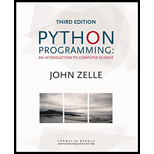
Program plan:
- Import random.
- Define “main()” function.
- Call the function “printIntro()”.
- Get input using “getInputs()” function.
- Call function “simNMatches()” to find the matches.
- Call function “printSummary()”.
- Define a function “printIntro()”.
- Print the statements.
- Define a function “getInputs()”.
- Get the value for simulation parameter “a” from the user.
- Get the value for simulation parameter “b” from the user.
- Get the value for simulation parameter “n” from the user.
- Returns the three simulation parameters.
- Define a function “simNMatches()”.
- Initialize values.
- Iterate a for loop up to “n”.
- Call the function “simOneMatch()”.
- If condition.
- Increment the value of “matchA” by 1.
- Else.
- Increment the value of “matchB” by 1.
- Return the value of “matchA” and “matchB”.
- Define a function “simOneMatch()”.
- Initialize the values “wisnA” and “WinsB” as 0.
- Accumulate number of games.
- Iterate a while loop.
- Call a function “simOneGame()”.
- If condition.
- Increment the value of “winA” by 1.
- Increment the value of “x” by 1.
- Else condition.
- Increment the value of “winsB” by 1.
- Increment the value of “x” by 1.
- Return the value of “winsA” and “winsB”.
- Define a function “simOneGame()”.
- Call a function “findService()” to a value “serving”.
- Initialize values.
- Iterate a while loop.
- If condition “serving == "A"”.
- If condition “random() < probA”.
-
- Increment the value of “scoreA” by 1.
- If not the condition.
-
- Assign a value “B” to a variable “serving”.
- Else if condition.
-
- If condition “random() < probB” increment the value of “scoreB” by 1.
- Increment the value of “scoreB” by 1.
- If not the condition.
-
- assign a value “A” to a variable “serving”
- Return “scoreA”, “scoreB”..
- assign a value “A” to a variable “serving”
- If condition “serving == "A"”.
- Define a function “findService()”.
- If condition “x % 2 == 0”.
- Return “A”.
-
-
- If not the condition.
- Return “B”.
- If not the condition.
-
-
- Return “A”.
- If condition “x % 2 == 0”.
- Define a function “gameOver()”.
- If the condition satisfies.
- Return the value of “b”.
-
-
-
- Else if condition “b == 0 and a == 7”.
- Return the value of “a”.
- Else if condition “abs(a-b) >= 2”.
- Return “True”.
- If not the condition
- Return a value “False”.
- Else if condition “b == 0 and a == 7”.
-
-
-
- Return the value of “b”.
- If the condition satisfies.
- Define a function “printSummary()”.
- Add the value of “matchA” and “matchB” and store in a variable “n”.
- Print the value of “n”.
- Print the value of “matchA”.
- Print the value of “matchB”.
- main for function call

This is a revision of the racquetball simulation. The problem has two major changes:
The program has to calculate results for best of n games.
First service is alternated between A and B. The odd numbered games of the match are served first by A and even numbered games are first served by B.
Explanation of Solution
Program:
#import random
from random import random
#define main() function
def main():
#call the function printIntro()
printIntro()
#get input using getInputs() function
probA, probB, n = getInputs()
#calling function simNMatches() to find the matches
matchA, matchB = simNMatches(n, probA, probB)
#call function printSummary()
printSummary(matchA, matchB)
#define a function printIntro()
def printIntro():
print("This program simulates a game of racquetball between two")
print('players called "A" and "B". The abilities of each player is')
print("indicated by a probability (a number between 0 and 1) that")
print("reflects the likelihood of a player winning the serve.")
print("Player A has the first serve.")
