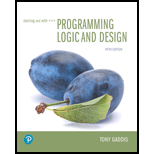
Assume the following main module is in a
// This program uses the binarySearch function to search for a
// name in the array. This program assumes the binarySearch
// function has already been defined.
Module main ()
Constant Integer SIZE = 5
Declare String names [SIZE] = "Zack", "James", "Pam",
"Marc" , "Susan"
Declare String searchName
Declare Integer index
Display "Enter a name to search for."
Input searchName
Set index = binarySearch(names, searchName , SIZE)
If index != - 1 Then
Display searchName, "was found."
Else
Display searchName, "was NOT found."
End If
End Module

Learn your wayIncludes step-by-step video

Chapter 9 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Programming in C
Java How To Program (Early Objects)
Problem Solving with C++ (10th Edition)
C Programming Language
Starting Out with Python (4th Edition)
C++ How to Program (10th Edition)
- Find the C program language Breaking the Code by CodeChum Admin ATTENTION SOLDIER! This will be the hardest mission of your life. Our intelligence department has caught the enemy sending codes from one base to another. These enemy codes can either be a simple communication message or a mastermind plan. Our intelligence department has determined that a mastermind plan is a code that has at least 1 consonant character. Since you know programming, your task is to check a code if it is a mastermind plan or not.arrow_forwardC Program Functions with 1D Arrays Create a program that asks the user for the size of an integer array and the elements of the array. Then, create a function with the following details: Parameters: An integer array The size of the integer array Return type - int Return value - the maximum value of the integer array Call the function you created in the main and pass the integer array and its size to it. Make sure to store the return value in a variable so you could print it afterwards. Input 1. First line contains the size of the array 2. Succeeding lines are the elements of an array Output Enter size of array: 5 Enter element 1: 1 Enter element 2: 2 Enter element 3: 3 Enter element 4: 4 Enter element 5: 5 Maximum element: 5arrow_forwardInstructions Write a program in C++ that uses a two-dimensional array to store the highest and lowest temperatures for each month of the year. The program should output the average high, average low, and the highest and lowest temperatures for the year. Your program must consist of the following functions: Function getData: This function reads and stores data in the two-dimensional array. Function averageHigh: This function calculates and returns the average high temperature for the year. Function averageLow: This function calculates and returns the average low temperature for the year. Function indexHighTemp: This function returns the index of the highest high temperature in the array. Function indexLowTemp: This function returns the index of the lowest low temperature in the array. These functions must all have the appropriate parameters. An example of the program is shown below: Enter high temperature for each month 60 68 72 72 73 76 80 81 75 72 67 66 Enter low temperature for…arrow_forward
- Java - Functions with 1D Arrays Create a program that asks the user for the size of an integer array and the elements of the array. Then, create a function called maxArray() with the following details: Parameters: An integer array The size of the integer array Return type - int Return value - the maximum value of the integer array Call the function you created in the main and pass the integer array and its size to it. Make sure to store the return value in a variable so you could print it afterwards. Input 1. First line contains the size of the array 2. Succeeding lines are the elements of an array 3. First line contains the size of the array 4. Succeeding lines are the elements of an array 5. First line contains the size of the array 6. Succeeding lines are the elements of an array 7. First line contains the size of the array 8. Succeeding lines are the elements of an array Output Enter size of array: 5 Enter element 1: 1 Enter element 2: 2 Enter…arrow_forwardPrograming language is Carrow_forwardMovie Data Write a program that will be used to gather statistical data about the number of movies college students see in a month. The program should ask the user how many students were surveyed and dynamically allocate an array of that size. The program then should allow the user to enter the number of movies each student has seen. It should then sort the scores and calculate the average. Modularity: Main: The main function should accept the number of students from the user and dynamically create an array large enough to contain number of movies watched for each student. Input validation: The number of students should be a positive integer. Print the average and free the allocated array when complete. Get the data: This function should get the number of movies watched by each college student. Input validation: The number of movies should be a positive integer. Sort the data: This function should sort the array in ascending order. Note you may use the Standard Template Library sort…arrow_forward
- Reverse ArrayWrite a function that accepts an int array and the array’s size as arguments. The function should create a copy of the array, except that the element values should be reversedin the copy. The function should return a pointer to the new array. Demonstrate thefunction in a complete program.arrow_forwardTo receive an array argument, a function's parameter list must specify that the function expects to receive an array. The size of the array is not required between the array brackets. Select one: True Falsearrow_forwardIn C Programming: Write a function inputAllCourses() which receives an array of course pointers and the array’s size, then allows the user to input all courses in the array by calling inputCourse()arrow_forward
- For C++: Design an application that has an array of at least 20 integers. It should call a module that uses the sequential search algorithm to locate one of the values. The module should keep a count of the number of comparisons it makes until it finds the value. Then the program should call another module that uses the binary search algorithm to locate the same value. It should also keep a count of the number of comparisons it makes. Display these values on the screen.arrow_forwardDuplicate Count Code in C Languagearrow_forwardIn C++ Create a function called CopyAndInsertArray. It should take in two arrays (the first array should be of size n and the second array should be of size n+1). The function should also take in a position and a number. It should copy the first array into the second array and place the new number at the proper position. In other words, you are creating a function that inserts a value into an array.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
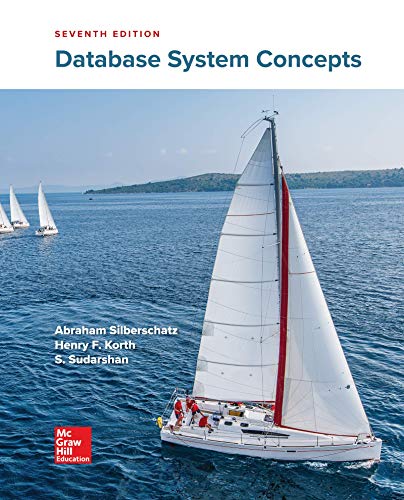
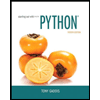
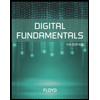
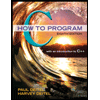
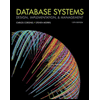
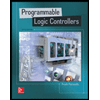