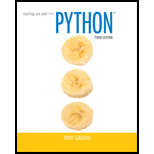
Starting Out with Python (3rd Edition)
3rd Edition
ISBN: 9780133582734
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 9, Problem 20SA
Explanation of Solution
Sets:
- • The set is an unordered collection of unique elements.
- • The set may contain different types of data; it can be numbers, characters, strings, and also class objects.
- • In Python, “set()” method is used to create the sets.
Subset:
- • If two sets are given, then if one set contains some or all the elements of other set, then that set is called the subset of other set.
- • The “issubset()” method can be used to check whether one set is a subset of the other.
Syntax:
In Python, the method “issubset()” can be used to check subset, and it can expressed as follows:
set1.issubset(set2)
Program:
#create the set set1 with five elements
set1 = set([100, 200, 300, 400, 500])
#create the set set2 with three elements
set2 = set([200, 400, 500])
#check whether set1 is subset of set2
print('set1 is subset of set2:',set1.issubset(set2))
#check whether set2 is subset of set1
print('set2 is subset of set1:',set2.issubset(set1))
Explanation of the program:
In the above Python code,
- • The first statement is to create the set “set1” using the “set()” function; the “set1” contains “100”, “200”, “300”, “400”, and “500”.
- • The second statement is to create the set “set2” using the “set()” function; the “set2” contains “200”, “400”, and “500”.
- • The third statement is to check whether “set1” is a subset of “set2”; if the result is “true”, it means “set1” is a subset of “set2”.
- • Here, the result is “false”; thus, “set1” is not a subset of “set2”.
- • The fourth statement is to check whether “set2” is a subset of “set1”; if the result is “true”, it means “set2” is a subset of “set1”.
- • Here, the result is “true”; thus, “set2” is a subset of “set1”...
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
I help understanding this question
d'y + 4dy +3y = a, Initial Conditions: y(0) = 5 & y'(0)=0
Where a = 10
a) Find y(t) =yh(t) +yp(t) in time domainIs the system over-damped, under-damped, or critical?
b) Find y(t) using Laplace Transforms
Given f(t)=a sin(ßt)
a = 10 & ß = 23
Find the Laplace Transform using the definition F(s) = ∫f(t)e-stdt
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Chapter 9 Solutions
Starting Out with Python (3rd Edition)
Ch. 9.1 - An element in a dictionary has two parts. What are...Ch. 9.1 - Which part of a dictionary element must be...Ch. 9.1 - Suppose ' start' : 1472 is an element in a...Ch. 9.1 - Suppose a dictionary named employee has been...Ch. 9.1 - What will the following code display? stuff = {1 :...Ch. 9.1 - Prob. 6CPCh. 9.1 - Suppose a dictionary named inventory exists. What...Ch. 9.1 - What will the following code display? stuff = {1 :...Ch. 9.1 - What will the following code display? stuff = {1 :...Ch. 9.1 - What is the difference between the dictionary...
Ch. 9.1 - Prob. 11CPCh. 9.1 - Prob. 12CPCh. 9.1 - What does the values method return?Ch. 9.2 - Prob. 14CPCh. 9.2 - Prob. 15CPCh. 9.2 - Prob. 16CPCh. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - Prob. 22CPCh. 9.2 - After the following statement executes, what...Ch. 9.2 - After the following statement executes, what...Ch. 9.2 - Prob. 25CPCh. 9.2 - Prob. 26CPCh. 9.2 - After the following code executes, what elements...Ch. 9.2 - Prob. 28CPCh. 9.2 - After the following code executes, what elements...Ch. 9.2 - After the following code executes, what elements...Ch. 9.2 - After the following code executes, what elements...Ch. 9.2 - Prob. 32CPCh. 9.3 - Prob. 33CPCh. 9.3 - When you open a file for the purpose of saving a...Ch. 9.3 - When you open a file for the purpose of retrieving...Ch. 9.3 - Prob. 36CPCh. 9.3 - Prob. 37CPCh. 9.3 - Prob. 38CPCh. 9 - You can use the __________ operator to determine...Ch. 9 - You use ________ to delete an element from a...Ch. 9 - The ________ function returns the number of...Ch. 9 - You can use_________ to create an empty...Ch. 9 - The _______ method returns a randomly selected...Ch. 9 - The __________ method returns the value associated...Ch. 9 - The __________ dictionary method returns the value...Ch. 9 - The ________ method returns all of a dictionary's...Ch. 9 - The following function returns the number of...Ch. 9 - Prob. 10MCCh. 9 - Prob. 11MCCh. 9 - This set method removes an element, but does not...Ch. 9 - Prob. 13MCCh. 9 - Prob. 14MCCh. 9 - This operator can be used to find the difference...Ch. 9 - Prob. 16MCCh. 9 - Prob. 17MCCh. 9 - The keys in a dictionary must be mutable objects.Ch. 9 - Dictionaries are not sequences.Ch. 9 - Prob. 3TFCh. 9 - Prob. 4TFCh. 9 - The dictionary method popitem does not raise an...Ch. 9 - The following statement creates an empty...Ch. 9 - Prob. 7TFCh. 9 - Prob. 8TFCh. 9 - Prob. 9TFCh. 9 - Prob. 10TFCh. 9 - What will the following code display? dct =...Ch. 9 - What will the following code display? dct =...Ch. 9 - What will the following code display? dct =...Ch. 9 - What will the following code display? stuff =...Ch. 9 - How do you delete an element from a dictionary?Ch. 9 - How do you determine the number of elements that...Ch. 9 - Prob. 7SACh. 9 - What values will the following code display? (Dont...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - After the following statement executes, what...Ch. 9 - What will the following code display? myset =...Ch. 9 - After the following code executes, what elements...Ch. 9 - Prob. 16SACh. 9 - Prob. 17SACh. 9 - Prob. 18SACh. 9 - After the following code executes, what elements...Ch. 9 - Prob. 20SACh. 9 - Write a statement that creates a dictionary...Ch. 9 - Write a statement that creates an empty...Ch. 9 - Assume the variable dct references a dictionary....Ch. 9 - Assume the variable dct references a dictionary....Ch. 9 - Prob. 5AWCh. 9 - Prob. 6AWCh. 9 - Prob. 7AWCh. 9 - Prob. 8AWCh. 9 - Prob. 9AWCh. 9 - Prob. 10AWCh. 9 - Assume the variable dct references a dictionary....Ch. 9 - Write code that retrieves and unpickles the...Ch. 9 - Course information Write a program that creates a...Ch. 9 - Capital Quiz The Capital Quiz Problem Write a...Ch. 9 - File Encryption and Decryption Write a program...Ch. 9 - Unique Words Write a program that opens a...Ch. 9 - Prob. 5PECh. 9 - File Analysis Write a program that reads the...Ch. 9 - World Series Winners In this chapters source code...Ch. 9 - Name and Email Addresses Write a program that...Ch. 9 - Blackjack Simulation Previously in this chapter...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).arrow_forwardI cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ? Cisco Packet Tracer 1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardusing r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forward
- using r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forwardusing r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forward
- I want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forwardCisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forward
- Transform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forwardConsidering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
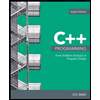
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
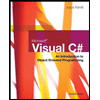
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
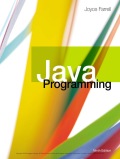
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
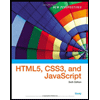
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
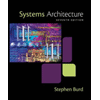
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning