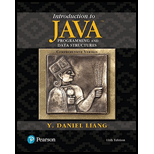
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 7.5, Problem 7.5.1CP
Use the arraycopy method to copy the following array to a target array t :
int[] source = {3 , 4 , 5};
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Use the arraycopy method to copy the following array to a target array t: int[] source = {3, 4, 5};
Print the elements of the array ‘a’ using the mentioned notations:
int a[]={1,2,3,4,5};
int *p;
p = a;
for (int i = 0; i< 5; i++)
{
//Subscript notation with name of array
//Subscript notation with pointer 'p'
//Offset notation using array name
//Offset notation using pointer 'p'
}
//convert array of numbers from farenheit to celcius// example:// argument: [23, 32, 41, 50, 59]// return: [-5, 0, 5, 10, 15]// hint: use Array.mapfunction toCelcius(array){
}
//write a function that takes an input array and returns an array of booleans (>=75) or fail (<75)// example:// argument: [20, 30, 50, 80, 90, 100]// return: [false, false, false, true, true, true]// hint: use Array.mapfunction passOrFail(array){
}
Chapter 7 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 7.2 - Prob. 7.2.1CPCh. 7.2 - Prob. 7.2.2CPCh. 7.2 - What is the output of the following code? int x =...Ch. 7.2 - Indicate true or false for the following...Ch. 7.2 - Which of the following statements are valid? a....Ch. 7.2 - Prob. 7.2.6CPCh. 7.2 - What is the array index type? What is the lowest...Ch. 7.2 - Write statements to do the following: a. Create an...Ch. 7.2 - What happens when your program attempts to access...Ch. 7.2 - Identify and fix the errors in the following code:...
Ch. 7.2 - What is the output of the following code? 1....Ch. 7.4 - Will the program pick four random cards if you...Ch. 7.5 - Use the arraycopy method to copy the following...Ch. 7.5 - Prob. 7.5.2CPCh. 7.7 - Suppose the following code is written to reverse...Ch. 7.8 - Prob. 7.8.1CPCh. 7.8 - Prob. 7.8.2CPCh. 7.9 - Prob. 7.9.1CPCh. 7.9 - Prob. 7.9.2CPCh. 7.10 - If high is a very large integer such as the...Ch. 7.10 - Prob. 7.10.2CPCh. 7.10 - Prob. 7.10.3CPCh. 7.11 - Prob. 7.11.1CPCh. 7.11 - How do you modify the selectionSort method in...Ch. 7.12 - What types of array can be sorted using the...Ch. 7.12 - To apply java.util.Arrays.binarySearch (array,...Ch. 7.12 - Show the output of the following code: int[] list1...Ch. 7.13 - This book declares the main method as public...Ch. 7.13 - Show the output of the following program when...Ch. 7 - (Assign grades) Write a program that reads student...Ch. 7 - (Reverse the numbers entered) Write a program that...Ch. 7 - (Count occurrence of numbers) Write a program that...Ch. 7 - (Analyze scores) Write a program that reads an...Ch. 7 - (Print distinct numbers) Write a program that...Ch. 7 - (Revise Listing 5.1 5, PrimeNumber.java) Listing...Ch. 7 - (Count single digits) Write a program that...Ch. 7 - (Average an array) Write two overloaded methods...Ch. 7 - (Find the smallest element) Write a method that...Ch. 7 - Prob. 7.10PECh. 7 - (Statistics: compute deviation) Programming...Ch. 7 - (Reverse an array) The reverse method in Section...Ch. 7 - Prob. 7.13PECh. 7 - Prob. 7.14PECh. 7 - 7 .15 (Eliminate duplicates) Write a method that...Ch. 7 - (Execution time) Write a program that randomly...Ch. 7 - Prob. 7.17PECh. 7 - (Bubble sort) Write a sort method that uses the...Ch. 7 - (Sorted?) Write the following method that returns...Ch. 7 - (Revise selection sort) In Listing 7 .8, you used...Ch. 7 - (Sum integers) Write a program that passes an...Ch. 7 - (Find the number of uppercase letters in a string)...Ch. 7 - (Game: locker puzzle) A school bas 100 lockers and...Ch. 7 - (Simulation: coupon collectors problem) Coupon...Ch. 7 - (Algebra: solve quadratic equations) Write a...Ch. 7 - (Strictly identical arrays) The arrays 1ist1 and...Ch. 7 - (Identical arrays) The arrays 1ist1 and 1ist2 are...Ch. 7 - (Math: combinations) Write a program that prompts...Ch. 7 - (Game: pick four cards) Write a program that picks...Ch. 7 - (Pattern recognition: consecutive four equal...Ch. 7 - (Merge two sorted Lists) Write the following...Ch. 7 - (Partition of a list) Write the following method...Ch. 7 - Prob. 7.33PECh. 7 - (Sort characters in a string) Write a method that...Ch. 7 - (Game: hangman) Write a hangman game that randomly...Ch. 7 - (Game: Eight Queens) The classic Eight Queens...Ch. 7 - Prob. 7.37PE
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Average Rainfall Write a program that uses nested loops to collect data and calculate the average rainfall over...
Starting Out with Python (4th Edition)
When displaying a Java applet, the browser invokes the _____ to interpret the bytecode into the appropriate mac...
Web Development and Design Foundations with HTML5 (8th Edition)
The size, shape, color and weight of an object are considered of the objects class.
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
The input operation that appears just before a validation loop is known as the a. prevalidation read b. primord...
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
In Exercises 55 through 60, find the value of the given function where a and b are numeric variables of type Do...
Introduction To Programming Using Visual Basic (11th Edition)
Determine the resultant internal normal force, shear force, and bending moment at point C in the beam.
Mechanics of Materials (10th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 1. Shift Left k Cells Use Python Consider an array named source. Write a method/function named shiftLeft( source, k) that shifts all the elements of the source array to the left by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftLeft(source,3) After calling shiftLeft(source,3), printing the array should give the output as: [ 40, 50, 60, 0, 0, 0 ]arrow_forwardCode for this in C: You have created three arrays:array A of size 100array B of size 50array C of size 200You are to store the first 100 numbers (1,2,3,...,100) into array A, the first 50 positive odd numbers (1,3,5,...,) into array B, and the reciprocal of each position [C(5) = 1/5] into array C. Then, output the contents of each array.arrow_forwardint[] cars = {1,2,3,4,5}; for (int i=0; iarrow_forwardThe array index can be any integer less than the array size… TRUE OR FALSEarrow_forwardCode Big Oh Notation my array = array(ï,[1,2,3,4,5|) for i in my_array print(i) 1. Code Big Oh Notation _print("Step 2") print(my_array[3]) 2. Code Big Oh Notation 3. print("Step 3") my_array.append(6) print(my array)arrow_forwardSales.java contains a Java program that prompts for and reads in the sales for each of 5 salespeople in a company. Now modify the program as follows: Declare an array called sales to save the sales of 5 salespeople. Complete the header of the first for loop. This loop initializes the array. Complete the header of the second for loop. This loop computes the total of array elements. Add code to compute and print the average sale. Add another loop to print the id of each salesperson and the number of their sales. The salespeople are objecting to having an id of 0—no one wants that designation. Modify your program so that the IDs run from 1-5 instead of 0-4. // *************************************************************** // Sales.java // // Reads in and stores sales for each of 5 salespeople. Displays // sales entered by salesperson id and total sales for all salespeople. // // *************************************************************** import java.util.Scanner;…arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
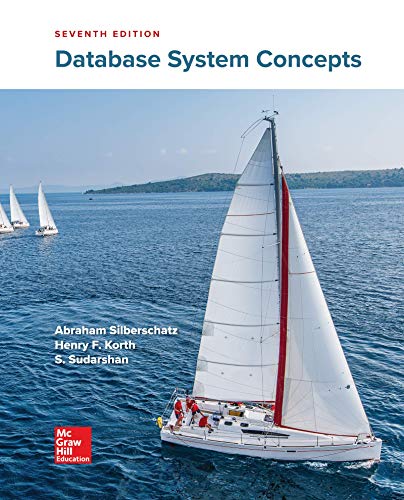
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
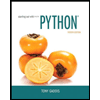
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
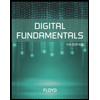
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
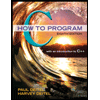
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
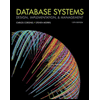
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
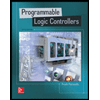
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
9.1: What is an Array? - Processing Tutorial; Author: The Coding Train;https://www.youtube.com/watch?v=NptnmWvkbTw;License: Standard Youtube License