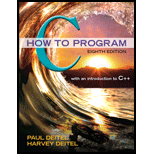
Answer each of the following:
- The _____ operator returns the location in memory where its operand is stored.
- The _____ operator returns the value of the object to which its operand points.
- To accomplish pass-by-reference when passing a nonarray variable to a function, it’s necessary to pass the _____ of the variable to the function.
(a)

To find the name of the operator that returns the memory location of an operand.
& operator.
Explanation of Solution
In C programming, language and symbol are used to get the address of an operand. The symbol (&) is also known as the ‘Address of’ operator.
Sample Program:
//include essential header files #include <stdio.h> //main function intmain() { //declare an operand int sample; //Print the address of operand named sample printf("%p",&sample); return0; }
Sample output:
(b)

To find the name of the operator that returns the value of an object who points to other operands.
* operator.
Explanation of Solution
In C programming language, * symbol is used to get the value of other operands that address it points.
Sample Program:
//include essential header files #include <stdio.h> //main function intmain() { //declare a operand int sample =10; //declare a pointer type operand int*point; point =&sample; //Print the value printf("%d",*point); return0; }
Sample output:
(c)

To find the component of a variable that should be passed to a function to accomplish pass by reference.
address.
Explanation of Solution
In pass by reference, any changes that a function performs on the passed variable always gets reflected in the actual variable. For this to accomplish, the address of the variable must be passed to function.
Sample Program:
//include essential header files #include <stdio.h> //function defination void passRef(int*a,int*b) { //declare a temp variable int temp; //assign variable a value to temp variable temp =*a; //assign variable b value to variable a *a =*b; //assign variable temp value to variable b *b = temp; //return the value return; } //main function intmain() { //declare variable int sample1 =10; int sample2 =15; //calling function using address of sample1 and sample2 passRef(&sample1,&sample2); //Print the values after swapping printf("sample1 = %d sample2 = %d", sample1, sample2); return0; }
Sample output:
Want to see more full solutions like this?
Chapter 7 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Vector Mechanics for Engineers: Statics
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out with Python (4th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Modern Database Management
- I'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forwardThe knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges (missing values vs. missing labels), they should not be combined in the same diagram. Instead, create two separate diagrams for clarity. For reference, I will attach a second image…arrow_forwardNote : please avoid using AI answer the question by carefully reading it and provide a clear and concise solutionHere is a clear background and explanation of the full method, including what each part is doing and why. Background & Motivation Missing values: Some input features (sensor channels) are missing for some samples due to sensor failure or corruption. Missing labels: Not all samples have a ground-truth RUL value. For example, data collected during normal operation is often unlabeled. Most traditional deep learning models require complete data and full labels. But in our case, both are incomplete. If we try to train a model directly, it will either fail to learn properly or discard valuable data. What We Are Doing: Overview We solve this using a Teacher–Student knowledge distillation framework: We train a Teacher model on a clean and complete dataset where both inputs and labels are available. We then use that Teacher to teach two separate Student models: Student A learns…arrow_forward
- Here is a clear background and explanation of the full method, including what each part is doing and why. Background & Motivation Missing values: Some input features (sensor channels) are missing for some samples due to sensor failure or corruption. Missing labels: Not all samples have a ground-truth RUL value. For example, data collected during normal operation is often unlabeled. Most traditional deep learning models require complete data and full labels. But in our case, both are incomplete. If we try to train a model directly, it will either fail to learn properly or discard valuable data. What We Are Doing: Overview We solve this using a Teacher–Student knowledge distillation framework: We train a Teacher model on a clean and complete dataset where both inputs and labels are available. We then use that Teacher to teach two separate Student models: Student A learns from incomplete input (some sensor values missing). Student B learns from incomplete labels (RUL labels missing…arrow_forwardhere is a diagram code : graph LR subgraph Inputs [Inputs] A[Input C (Complete Data)] --> TeacherModel B[Input M (Missing Data)] --> StudentA A --> StudentB end subgraph TeacherModel [Teacher Model (Pretrained)] C[Transformer Encoder T] --> D{Teacher Prediction y_t} C --> E[Internal Features f_t] end subgraph StudentA [Student Model A (Trainable - Handles Missing Input)] F[Transformer Encoder S_A] --> G{Student A Prediction y_s^A} B --> F end subgraph StudentB [Student Model B (Trainable - Handles Missing Labels)] H[Transformer Encoder S_B] --> I{Student B Prediction y_s^B} A --> H end subgraph GroundTruth [Ground Truth RUL (Partial Labels)] J[RUL Labels] end subgraph KnowledgeDistillationA [Knowledge Distillation Block for Student A] K[Prediction Distillation Loss (y_s^A vs y_t)] L[Feature Alignment Loss (f_s^A vs f_t)] D -- Prediction Guidance --> K E -- Feature Guidance --> L G --> K F --> L J -- Supervised Guidance (if available) --> G K…arrow_forwarddetails explanation and background We solve this using a Teacher–Student knowledge distillation framework: We train a Teacher model on a clean and complete dataset where both inputs and labels are available. We then use that Teacher to teach two separate Student models: Student A learns from incomplete input (some sensor values missing). Student B learns from incomplete labels (RUL labels missing for some samples). We use knowledge distillation to guide both students, even when labels are missing. Why We Use Two Students Student A handles Missing Input Features: It receives input with some features masked out. Since it cannot see the full input, we help it by transferring internal features (feature distillation) and predictions from the teacher. Student B handles Missing RUL Labels: It receives full input but does not always have a ground-truth RUL label. We guide it using the predictions of the teacher model (prediction distillation). Using two students allows each to specialize in…arrow_forward
- We are doing a custom JSTL custom tag to make display page to access a tag handler. Write two custom tags: 1) A single tag which prints a number (from 0-99) as words. Ex: <abc:numAsWords val="32"/> --> produces: thirty-two 2) A paired tag which puts the body in a DIV with our team colors. Ex: <abc:teamColors school="gophers" reverse="true"> <p>Big game today</p> <p>Bring your lucky hat</p> <-- these will be green text on blue background </abc:teamColors> Details: The attribute for numAsWords will be just val, from 0 to 99 - spelling, etc... isn't important here. Print "twenty-six" or "Twenty six" ... . Attributes for teamColors are: school, a "required" string, and reversed, a non-required boolean. - pick any four schools. I picked gophers, cyclones, hawkeyes and cornhuskers - each school has two colors. Pick whatever seems best. For oine I picked "cyclones" and red text on a gold body - if…arrow_forwardI want a database on MySQL to analyze blood disease analyses with a selection of all its commands, with an ER drawing, and a complete chart for normalization. I want them completely.arrow_forwardAssignment Instructions: You are tasked with developing a program to use city data from an online database and generate a city details report. 1) Create a new Project in Eclipse called "HW7". 2) Create a class "City.java" in the project and implement the UML diagram shown below and add comments to your program. 3) The logic for the method "getCityCategory" of City Class is below: a. If the population of a city is greater than 10000000, then the method returns "MEGA" b. If the population of a city is greater than 1000000 and less than 10000000, then the method returns "LARGE" c. If the population of a city is greater than 100000 and less than 1000000, then the method returns "MEDIUM" d. If the population of a city is below 100000, then the method returns "SMALL" 4) You should create another new Java program inside the project. Name the program as "xxxx_program.java”, where xxxx is your Kean username. 3) Implement the following methods inside the xxxx_program program The main method…arrow_forward
- CPS 2231 - Computer Programming – Spring 2025 City Report Application - Due Date: Concepts: Classes and Objects, Reading from a file and generating report Point value: 40 points. The purpose of this project is to give students exposure to object-oriented design and programming using classes in a realistic application that involves arrays of objects and generating reports. Assignment Instructions: You are tasked with developing a program to use city data from an online database and generate a city details report. 1) Create a new Project in Eclipse called "HW7”. 2) Create a class "City.java" in the project and implement the UML diagram shown below and add comments to your program. 3) The logic for the method "getCityCategory" of City Class is below: a. If the population of a city is greater than 10000000, then the method returns "MEGA" b. If the population of a city is greater than 1000000 and less than 10000000, then the method returns "LARGE" c. If the population of a city is greater…arrow_forwardPlease calculate the average best-case IPC attainable on this code with a 2-wide, in-order, superscalar machine: ADD X1, X2, X3 SUB X3, X1, 0x100 ORR X9, X10, X11 ADD X11, X3, X2 SUB X9, X1, X3 ADD X1, X2, X3 AND X3, X1, X9 ORR X1, X11, X9 SUB X13, X14, X15 ADD X16, X13, X14arrow_forwardOutline the overall steps for configuring and securing Linux servers Consider and describe how a mixed Operating System environment will affect what you have to do to protect the company assets Describe at least three technologies that will help to protect CIA of data on Linux systemsarrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
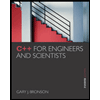
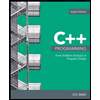
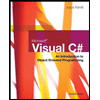
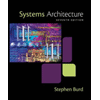
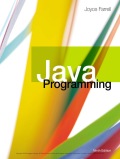