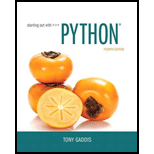
Starting Out with Python (4th Edition)
4th Edition
ISBN: 9780134444321
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8.1, Problem 4CP
Program Plan Intro
Accessing characters in string:
- • In Python, there are two techniques for accessing characters present in string.
- ○ Using “for” loop.
- ■ Each time the loop operates, variable will refer a copy of character in the string that begins with the first character.
- ○ Using indexing.
- ■ The characters can be accessed using index.
- ■ Each character of a string has an index that specifies the string’s position.
- ■ Indexing starts at 0 and ends with a count of one less than the total number of characters in a string.
- ○ Using “for” loop.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Considering the TM example of binary sum ( see attached)do the step-by-step of execution for the binary numbers 1101 and 11.
Feel free to use the Formal Language Editor Tool to execute it;
Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step.
I need help on inculding additonal code where I can can do the opposite code of MatLab, where the function of t that I enter becomes the result of F(t), in other words, turning the time-domain f(t) into the frequency-domain function F(s):
I need help with the TM computation step-by-step execution for the binary numbers 1101 and 11.
Formal Language Editor Tool can be used to execute it; Write it down the current state of the tape (including the head position) and indicate the current state of the TM at each step;
Chapter 8 Solutions
Starting Out with Python (4th Edition)
Ch. 8.1 - Assume the variable name references a string....Ch. 8.1 - What is the index of the first character in a...Ch. 8.1 - If a string has 10 characters, what is the index...Ch. 8.1 - Prob. 4CPCh. 8.1 - Prob. 5CPCh. 8.1 - Prob. 6CPCh. 8.2 - Prob. 7CPCh. 8.2 - Prob. 8CPCh. 8.2 - Prob. 9CPCh. 8.2 - What will the following code display? mystring =...
Ch. 8.3 - Prob. 11CPCh. 8.3 - Prob. 12CPCh. 8.3 - Write an if statement that displays Digit" if the...Ch. 8.3 - What is the output of the following code? ch = 'a'...Ch. 8.3 - Write a loop that asks the user Do you want to...Ch. 8.3 - Prob. 16CPCh. 8.3 - Write a loop that counts the number of uppercase...Ch. 8.3 - Assume the following statement appears in a...Ch. 8.3 - Assume the following statement appears in a...Ch. 8 - This is the first index in a string. a. 1 b. 1 c....Ch. 8 - This is the last index in a string. a. 1 b. 99 c....Ch. 8 - This will happen if you try to use an index that...Ch. 8 - This function returns the length of a string. a....Ch. 8 - This string method returns a copy of the string...Ch. 8 - This string method returns the lowest index in the...Ch. 8 - This operator determines whether one string is...Ch. 8 - This string method returns true if a string...Ch. 8 - This string method returns true if a string...Ch. 8 - This string method returns a copy of the string...Ch. 8 - Once a string is created, it cannot be changed.Ch. 8 - You can use the for loop to iterate over the...Ch. 8 - The isupper method converts a string to all...Ch. 8 - The repetition operator () works with strings as...Ch. 8 - Prob. 5TFCh. 8 - What does the following code display? mystr =...Ch. 8 - What does the following code display? mystr =...Ch. 8 - What will the following code display? mystring =...Ch. 8 - Prob. 4SACh. 8 - What does the following code display? name = 'joe'...Ch. 8 - Assume choice references a string. The following...Ch. 8 - Write a loop that counts the number of space...Ch. 8 - Write a loop that counts the number of digits that...Ch. 8 - Write a loop that counts the number of lowercase...Ch. 8 - Write a function that accepts a string as an...Ch. 8 - Prob. 6AWCh. 8 - Write a function that accepts a string as an...Ch. 8 - Assume mystrinc references a string. Write a...Ch. 8 - Assume mystring references a string. Write a...Ch. 8 - Look at the following statement: mystring =...Ch. 8 - Initials Write a program that gets a string...Ch. 8 - Sum of Digits in a String Write a program that...Ch. 8 - Date Printer Write a program that reads a string...Ch. 8 - Prob. 4PECh. 8 - Alphabetic Telephone Number Translator Many...Ch. 8 - Average Number of Words If you have downloaded the...Ch. 8 - If you have downloaded the source code you will...Ch. 8 - Sentence Capitalizer Write a program with a...Ch. 8 - Prob. 10PECh. 8 - Prob. 11PECh. 8 - Word Separator Write a program that accepts as...Ch. 8 - Pig Latin Write a program that accepts a sentence...Ch. 8 - PowerBall Lottery To play the PowerBall lottery,...Ch. 8 - Gas Prices In the student sample program files for...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Ensure you answer the question asked at the end of the document. Do not just paste things without the GNS3 console outputsarrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSolve this "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward
- "Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forward"Do not use AI tools. Solve the problem by hand on paper only and upload a photo of your handwritten solution."arrow_forwardSpecifications: Part-1Part-1: DescriptionIn this part of the lab you will build a single operation ALU. This ALU will implement a bitwise left rotation. Forthis lab assignment you are not allowed to use Digital's Arithmetic components.IF YOU ARE FOUND USING THEM, YOU WILL RECEIVE A ZERO FOR LAB2!The ALU you will be implementing consists of two 4-bit inputs (named inA and inB) and one 4-bit output (named out). Your ALU must rotate the bits in inA by the amount given by inB (i.e. 0-15).Part-1: User InterfaceYou are provided an interface file lab2_part1.dig; start Part-1 from this file.NOTE: You are not permitted to edit the content inside the dotted lines rectangle. Part-1: ExampleIn the figure above, the input values that we have selected to test are inA = {inA_3, inA_2, inA_1, inA_0} = {0, 1, 0,0} and inB = {inB_3, inB_2, inB_1, inB_0} = {0, 0, 1, 0}. Therefore, we must rotate the bus 0100 bitwise left by00102, or 2 in base 10, to get {0, 0, 0, 1}. Please note that a rotation left is…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
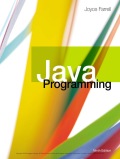
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
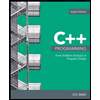
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
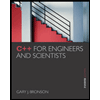
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
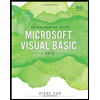
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
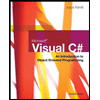
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,