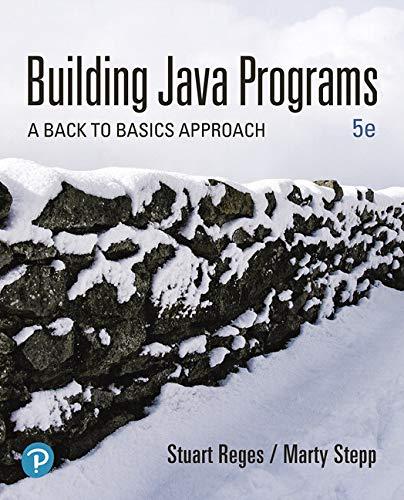
Explanation of Solution
“quadrant” method:
//definition of "Point" class
public class Point
{
//declare the required variables
int x;
int y;
//definition of "quadrant" method
public int quadrant()
{
//check "x" and "y" is greater than 0
if (x > 0 && y > 0)
{
//return "1"
return 1;
}
/*check "x" is less than 0 and "y" is greater than 0*/
else if (x < 0 && y > 0)
{
//return "2"
return 2;
}
//check "x" and "y" is less than 0
else if (x < 0 && y < 0)
{
//return "3"
return 3;
}
/*check "x" is greater than 0 and "y" is less than 0*/
else if (x > 0 && y < 0)
{
//return "4"
return 4;
}
//otherwise
else
{
//return "0"
return 0;
}
}
}
Explanation:
- In the above program, the “Point” is the class name,
- Inside the “Point” class, declare the “x” and “y” variables and the accessor method, the “quadrant” is the method name
- If the “x” and “y” are greater than 0, then return 1 to the called method.
- If the “x” is less than 0 and “y” is greater than 0, then return 2 to the called method.
- If the “x” and “y” are less than 0, then return 3 to the called method.
- If the “x” is greater than 0 and “y” is less than 0, then return 4 to the called method.
Want to see more full solutions like this?
Chapter 8 Solutions
Building Java Programs: A Back To Basics Approach (5th Edition)
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
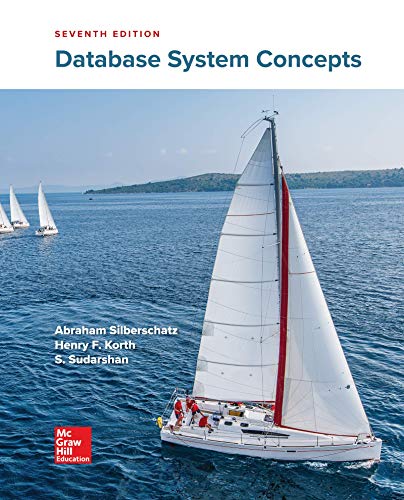
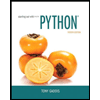
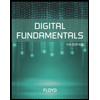
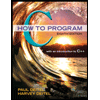
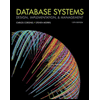
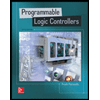