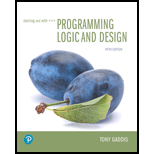
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 9.3, Problem 9.2CP
Program Plan Intro
Sorting:
When contents of the array being arranged in the particular order is called as sorting. The order of arranging the contents can be ascending or descending order.
Types of sorting:
- Bubble sort
- Selection sort
- Insertion sort
- Merge sort
- Quick sort
- Shell sort
- Heap sort
These sort have the possibility of sorting the array in both ascending and descending order.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even.
By using java, Implement the Double Insertion sort algorithm on a randomly generated list of N integer numbers. Your…
Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even.
Implement the Double Insertion sort algorithm by using JAVA on a randomly generated list of N integer Your program…
Double Insertion Sort is a variation on Insertion Sort that works from the middle of the array out. At each iteration, some middle portion of the array is sorted. On the next iteration, take the two adjacent elements to the sorted portion of the array. If they are out of order with respect to each other, then swap them. Now, push the left element toward the right in the array so long as it is greater than the element to its right. And push the right element toward the left in the array so long as it is less than the element to its left. The algorithm begins by processing the middle two elements of the array if the array is even. If the array is odd, then skip processing the middle item and begin with processing the elements to its immediate left and right. Implement Double Insertion Sort, being careful to properly handle both when the array is odd and when it is even.
Improved Bubble Sort: One possible improvement for Bubble Sort would be to add a flag variable and a test that…
Chapter 9 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 9.3 - Which of the sorting algorithms discussed makes...Ch. 9.3 - Prob. 9.2CPCh. 9.3 - Prob. 9.3CPCh. 9.4 - Prob. 9.4CPCh. 9.4 - On average, with an array of 1,000 elements, how...Ch. 9.4 - Prob. 9.6CPCh. 9 - Prob. 1MCCh. 9 - Prob. 2MCCh. 9 - Prob. 3MCCh. 9 - Prob. 4MC
Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - Prob. 1TFCh. 9 - Prob. 2TFCh. 9 - Prob. 3TFCh. 9 - Prob. 4TFCh. 9 - Prob. 5TFCh. 9 - Prob. 1AWCh. 9 - Prob. 2AWCh. 9 - Prob. 3AWCh. 9 - What algorithm does the following pseudocode...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - Prob. 4SACh. 9 - Prob. 5SACh. 9 - Why is the selection sort more efficient than the...Ch. 9 - Prob. 7SACh. 9 - Prob. 8SACh. 9 - Assume the following main module is in a program...Ch. 9 - Prob. 1PECh. 9 - Sorted Names Design a program that allows the user...Ch. 9 - Rainfall Program Modification Recall that...Ch. 9 - Name Search Modify the Sorted Names program that...Ch. 9 - Charge Account Validation Recall that Programming...Ch. 9 - Prob. 7PECh. 9 - Sorting Benchmarks Modify the modules presented in...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A sort algorithm that finds the smallest element of the array and interchanges it with the element in the first position of the array. Then it finds the second smallest element from the remaining elements in the array and places it in the second position of the array and so onarrow_forwardThis is possible because the algorithm assumes that each of the n input elements is in the range [0, k], i.e. no element is smaller than 0 nor greater than k. The input is an array A indexed from 1 to n, the output is stored in the array B also indexed from 1 ton, and the array C is used as a temporary container, indexed from 0 to k. 1 2 3 5 6 7 8 10 11 12 let C[0..k] be a new array for i=0 to k C[i] = 0 for j = 1 to A.length C[A[j]] = C[A[j]] + 1 // C[i] now contains the number of elements equal to i. for i=1 to k C[i] = C[i] + C[i-1] // C[i] now contains the number of elements less than or equal to i. for j = A.length downto 1 B[C[A[j]]]= A[j] C[A[j]] = C[A[j]] - 1 Given an input array A = [5, 1, 2, 1, 5, 3], what is the status of C' at the end of the algorithm? ○ 1, 2, 3, 4, 4,6 none of the others O 0, 1, 2, 3, 5,5 O 4, 4, 5, 2,0 O 0, 0, 2, 3, 4, 4arrow_forwardWrite a program that reads the numbers and sorts them by using the Counting Sort algorithm and finally search a number from that array using Linear Search Algorithm. Input: 3 6 5 4 789 Search Item: 7 Output: Sorted Array: 3 4 5 6 789 Search item 7 is found.arrow_forward
- Mina always wants things to be sorted. She loves perfection in work and doesn't worry about time. Her brother gifted her some boxes with different sizes. Now she needs your help to sort it. You're given n boxes with different sizes and your task is to sort the boxes in ascending order of size. You should recursively partition the array with a number p so that all the elements greater than p are to its right and all the elements lower than p to its left to sort the boxes. Make sure you are giving a solution that doesn't exceed the time complexity of O(n^2), but on average has complexity of O(nlogn) a. Which algorithm will you suggest to Mina? Explain if your algorithm is stable or unstable with appropriate example b. Show the step by step simulation of your algorithm on the boxes of size 5, 9, 10, 3, 15, 12, 6, 20, 9, 13, 3, 10, 1, 2. c. Give an example of box sizes when it will be worst case and when it will be the best case for this algorithm.arrow_forward1. Write a program that compares all four advanced sorting algorithms . To perform the tests, create a randomly generated array of 1,000 elements. What is the ranking of the algorithms? What happens when you increase the array size to 10,000 elements and then 100,000 elements?arrow_forwardThe binary search algorithm that follows may be used to search an array when the elements are in order. This algorithm is analogous to the following approach to finding a name in a telephone book. a. Open the book in the middle and look at the middle name on the page. b. b. If the middle name isn't the one, you're looking for, decide whether it comes before or after the name you want. c. Take the appropriate half of the section of the book you were looking in and repeat these steps until you land on the name. 1. Let the bottom be the subscript of the initial array element. 2. Let the top be the subscript of the last array element. 3. Let found be false. 4. Repeat as long as the bottom isn't greater than the top and the target has not been found. 5. Let middle be the subscript of the element halfway between bottom and top. 6. If the element in the middle is the target 7. Set found to true and index to middle. else if the element in the middle is larger than the target 8. Let the top be…arrow_forward
- the qusetion on the picturearrow_forwardSort the array (D, G, J, F, A, C) using selection sort (show the array after each step).arrow_forwardAn input array consists of multiple integers. Write a sorting program to sort the content of thearray in place.Sample:Input: {4,2,0,3,4,0,4,1,2,1,3}Output: [0 0 1 1 2 2 3 3 4 4 4]arrow_forward
- Javaarrow_forwardMultiply left and right array sum. Basic Accuracy: 54.29% Submissions: 12630 Points: 1 Pitsy needs help in the given task by her teacher. The task is to divide a array into two sub array (left and right) containing n/2 elements each and do the sum of the subarrays and then multiply both the subarrays. Example 1: â€arrow_forward23 37 56 74 80 90 112 114 123 129 208 249 266 270 271 Suppose you use binary search to search for the value 129 in the array above. Fill in the values for each iteration of the search. The first one is filled in as an example. Remember high and low store the indices that track which part of the array is being considered. Iteration 1: high: 14 low: 0 mid: 7 Iteration 2: high: low: mid: Iteration 3: high: low: mid:arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
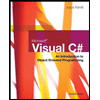
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage