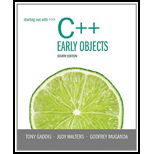
Write four lines of code that might appear in a client
Define an instance of the Pizza class named myPizza.
Call a Pizza function to set the price.
Call a Pizza function to set the size (i.e., the radius).
Call a Pizza function to return the price per square inch and then print it.

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Starting Out with C++: Early Objects
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Modern Database Management
SURVEY OF OPERATING SYSTEMS
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Management Information Systems: Managing The Digital Firm (16th Edition)
- In C++ create a program that acts like a bank account. That has 3 classes. The first class must be a generic class that holds basic info deposit, withdraw, calculate monthly interest rate, and a monthly service fee.The second class must be a savings account class that if the savings account falls below $25 it becomes inactive. The final class must be a checking account if someone withdraws more than what they have a service charge of $15 is added and go into a negative balance. The output should display the basic information how much someone deposited or withdrew and their monthly interest rate.arrow_forwardYou need 5 java classes and these include Netflix Class (Parent class of Movie and TVShow class) Movie Class TVShow Class Test/TestDriver Class Database Class Please read the questions carefully. TestDriver is given for the other image.arrow_forwardPYTHON PROGRAMMING ONLY NEED HELParrow_forward
- In C++ create a program that acts like a bank account. It asks the user whether to deposit or withdraw. That has 3 classes. The first class must be a generic class that holds basic info deposit, withdraw, calculate monthly interest rate, and a monthly service fee.The second class must be a savings account class that if the savings account falls below $25 it becomes inactive. The final class must be a checking account if someone withdraws more than what they have a service charge of $15 is added and go into a negative balance. The output should display the basic information how much someone deposited or withdrew and their monthly interest rate.arrow_forwardIn C++ create a program that acts like a bank account. It prompts the user for their input whether to deposit or withdraw. That has 3 classes. The first class must be a generic class that holds basic info deposit, withdraw, calculate monthly interest rate, and a monthly service fee.The second class must be a savings account class that if the savings account falls below $25 it becomes inactive. The final class must be a checking account if someone withdraws more than what they have a service charge of $15 is added and go into a negative balance. The output should display the basic information how much someone deposited or withdrew and their monthly interest rate.arrow_forwardAssignment 1. You are to write a simple program with two classes. One controller class and a class to hold your object definition. (Similar to what we will use in class) 2. Read in the following from the JOptionPane input window: a. Customer First Name b. Customer Last Name c. Customer Phone Number (example: 9093873744) d. Number of Vehicles to be manufactured (example: 5) e. Number of fuel tanks to be mounted on the vehicle. 3. Make sure the following is entered correctly, otherwise display an error message and ask the user to re-enter the data: a. The first name of the customer is not blank b. The last name of the customer is not blank c. The phone number of the customer is not blank and is 10 characters d. The number of vehicles is between 1 and 10 (includes 1 and 10) e. The number of fuel tanks can only be (2,4,8,10, 15,20) 4. Compute the cost for manufacturing vehicles using the price of $500.19 per vehicle 5. Compute the cost for fuel tanks using the price of $2.15 per fuel cell.…arrow_forward
- Implement functions and expressions into your existing code: In your game console class add a start function. Start function will prompt user for an email address. Use a regular expression to validate that its a valid email address and prompt again until they enter valid one. Next, prompt user to enter credit card number. Use a regular expression to validate the credit card. Prompt them again until they enter a valid one. Existing Code: class Game {constructor(name){this.name = name;}} class GameConsole{constructor(){this.games = [];} load(){var gameNames = ["Zelda", "Halo", "Mario", "The God Among Us\n"];for (var i = 0; i < gameNames.length; i++) {let game = new Game(gameNames[i]);this.games.push(game);}} log(){console.log("Games Loaded:");for (var i = 0; i < this.games.length; i++) {var name = this.games[i].name;console.log(name);}}} let gamecon = new GameConsole();gamecon.load();gamecon.log(); const prompt = require('prompt-sync')(); var adventurersName = ["Captain Thomas…arrow_forwardImplement a class called Account which defines a modified banking account that can be created with a given balance and account name. It must be able to perform the following operations in the public interface: - Accepts an amount to deposit the balance - Withdraws an amount from the balance - Returns its balance - Returns its name - Displays its name and balance Write a Java application called AccountSystem to simulate an instance of the class. You need to use deposit and withdrawal methods in this program.arrow_forwardProblem C • -3: method consonants() had more than one loop • -3: method initials () had more than one loop • -3: at least one method used nested loopsarrow_forward
- In C++ Create a class called Pencil. Pencil has a type. Create another class called Customer. The customer class should have a ID number, array (using pointers) called purchases, and a Pencil variable. Make sure to create the purchases array in the constructor. Create a function to fill in purchase amount. Create a way to output all the information about a customer, including all the details about their type of pencil.arrow_forwardIN C++, Create a class called Invoice that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four pieces of information as instance variables - a part number (type String), a part description (type String), a quantity of the item being purchased (type int) and a price per item (double). Your class should have a constructor that initializes the four instance variables. Provide a set and a get method for each instance variable. In addition, provide a method named getInvoiceAmount that calculates the invoice amount (i.e., multiplies the quantity by the price per item), then returns the amount as a double value. If the quantity is not positive, it should be set to 0. If the price per item is not positive, it should be set to0. Write a test app named InvoiceTest that demonstrates class Invoice's capabilities.arrow_forwardUsing c++ programming language. Write a class Distance that holds distances or measurements expressed in feets and inches. Thisclass has two private data members: feet: An integer that holds the feet. inches: An integer that holds the inches. Write a constructor with default parameters that initializes each data member of the class.If inches are greater than equal to 12 then they must be appropriately converted tocorresponding feet. Generate appropriate getter-setter functions for the data members.o void setFeet(int f) and int getFeet()consto void setInches(int i) It should ensure proper conversion to feet.o int getInches() const Define an operator ‘+’ that overloads the standard ‘+’ math operator and allows oneDistance object to be added to another. Distance operator+ (const Distance &obj). Define an operator - function that overloads the standard ‘-‘ math operator and allowssubtracting one Distance object from another. Distance operator-(const Distance &obj)…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
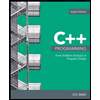