#define a function getInputs()
def getInputs():
#get the value for simulation parameter ‘a’ from the user
a = eval(input("What is the prob. player A wins a serve? "))
#get the value for simulation parameter ‘b’ from the user
b = eval(input("What is the prob. player B wins a serve? "))
#get the value for simulation parameter ‘n’ from the user
n = eval(input("How many games to simulate? "))
#Returns the three simulation parameters
return a, b, n
#define a function simNMatches()
def simNMatches(n, probA, probB):
#initialize values
matchA = matchB = 0
#iterate a for loop up to n
for i in range(n):
#call the function simOneMatch()
winsA, winsB = simOneMatch(probA, probB)
#if condition
if winsA > winsB:
#increment the value of matchA by 1
matchA = matchA + 1
#else
else:
#increment the value of matchB by 1
matchB = matchB + 1
#return the value of matchA and matchB
return matchA, matchB
#define a function simOneMatch()
def simOneMatch(probA, probB):
#initialize the values wisnA and WinsB as 0
winsA = winsB = 0
#accumulate number of games
x = 1
#iterate a while loop
while winsA !=2 and winsB !=2:
#calling a function simOneGame()
scoreA, scoreB = simOneGame(probA, probB, x)
#if condition
if scoreA > scoreB:
#increment the value of winA by 1
winsA = winsA + 1
#increment the value of x by 1
x = x+1
#else condition
else:
#increment the value of winsB by 1
winsB = winsB + 1
#increment the value of x by 1.
x = x+1
#return the value of winsA and winsB
return winsA, winsB
#define a function simOneGame()
def simOneGame(probA, probB, x):
#call a function findService() to a value “serving”
serving = findService(x)
#initialize values
scoreA = 0
scoreB = 0
#iterate a while loop.
while not gameOver(scoreA, scoreB):
#if condition
if serving == "A":
#if condition
if random() < probA:
#increment the value of scoreA by 1
scoreA = scoreA + 1
#if not the condition
else:
#assign a value “B” to a variable “serving”
serving = "B"
#else if condition
elif serving == "B":
#if condition
if random() < probB:
#increment the value of scoreB by 1
scoreB = scoreB + 1
#if not the condition
else:
#assign a value “A” to a variable “serving”
serving = "A"
#return
return scoreA, scoreB
#define a function findService()
def findService(x):
#if condition
if x % 2 == 0:
#return “A”
return "A"
#if not the condition
else:
#return “B”
return "B"
#define a function gameOver()
def gameOver(a, b):
#if the condition satisfies
if a == 0 and b == 7:
#return the value of “b”
return b == 7
#else if condition
elif b == 0 and a == 7:
#return the value of a
return a == 7
#else if condition
elif abs(a-b) >= 2:
#return “True”
return True
#if not the condition
else:
#return a value “False”
return False
#define a function printSummary()
# Prints a summary of wins for each players
def printSummary(matchA, matchB):
#add the value of matchA and matchB and store in a variable n.
n = matchA + matchB
#print the value of n
print("\nGames simulated: ", n)
#print the value of matchA
print("Wins for A: {0} ({1:0.1%})".format(matchA, matchA/n))
#print the value of matchB
print("Wins for B: {0} ({1:0.1%})".format(matchB, matchB/n))
# main for function call
if __name__ == '__main__': main()
Output:
This program simulates a game of racquetball between two
players called "A" and "B". The abilities of each player is
indicated by a probability (a number between 0 and 1) that
reflects the likelihood of a player winning the serve.
Player A has the first serve.
What is the prob. player A wins a serve? 0.5
What is the prob. player B wins a serve? 0.7
How many games to simulate? 2
Games simulated: 2
Wins for A: 1 (50.0%)
Wins for B: 1 (50.0%)
Want to see more full solutions like this?
