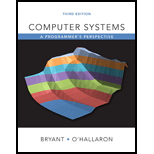
Concept explainers
Explanation of Solution
Perform Next-fit search instead of First-fit search:
In the “Section 9.9.12 (mm.c)”, remove the lines that starts from “/* $begin mmfirstfit */” and ends with “return NULL; /* No fit */”.
Add the following highlighted code to the “Section 9.9.12 (mm.c)”. The modified “mm.c” file is as follows:
/* Global variables */
static char *heap_listp = 0; /* Pointer to first block */
// Rover for Next fit
static char *rover;
/* Function prototypes for internal helper routines */
static void *extend_heap(size_t words);
heap_listp += (2 * WSIZE);
/* $end mminit */
// Assign heap_listp to rover
rover = heap_listp;
/* $begin mminit */
/* Extend the empty heap with a free block of CHUNKSIZE bytes */
bp = PREV_BLKP(bp);
}
/* $end mmfree */
// If condition to check the rover is not representing the free block
if ((rover > (char *)bp) && (rover < NEXT_BLKP(bp)))
// Assign bp to rover
rover = bp;
/* $begin mmfree */
return bp;
}
{
/* Next fit search instead of first fit search*/
// Assign rover to the character pointer oldrover
char *oldrover = rover;
/* Finding next fit using for loop */
// For loop to search from rover to the end of the list
for (; GET_SIZE(HDRP(rover)) > 0; rover = NEXT_BLKP(rover))
// If condition to check allocation and size of rover
if (!GET_ALLOC(HDRP(rover)) && (asize <= GET_SIZE(HDRP(rover))))
// Return rover
return rover;
// For loop to search from start of list to old rover
for (rover = heap_listp; rover < oldrover; rover = NEXT_BLKP(rover))
// If condition to check allocation and size of rover
if (!GET_ALLOC(HDRP(rover)) && (asize <= GET_SIZE(HDRP(rover))))
// Return rover
return rover;
// Otherwise return null
return NULL;
}
/* $end mmfirstfit */
Filename: main.c
// Include libraries
#include <stdio.h>
#include <stdlib.h>
#include <assert.h>
// Include required header files
#include "csapp...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- what is a feature in the Windows Server Security Compliance Toolkit, thank you.arrow_forwardYou will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forward
- Show all the workarrow_forward[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}. 2) [12 marks] Consider the following languages over the alphabet ={a ,b}, (i) The language of all words that begin and end an a (ii) The language where every a in a word is immediately followed by at least one b. (a) Express each as a Regular Expression (b) Draw an FA for each language (c) For Language (i), draw a TG using at most 3 states (d) For Language (ii), construct a CFG.arrow_forwardQuestion 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
- Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.arrow_forwardCan I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
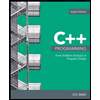
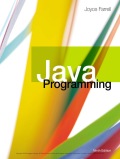
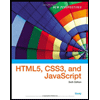
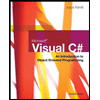