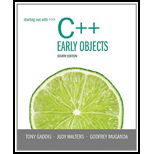
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 6.5, Problem 6.9CP
What is the output of the following
#include <iostream>
using namespace std;
void fund1(double, int); // Function prototype
int main()
int x = 0;
double y = 1.5;
cout << x << " " << y << endl;
func1(y, x);
cout << x << " " << y << endl;
return 0;
}
void fund (double a. int b)
{
cout ,<< a << " " << b << endl;
a = 0.0;
b = 10;
cout << a << " " << b << endl:
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Solution by C++
C++
2.A. Is it possible to pass variable y as parameter to the functions?
2.B. Supply the missing code stated in TODO at line 17-19.
2.C Supply the missing code stated in TODO at line 21-22.
2.D. (Assuming the code is complete) What will be the display of this program?
Chapter 6 Solutions
Starting Out with C++: Early Objects
Ch. 6.2 - Prob. 6.1CPCh. 6.2 - Prob. 6.2CPCh. 6.2 - Prob. 6.3CPCh. 6.2 - Prob. 6.4CPCh. 6.5 - Indicate which of the following is the function...Ch. 6.5 - Prob. 6.6CPCh. 6.5 - Prob. 6.7CPCh. 6.5 - Prob. 6.8CPCh. 6.5 - What is the output of the following program?...Ch. 6.5 - The following program skeleton asks for the number...
Ch. 6.8 - How many return values may a function have?Ch. 6.8 - Write a header for a function named distance. The...Ch. 6.8 - Write a header for a function named days. The...Ch. 6.8 - Prob. 6.14CPCh. 6.8 - Write a header for a function named lightYears....Ch. 6.11 - What is the difference between a static local...Ch. 6.11 - Prob. 6.17CPCh. 6.11 - Prob. 6.18CPCh. 6.13 - Prob. 6.19CPCh. 6.13 - Write the prototype and header for a function...Ch. 6.13 - Write the prototype and header for a function...Ch. 6.13 - What is the output of the following program?...Ch. 6.13 - The following program asks the user to enter two...Ch. 6.15 - Is it required that overloaded functions have...Ch. 6.15 - What is the output of the following program code?...Ch. 6.15 - What is the output of the following program code?...Ch. 6 - The ____ is the part of a function definition that...Ch. 6 - If a function doesnt return a value, the word...Ch. 6 - If function showValue has the following header:...Ch. 6 - Either a functions ____ or its ____ must precede...Ch. 6 - Values that are sent into a function are called...Ch. 6 - Special variables that hold copies of function...Ch. 6 - When only a copy of an argument is passed to a...Ch. 6 - A(n)_____ eliminates the need to place a function...Ch. 6 - A(n)_____ variable is defined inside a function...Ch. 6 - ____ variables are defined outside all functions...Ch. 6 - _____ variables provide an easy way to share large...Ch. 6 - Unless you explicitly initialize numeric global...Ch. 6 - If a function has a local variable with the same...Ch. 6 - ______ local variables retain their value between...Ch. 6 - The _____ statement causes a function to end...Ch. 6 - ______ arguments are passed to parameters...Ch. 6 - When a function uses a mixture of parameters with...Ch. 6 - Prob. 18RQECh. 6 - When used as parameters, _______ variables allow a...Ch. 6 - Reference variables are defined like regular...Ch. 6 - Reference variables allow arguments to be passed...Ch. 6 - The ________ function causes a program to...Ch. 6 - Two or more functions may have the same name, as...Ch. 6 - What is the advantage of breaking your...Ch. 6 - What is the difference between an argument and a...Ch. 6 - When a function accepts multiple arguments, does...Ch. 6 - What does it mean to overload a function?Ch. 6 - If you are writing a function that accepts an...Ch. 6 - Give an example of where an argument should he...Ch. 6 - How do you return a value from a function?Ch. 6 - Prob. 31RQECh. 6 - Prob. 32RQECh. 6 - The following statement calls a function named...Ch. 6 - A program contains the following function, int...Ch. 6 - Write a function, named timesTen, that accepts an...Ch. 6 - A program contains the following function. void...Ch. 6 - Write a function named getNumber, which uses a...Ch. 6 - Write a function named biggest that receives three...Ch. 6 - Prob. 39RQECh. 6 - Markup Write a program that asks the user to enter...Ch. 6 - Celsius Temperature Table The formula for...Ch. 6 - Falling Distance The following formula can be used...Ch. 6 - Kinetic Energy In physics, an object that is in...Ch. 6 - Winning Division Write a program that determines...Ch. 6 - Prob. 6PCCh. 6 - Lowest Score Drop Write a program that calculates...Ch. 6 - Star Search A particular talent competition has...Ch. 6 - isPrime Function A prime number is an integer...Ch. 6 - Present Value Suppose you want to deposit a...Ch. 6 - Stock Profit The profit from the sale of a stock...Ch. 6 - Multiple Stock Sales Use the function that you...Ch. 6 - Order Status The Middletown Wholesale Copper Wire...Ch. 6 - Overloaded Hospital Write a program that computes...Ch. 6 - Population In a population, the birth rate is the...Ch. 6 - Transient Population Modify Programming Challenge...Ch. 6 - Using FilesHospital Report Modify Programming...Ch. 6 - Group Project 20. Using FilesTravel Expenses This...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
Look at the following class definitions: class Vegetable { public virtual void message() { MessageBox. Show (I ...
Starting out with Visual C# (4th Edition)
When displaying a Java applet, the browser invokes the _____ to interpret the bytecode into the appropriate mac...
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Use what you've learned about the binary numbering system in this chapter to convert the following decimal numb...
Starting Out with Python (4th Edition)
Write a program to print the value of EOF.
C Programming Language
Why do many programming languages implement I/O operations as if they were calls to functions?
Computer Science: An Overview (12th Edition)
What is a control structure?
Starting Out with Python (3rd Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- C++ Functions provide a means to modularize applications Write a function called "Calculate" takes two double arguments returns a double result For example, the following is a function that takes a single "double" argument and returns a "double" result double squareArea(double side){ double lArea; lArea = side * side; return lArea;}arrow_forwardC++ Write a function for the function prototype int triple(int);. The function should return the cube of the parameter. int triple(int); // prototypearrow_forward6: Write a program in C++ that contains overloaded functions with name óvoperation(). When the main program makes a call to Ovoperation the program performs the following according to the arguments of the function call: • Ovoperation(): returns an int 0. • Ovoperation(float, float) returns the maximum between the arguments. • Ovoperation(int, int, int) returns the minimum value among the arguments. • Ovoperation{char) return the next ASCII character (e.g. OpOperation('a') returns b').arrow_forward
- 06: Write a program in C+ that contains overloaded functions with name Ovoperation(). When the main program makes a call to Ovoperation the program performs the following according to the arguments of the function call: • ovoperation(): returns an int 0. • Ovoperation(float, float) returns the maximum between the arguments. • Ovoperation(int, int, int) returns the minimum value among the arguments. • OvOperation(char) return the next ASCII character (eg. OpOperation('a) returns b'). int main () cout < Opoperation ('w')<< endl << Opoperation () < endl: cout « Opoperation (1.1,0.5) << endl << opoperation (4.-2,9): return 0;arrow_forwardQ6: Write a program in C++ that contains overloaded functions with name OvOperation(). When the main program makes a call to OvOperation the program performs the following according to the arguments of the function call: • OvOperation(): returns an int 0. • OvOperation(float, float) returns the maximum between the arguments. • OvOperation(int, int, int) returns the minimum value among the arguments. OvOperation(char) return the next ASCII character (e.g. OpOperation('a') returns 'b'). int main () cout << Opoperation ( 'w' )<< endl << Opoperation () « endl; cout << OpOperation (1.1,0.5) << endl << Opoperation (4,-2,9); return 0;arrow_forwardQ6: Write a program in C++ that contains overloaded functions with name OvOperation(). When the main program makes a call to OvOperation the program performs the following according to the arguments of the function call: OvOperation(): returns an int 0. OvOperation(float, float) returns the maximum between the arguments. OvOperation(int, int, int) returns the minimum value among the arguments. OvOperation(char) return the next ASCII character (e.g. OpOperation('a') returns 'bʻ).arrow_forward
