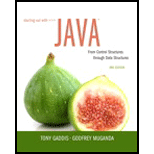
Assuming the following enum declaration exists:
enum Dog { POODLE, BOXER, TERRIER }
what will the following statements display?
- a. System.out.println(Dog.POODLE + “\n” +
Dog.BOXER + ”\n” +
Dog.TERRIER);
- b. System.out.println(Dog.POODLE.ordinal() + ”\n” +
Dog.BOXER.ordinal() + ”\n” +
Dog.TERRIER.ordinal ());
- c. Dog myDog = Dog.BOXER;
if (myDog.compareTo(Dog.TERRIER) > 0)
System.out.println(myDog + “ is greater than ” +
Dog.TERRIER);
else
System.out.println(myDog + “ is NOT greater than ” +
Dog.TERRIER);

Want to see the full answer?
Check out a sample textbook solution
Chapter 8 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Additional Engineering Textbook Solutions
Objects First with Java: A Practical Introduction Using BlueJ (6th Edition)
Software Engineering (10th Edition)
Starting Out With Visual Basic (8th Edition)
Problem Solving with C++ (9th Edition)
C Programming Language
Starting Out with Python (4th Edition)
- Consider the following data type definitions struct A{ char c; int i;}; union U{ struct A a1; struct A a2;}; And the following statements. You can assume that rest of the code is valid. union U u1;u1.a1.c = 'A';u1.a2.c = 'B'; u1.a2.i = 50; What would happen if you do a printf("%c", u1.a1.c); ? Choose ONLY the correct answers (one or more). Question 9 options: It will print B The behaviour is undefined, it might print either A or B It will print A It will print the character whose ASCII equivalent is 50 It will print ABarrow_forwardusing System; using System.Linq; using System.Text; namespace Games; { class Program { staticvoid Main(string[] args) { int choice,games_choice; cout<<"What are your favorite games - outdoor or indoor"<<endl; cout<<"Press 1 for 'outdoor' or Press 2 for 'indoor'"<<endl; cin>>choice; if(choice==1) { cout<<"What is your favorite outdoor game"<<endl; cout<<"Press 1 for Tennis"<<endl; cout<<"Press 2 for Football"<<endl; cout<<"Press 3 for Cricket"<<endl; cin>>games_choice; if(games_choice==1) cout<<"I also like Tennis"<<endl; elseif(games_choice==2) cout<<"I also like Football"<<endl; elseif(games_choice==3) cout<<"I also like Cricket"<<endl; } elseif(choice==2) { cout<<"What is your favorite indoor game"<<endl; cout<<"Press 1 for Ludo"<<endl; cout<<"Press 2 for Chess"<<endl; cout<<"Press 3 for Cards"<<endl;…arrow_forwardQ No 3. #include <string> using namespace std; class Account { public: Account(string accountName, int initialBalance) { name=accountName; if (initialBalance > 0) { balance = initialBalance; } } void deposit(int depositAmount) { if (depositAmount > 0) { balance = balance + depositAmount; } } int getBalance() const { return balance; } void setName(string accountName) { name = accountName; } string getName() const { return name; } private: string name; int balance; }; Rewrite the following code in correct form. a) Assume the following prototype of destructor is declared in class Time: void ~Time(int); b) Assume the following prototype of constructor is declared in class Employee: int Employee(string, string);…arrow_forward
- struct gameType { string title; int numPlayers; bool online; }; gameType Awesome[50]; Write the C++ statements that print out all gameType fields from Awesome whose numPlayers is > 1.arrow_forwardLook at the following declaration:enum Flower { Rose, Daisy, Petunia } What is the name of the data type?arrow_forwardIn Pascal language When you have declared an enumerated type, you can declare variables of which type?arrow_forward
- Computer Science C++ Help Write the declaration for a stuct that has the following public data memebers: a string for the name of an insured person, a string for a policy number, floating- point numbers for the insurance amount and premium amount, and an integer for a building type code. Following your struct declaration, write the declaration for stuct varaible and your stuct typearrow_forwarddebugarrow_forwardvoid funOne(int a, int& b, char v); void main() { int num1=10; char ch='A'; funOne(num1, 15,ch)<arrow_forward#include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward#include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forward#include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
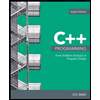
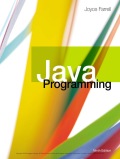