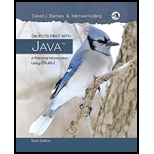
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
6th Edition
ISBN: 9780134477367
Author: David J. Barnes, Michael Kolling
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 9, Problem 1E
Program Plan Intro
To test that comments can be added, and to inspect the data element of the ArrayList object.
Expert Solution & Answer

Explanation of Solution
Explanation:
Java allows using the JUnit test framework to write and execute automated tests. It contains a set of assert methods to test method code. It uses @Test annotation to define the test case. It also includes two methods:
- setUp() method that runs before every test invocation.
- tearDown() method that runs after every test method.
Program:
The required Java code for JUnit test case is given below:
import static org.junit.Assert.*; import org.junit.After; import org.junit.Before; import org.junit.Test; // The test class SalesItemTest. public class SalesItemTest { // Default constructor public SalesItemTest() { } // Sets up the test fixture. @Before public void setUp() { } // Tears down the test fixture. @After public void tearDown() { } /& * Test case to add two comments and that check the size of ArrayList is correct afterwards. */ @Test public void testAddComments() { SalesItem salesItem1 = new SalesItem("Java for complete Idiots", 21998); assertEquals(true, salesItem1.addComment("James Duckling", "This book is great.", 4)); assertEquals(true, salesItem1.addComment("Jhoky Jonathan", "Super Java book.", 5)); assertEquals(2, salesItem1.getNumberOfComments()); } }
In the above JUnit test case, the two comments are added then test the size of ArrayList by comparing the return value of getNumberOfComments() method with 2.
The inspector to check for the comment list, their size, and elementData field of ArrayList object is as follows:
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
Students have asked these similar questions
Write out the header of a for-each loop to process an ArrayList called tracks. Don’t worry about the loop’s body.
Update it to create a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it into an ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed):
d) Use lambdas and streams to calculate the total average of all Students grades, then show the result.
e) Use lambdas and streams to group Students by major, then show the results.
1.
Ch3HW;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class MainApp extends Application{
@Override
publicvoidstart(StageprimaryStage) throwsException {
FXMLLoader loader…
Update it to create a class Student that includes four properties: an id (type Integer), a name (type String), a Major (type String) and a Grade (type Double). Use class Student to create objects (using buttonAdd) that will be read from the TextFields then save it into an ArrayList. Perform the following queries on the ArrayList of Student objects and show the results on the listViewStudents (Hint: add buttons as needed):
d) Use lambdas and streams to calculate the total average of all Students grades, then show the result.
1.
Ch3HW;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class MainApp extends Application{
@Override
publicvoidstart(StageprimaryStage) throwsException {
FXMLLoader loader =newFXMLLoader(getClass().getResource("StudentScreen.fxml"));
Parent parent = loader.load();
Scene scene =newScene(parent);…
Chapter 9 Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Knowledge Booster
Similar questions
- How do you clear the whole canvas?arrow_forwardPlease help me create a cave class for a Hunt the Wumpus game. You can read the rules in it's entirety of the Hunt the Wumpus game online to get a better idea of the specifications. It's an actual game. INFORMATION: The object of this game is to find and kill the Wumpus within a minimum number of moves, before you get exhausted or run out of arrows. There is only one way to win: you must discover which room the Wumpus is hiding in and kill it by shooting an arrow into that room from an adjacent room. The Cave The Wumpus lives in a cave of 30 rooms. The rooms are hexagonal. Each room has up to 3 tunnels, allowing access to 1, 2 or 3 (out of 6) adjacent rooms. The attached diagram shows the layout of rooms in the cave. The map wraps around such that rooms on the edges (white cells) have neighbors from the opposite edge (blue cells). E.g., the neighbors of room 1 are rooms 25, 26, 2, 7, 6, and 30, and you could choose to connect room 1 to any of these rooms. Observe how room 1…arrow_forward3. Create an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to avoid method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time. See Sample Output. SAMPLE OUTPUT Here is the list Lionel Messi Drake Adele Dwayne Johnson Beyonce Here is the new list * Lionel Messi * Drake * Taylor Swift * Adele * Beyonce Using an iterator, here is the list Lionel Messi Drake Taylor Swift Adele Beyoncearrow_forward
- Write out what you think the outer wrappers of the Student and LabClass classes might look like; do not worry about the inner part.arrow_forwardImplement the "paint fill"feature seen in several picture editing products.To put it another way, if you have a screen (represented by a two-dimensional array of colours), a point, and a new colour, Fill in the surrounding area until the colour shifts away from the original.arrow_forwardCreate some Student objects. Call the getName method on each object. Explain what is happening.arrow_forward
- List all of the external method calls that are made in the draw method of Picture on the Triangle object called roof.arrow_forwardIs there a penalty for adding a new key-value combination to an existing map entry?arrow_forwardIf you apply the Visitor design pattern in your design, it becomes easy to add new ConcreteElement classes to the Element hierarchy. Is this true or false?arrow_forward
- Provide a code segment that creates an arraylist of three integers and prints these values on the screen. Then add two more integers and print out the last integer on the screen. Note: You are free to choose integers.arrow_forwardCreate an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to a void method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time.arrow_forwardThe UnsortedTableMap class is located in the maps folder. In the attachment, you will find a partially implemented class called UnsortedMapOfLists, which extends UnsortedTableMap. The values associated with the keys in UnsortedMapOfLists are arrays. So you have an array with each key. Off course you can save an array in the value of UnsortedTableMap class without the class we are going to develop, but our class makes this easier, as it allows using a syntax like this: myMap["somekey", 1] to reach element at index 1 in the array associated with key "somekey". I already implemented methods __setitem__ and __getitem__. Your task is to: implement method __delitem__ . Deletion will be performed for an element in the array. If index is not given, delete the array associated with the key. implement a function (not a method in the class) to swap the arrays associated with two given keys. Add the required comment in method __getitem__. implement a main to verify that your code is working…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
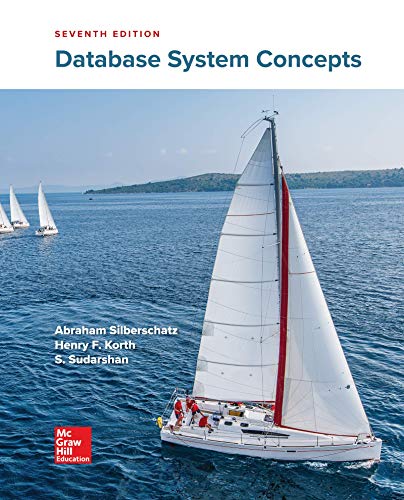
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
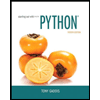
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
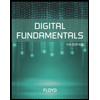
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
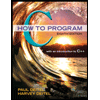
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
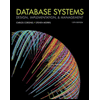
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
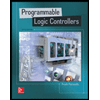
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education