Chapter 9 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- How do you resize a graphic object horizontally while keeping the center position fixed? Question 20Select one: a. Drag a side sizing handle. b. Press [Ctrl] and drag a side sizing handle. c. Press [Alt] and drag a side sizing handle. d. Press [Shift] and drag a side sizing handle.arrow_forwardWhich of the following indicates that a graphic is anchored to the nearest paragraph? Question 18Select one: a. X and Y coordinates b. An anchor symbol c. A paragraph symbol d. ruler marksarrow_forwardWhich command in the Adjust group allows you to change one picture for another but retain the original picture's size and formatting? Question 17Select one: a. Change Picture b. Replace c. Swap d. Relinkarrow_forward
- How do you insert multiple rows at the same time? Question 10Select one: a. Select the number of rows you want to insert, then use an Insert Control or use the buttons on the Ribbon. b. Click Insert Multiple Rows in the Rows & Columns group. c. Select one row and click the Insert Above or Insert Below button. You will be prompted to choose how many rows to insert. d. You cannot insert multiple rows at the same time.arrow_forwardHow do you center the text vertically in each table cell? Question 9Select one: a. Select the table and click the Distribute Columns button. b. Select the table and click the Center button in the Paragraph group on the Home tab. c. Select the table and click the AutoFit button. d. Click the Select button in the Table group, click Select Table, then click the Align Center Left button in the Alignment group.arrow_forwardA(n) ____ is a box formed by the intersection of a column and a row. Question 8Select one: a. divider b. table c. border d. cellarrow_forward
- A ____ row is the first row of a table that contains the column headings. Question 7Select one: a. header b. primary c. title d. headingarrow_forwardThe Horse table has the following columns: ID - integer, auto increment, primary key RegisteredName - variable-length string Breed - variable-length string Height - decimal number BirthDate - date Delete the following rows: Horse with ID 5 All horses with breed Holsteiner or Paint All horses born before March 13, 2013 To confirm that the deletes are correct, add the SELECT * FROM HORSE; statement.arrow_forwardWhy is Linux popular? What would make someone choose a Linux OS over others? What makes a server? How is a server different from a workstation? What considerations do you have to keep in mind when choosing between physical, hybrid, or virtual server and what are the reasons to choose a virtual installation over the other options?arrow_forward
- Objective you will: 1. Implement a Binary Search Tree (BST) from scratch, including the Big Five (Rule of Five) 2. Implement the TreeSort algorithm using a in-order traversal to store sorted elements in a vector. 3. Compare the performance of TreeSort with C++'s std::sort on large datasets. Part 1: Understanding TreeSort How TreeSort Works TreeSort is a comparison-based sorting algorithm that leverages a Binary Search Tree (BST): 1. Insert all elements into a BST (logically sorting them). 2. Traverse the BST in-order to extract elements in sorted order. 3. Store the sorted elements in a vector. Time Complexity Operation Average Case Worst Case (Unbalanced Tree)Insertion 0(1log n) 0 (n)Traversal (Pre-order) 0(n) 0 (n)Overall Complexity 0(n log n) 0(n^2) (degenerated tree) Note: To improve performance, you could use a…arrow_forwardI need help fixing the minor issue where the text isn't in the proper place, and to ensure that the frequency cutoff is at the right place. My code: % Define frequency range for the plot f = logspace(1, 5, 500); % Frequency range from 10 Hz to 100 kHz w = 2 * pi * f; % Angular frequency % Parameters for the filters - let's adjust these to get more reasonable cutoffs R = 1e3; % Resistance in ohms (1 kΩ) C = 1e-6; % Capacitance in farads (1 μF) % For bandpass, we need appropriate L value for desired cutoffs L = 0.1; % Inductance in henries - adjusted for better bandpass response % Calculate cutoff frequencies first to verify they're in desired range f_cutoff_RC = 1 / (2 * pi * R * C); f_resonance = 1 / (2 * pi * sqrt(L * C)); Q_factor = (1/R) * sqrt(L/C); f_lower_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) + 1/(2*Q_factor)); f_upper_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) - 1/(2*Q_factor)); % Transfer functions % Low-pass filter (RC) H_low = 1 ./ (1 + 1i * w *…arrow_forwardMy code is experincing minor issue where the text isn't in the proper place, and to ensure that the frequency cutoff is at the right place. My code: % Define frequency range for the plot f = logspace(1, 5, 500); % Frequency range from 10 Hz to 100 kHz w = 2 * pi * f; % Angular frequency % Parameters for the filters - let's adjust these to get more reasonable cutoffs R = 1e3; % Resistance in ohms (1 kΩ) C = 1e-6; % Capacitance in farads (1 μF) % For bandpass, we need appropriate L value for desired cutoffs L = 0.1; % Inductance in henries - adjusted for better bandpass response % Calculate cutoff frequencies first to verify they're in desired range f_cutoff_RC = 1 / (2 * pi * R * C); f_resonance = 1 / (2 * pi * sqrt(L * C)); Q_factor = (1/R) * sqrt(L/C); f_lower_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) + 1/(2*Q_factor)); f_upper_cutoff = f_resonance / (sqrt(1 + 1/(4*Q_factor^2)) - 1/(2*Q_factor)); % Transfer functions % Low-pass filter (RC) H_low = 1 ./ (1 + 1i * w *…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
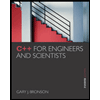
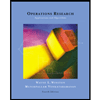
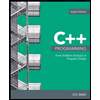
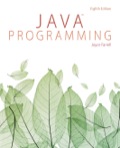