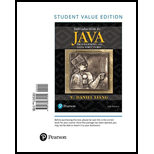
Concept explainers
(Sum elements column by column) Write a method that returns the sum of all the elements in a specified column in a matrix using the following header:
public static double sumColumn(double[][] m, int columnIndex)
Write a test program that reads a 3-by-4 matrix and displays the sum of each column. Here is a sample run:

Sum of elements column by column
Program Plan:
- Include the “Scanner” package to get input values from user.
- Define the class named “Test”.
- Define the main method.
- Define the object “obj” for “Scanner” class to get input.
- Declare the multi-dimensional array named “array”, and prompt the user to get array input.
- Define the “for” loops, that get the input values for the variable “array”.
- Define the “for” loop, that call the method “sumColumn()” with two arguments, they are array and index of array.
- Define the method named “sumColumn()” with two arguments. One is a array variable “m” in type of “double” and another one is “columnIndex” with integer data type.
- Declare the variable “total” in type of “double” and initialize the variable with “0”.
- Set the “for” loop, the loop executes from “0” to length of array “m”.
- Add the column values and store it into the “total”.
- Return the value of “total”.
- Define the main method.
The following JAVA code is to sum the elements of array column by column using the method “sumColumn(double[][] m, int columnIndex)”.
Explanation of Solution
Program:
//Insert package
import java.util.Scanner;
//Class definition
public class Test
{
//Main method
public static void main(String[] args)
{
//Assign the object for "Scanner" class
Scanner obj = new Scanner(System.in);
//Print statement
System.out.print("Enter a 3 by 4 matrix row by row: ");
//Declaration of variable
double[][] array = new double[3][4];
//Outer loop
for (int i = 0; i < array.length; i++)
//Inner Loop
for (int j = 0; j <array[i].length; j++)
//Get input from user
array[i][j] = obj.nextDouble();
//Loop
for (int j = 0; j < array[0].length; j++)
{
//Print statement with function call
System.out.println("Sum of the elements at column " + j + " is " + sumColumn(array, j));
}
}
//Function definition
public static double sumColumn(double[][] m, int columnIndex)
{
//Declaration of variable
double total = 0;
//Loop
for (int i = 0; i < m.length; i++)
//Add the array values into variable
total += m[i][columnIndex];
//Return statement
return total;
}
}
Enter a 3 by 4 matrix row by row:
1.5 2 3 4
5.5 6 7 8
9.5 1 3 1
Sum of the elements at column 0 is 16.5
Sum of the elements at column 1 is 9.0
Sum of the elements at column 2 is 13.0
Sum of the elements at column 3 is 13.0
Want to see more full solutions like this?
Chapter 8 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version, Student Value Edition (11th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Concepts Of Programming Languages
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
- 3-Write a test program that reads a n-by-m matrix Reads a real numbers and computes the sum and average.arrow_forwardWrite a function that displays an n-by-n matrix using the following header: void printMatrix(int n)Each element is 0 or 1, which is generated randomly. Write a test program that prompts the user to enter n and displays an n-by-n matrix.arrow_forwardWrite a function that returns the sum of all the elements in a specified column in a matrix using the following header:def sumColumn(m, columnIndex): Write a test program that reads a 3x4 matrix and displays the sum of each column.arrow_forward
- i. Creates a matrix using: B=rand (20) a-write a program to find all numbers less than or equal 0.5 and put them in a new matrix with dimension 20x10. you can extend with zeros to fill the matrix. b- Do same as point a for all numbers above 0.5. c- Count all the numbers less or equal 0.3 , the numbers between 0.3 and 0.7 and count the numbers more than or equal 0.7.Put the results in a new vector with dimension 3x1. d- Replace the numbers of between 0.7 and 0.9 with 1. e- Add all the elements column wise.arrow_forward2-Write a test program that reads a 4-by-4 matrix and displays the multiply of each rowarrow_forwardWrite method body of following methods: DeleteNum(int position) // This method will delete element from array at position entered by the user arrange the data after that position to left. (Using While loop) DeleteNum(int from, int to) // This method will delete elements from array at range given by the user, as the user inputs two positions i.e start and end, the program should than delete all numbers in that range. (Use C++ to code)arrow_forward
- An array index should normally be of type float. State whether the statement is true or false. If false, explain why.arrow_forward(FYI: Pseudocode is required (Not any programming language) Design a pseudocode program that loads an array with the following 7 values. Add one more word (of your own choosing) for a total of 8 words. biffcomelyfezmottleperukebedraggledquisling Be sure to use lowercase, as shown above. This will make the processing easier. Ask the user to enter a word Search through this array until you find a match with the word the user entered. Once you find a match, output "Yes, that word is in the dictionary". If you get to the end of the array and do NOT find a match, output "No, that word is not in the dictionary". The program should work with any set of words in the arrays. If I were to change the words in the arrays, it should still work. If you need help, look at the search example in your textbook.arrow_forwardAlert dont submit AI generated answer.arrow_forward
- Java Objective:Design and implement simple matrix manipulation techniques.Details:Your java program should use 2D arrays to implement simple matrix operations.Your program should do the following:• Read the number of rows and columns of a matrix M1 from the user. Use an input validation loop to make sure the values are greater than 0. • Read the elements of M1 in row major order• Print M1 to the console; make sure you format as a matirx• Repeat the previous steps for a second matrix M2• Create a matrix M3 that is the transpose of M1 and print it to the console• Check if M1 and M2 can be added (should have the same dimensions). If possible, add M1 and M2 and print the result to the console. Otherwise print an error message.• Multiply M1 and M2 if possible and print to the console. If the matrices cannot be multiplied, print an error message. Implementation requirements:• Use a helper method for reading a positive integer using an input validation loop.• Use a helper method for printing…arrow_forwardwhat is correct answer?arrow_forwardQ2: In this question, you will write a Python function to compute the Hadamard product of two matrices. The Hadamard product is the "component-wise" product of two matrices. That is, each entry of the product matrix is equal to the product of its corresponding entries of the input matrices. Here is an example: a11 a12 a21 a22 a23 a31 a13 SH a32 033 A b11 b12 b13 b21 b22 b23 b31 b32 b33. B = [an bu a21 b21 a31 b31 a12 b12 a22 b22 a32 b32 a13 b13 a23 b23 a33 b33. Hadamard product of A and B Write a Python function to compute the Hadamard product of two matrices as follows. a) The inputs to the function are two matrices. b) The function should check whether the two matrices have the same size. If not, it should print "The input matrices are not of the same size". c) If two matrices are of the same size, the function computes the Hadamard product and returns the answer. Use for loops to compute the Hadamard product.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
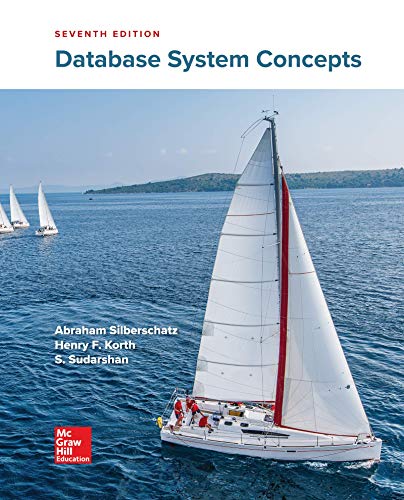
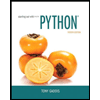
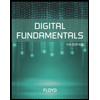
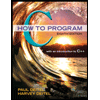
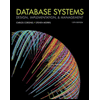
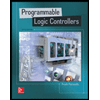