- Exercise 4 The following codes will show the use of static specifier. #include using namespace std; int assignID (void) ; int assignIDnonstatic (void); void main (void) ( int i, id; cout << "Call a function with static variable\n"; for (i-0;i<5;i++) ( id = assignID(); cout << "Student ID #" << id << endl; cout << "\nCall a function with non static variable\n"; for (i-0;i<5;i++) ( id = assignIDnonstatic (); cout << "Student ID #" << id << endl; 1. system("pause"); int assignID (veid) static int ID=03; ID++B return ID; int assignIDnonstatic (void) ( Ent ID=0: ID++: return ID;arrow_forwardstruct date{ int day; int month; int year; }; Write a function named void increaseDay(struct date *d) that increases the value of a variable of struct date type with integer year, month, and day members by one day. Write a function named void decreaseDay(struct date *d) that decreases the value of a variable of struct date type with integer year, month, and day members by one day. Write a C program that reads from the user a date in d/m/y format and the amount of increase or decrease as an integer. Display the new date in d/m/y format. You may call related functions as many as given increase or decrease value. Note 1: You do not need to consider leap years. Use always 28 days for month February. Note 2: Do not modify the function prototypes. Sample Input1: Sample Output1: 12/8/1990 -5 7/8/1990 Sample Input2: Sample Output2: 26/2/2005 5 3/3/2005 Sample Input3: Sample Output3: 29/12/1998 7 5/1/1999…arrow_forward06: Write a program in C++ that contains overloaded functions with name OvOperation(). When the main es a call to Ovoperation the program performs the following according to the arguments program makes. of the function call: • Ovoperation(): returns an int 0. • Ovoperation(float, float) returns the maximum between the arguments. • Ovoperation(int, int, int) returns the minimum value among the arguments. • Ovoperation(char) return the next ASCII character (eg. OpOperation('a') returns b').arrow_forward
- Programming Skills: Complete the function egg_category which returns a str describing an egg's category given its int weight in grams. Here is a table specifying the weight ranges if an egg's weight is on the boundary between two category ranges, it is assigned the smaller category. Category Small Meduim Weight no more than 50 grams 50-57 grams Category Large Jumbo Weight 57-64 grams more than 64 grams def egg_category (weight): %# Return a str describing the category of an egg of the specified int weight.arrow_forwardmodule 4: Categorize Functions and provide description of what each function can do.arrow_forwardExercise 5 #include using namespace std/ int squareByValue ( int ); // function prototypes void squareByReference ( int * ); // function prototype with pointer as argument int main () int x = 2, z = 4; cout << "x = " << x << " before squareByValue\n" << "Value returned by squareByValue: < squareByValue ( x ) << endl << "x = " << x << after squareByValue\n" << endl; cout << "z = " << z << " before squareByReference" <arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
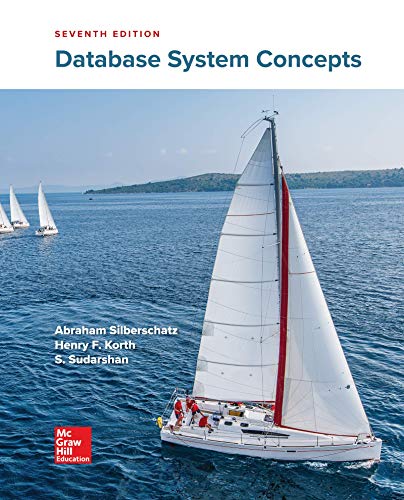
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
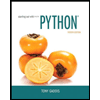
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
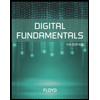
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
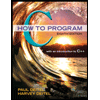
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
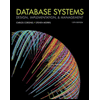
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
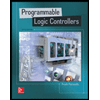
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
What Are Data Types?; Author: Jabrils;https://www.youtube.com/watch?v=A37-3lflh8I;License: Standard YouTube License, CC-BY
Data Types; Author: CS50;https://www.youtube.com/watch?v=Fc9htmvVZ9U;License: Standard Youtube License