong in the following code? 1. class Test...Ch. 9.5 - Prob. 9.5.7CPCh. 9.6 - Prob. 9.6.1CPCh. 9.6 - Prob. 9.6.2CPCh. 9.6 - Which packages contain the classes Date, Random,...Ch. 9.7 - Suppose the class F is defined in (a). Let f be an...Ch. 9.7 - Prob. 9.7.2CPCh. 9.7 - Can you invoke an instance method or reference an...Ch. 9.9 - Prob. 9.9.1CPCh. 9.9 - Prob. 9.9.2CPCh. 9.9 - In the following code, radius is private in the...Ch. 9.10 - Prob. 9.10.1CPCh. 9.10 - Show the output of the following program: public...Ch. 9.10 - Show the output of the following code:Ch. 9.10 - Prob. 9.10.4CPCh. 9.11 - What is wrong in the following code? 1public class...Ch. 9.12 - If a class contains only private data fields and...Ch. 9.12 - If all the data fields in a class are private and...Ch. 9.12 - Is the following class immutable? public class A {...Ch. 9.13 - What is the output of the following program?...Ch. 9.14 - Prob. 9.14.2CPCh. 9.14 - Prob. 9.14.3CPCh. 9 - (The Rectangle class) Following the example of the...Ch. 9 - (The Stock class) Following the example of the...Ch. 9 - (Use the Date class) Write a program that creates...Ch. 9 - Prob. 9.4PECh. 9 - (Use the GregorianCa1endar class) Java API has the...Ch. 9 - (Stopwatch) Design a class named StopWatch. The...Ch. 9 - (The Account class) Design a class named Account...Ch. 9 - (The Fan class) Design a class named Fan to...Ch. 9 - (Geometry: n-sided regular polygon) In an n-sided...Ch. 9 - Prob. 9.10PECh. 9 - (Algebra: 2 2linear equations) Design a class...Ch. 9 - (Geometry: intersecting point) Suppose two line...Ch. 9 - (The Location class) Design a class named Location...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- For parameter passing, call by constant reference is for the function if the value of the actual parameter isn't changed and does no copying. Group of answer choices True Falsearrow_forwardimplement pass-by-value parameter passing method.USE C LANGUAGEarrow_forwardINSTRUCTIONS: Write a C++ script/code to do the given problems. MOVIE PROBLEM: Write a function that checks whether a person can watch an R18+ rated movie. One of the following two conditions is required for admittance: The person is atleast 18 years old. • They have parental supervision. The function accepts two parameters, age and isSupervised. Return a boolean. Example: acceptIntoMovie(14, true) → true acceptIntoMovie(14, false) → falsearrow_forward
- (Financial application: payroll) Write a Java program that reads the following information and prints a payroll statement:arrow_forwardThe parameters passed to function are .called formal parameters True O Fales Oarrow_forwardModify the program so that it does the following: Performs a function that gets the area of a rectangle. The function must receive two parameters (decimal numbers) that represent the base and height of the rectangle and must return the calculated value (decimal number). Perform a second function that obtains the total area of a rectangular prism with a rectangular base. The total area of such a prism is equal to the sum of the areas of each of its faces. It uses calls to the previous function for this calculation. Call this last function in the main with user data. Execution example Give me the base: 21.3 Give me the height: 10 Give me the depth: 2.0 The total area of the prism is: 551.2arrow_forward
- C++ programming Language Write a program that converts a number entered in Roman numerals todecimal form. Program should consist of a class, say romanType. Anobject of romanType should do the following:a. Store the number as a Roman numeral.b. Convert and store the number into decimal form.c. Print the number as a Roman numeral or decimal number as requested by the user. (Write two separate functions—one to print the number as aRoman numeral and the other to print the number as a decimal number.)The decimal values of the Roman numerals are:M 1000D 500C 100L 50X 10V 5I 1Remember, a larger numeral preceding a smaller numeral means addition,so LX is 60. A smaller numeral preceding a larger numeral means subtraction, so XL is 40. Any place in a decimal number, such as the 1s place, the10s place, and so on, requires from zero to four Roman numerals. (The program must include implementation files, .cpp and .h )arrow_forward(Learning Objective: students will be able to apply their knowledge of the built-in random package to generate simulations of simple phenomena.) Write a function: • dicesim(D1,D2,trials) that takes as input the number of sides on die 1 (D1) and die2 (D2) and the number of trials. Your function should repeatedly sum pairs of random numbers between 1 and D1 and 1 and D2 and keep track of how many times each sum occurs. The function returns a numpy array with the fraction each sum of rolls occured. Since the numbers are chosen at random, the fractions will differ some from run to run. One run of the function print(p22.dicesim(6,6,10000)) resulted in: [0. 0. 0.0259 0.0615 0.0791 0.1086 0.139 0.1633 0.1385 0.114 0.0833 0.0587 0.0281] or displayed using the code from Section 16.1.1.: PMF of X Note: you should submit a file with only the standard comments at the top and the function. The grading scripts will then import the file for testing.arrow_forwarda. Differentiate between implicit type conversiona nd Explicit type conversion with the help of example. b. What is the use of continue statement exaplin with appropriate example.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
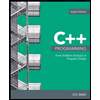
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning