Explanation of Solution
Output of the Program:
The given program has been added with comments and line number.
/*Include required variables*/
#include <iostream> //Line 1
using namespace std; //Line 2
/*Function prototype*/
void myFunc();//Line 3
/*Main function*/
int main()//Line 4
{//Line 5
/*Variable "var" is declared as "int" data type and it is assigned with the value "100"*/
int var = 100; //Line 6
/*Print the value*/
cout << var << endl; //Line 7
/*Call the function "myFunc()"*/
myFunc();//Line 8
/*Display the value of variable "val" after calling the function*/
cout << var << endl; //Line 9
/*Return the value 0*/
return 0; //Line 10
}//Line 11
/* Function definition */
void myFunc()//Line 12
{//Line 13
/*Variable "var" is declared as "int" data type and it is assigned with the value "50"*/
int var = 50; //Line 14
/* Print the value of the variable "var" */
cout << var << endl; //Line 15
}//Line 16
Explanation:
The above program explains the scope of the local variable...

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
STARTING OUT WITH C++ MPL
- #include<stdio.h> //Standard library math.h for Mathematics functions #include<math.h> //function declaration void function1(void); void function2(void); int main(void) { //calling both function function1(); function2(); printf("\nMain function for Ceil value: %f",ceil(4.5)); return0; } // Defining the first function void function1(void) { printf("\nFirst function for Square Root: %f",sqrt(91)); } //Defining the second function void function2(void) { printf("\nSecond function for Power: %f",pow(2,4)); } I need to do what the picture is asking for.arrow_forwardSolution by C++arrow_forward14. What is the value of a and b after the function call myfunction1(a,b) in the main function? void myfunction1(int a, int &b) { a+t; b++; int main() { int a=5,b=53; myfunction1(a,b): } a) a=5,b=5 b) a=5,b=6 c) a=6,b=5 d) a=6,b=6arrow_forward
- C++arrow_forwardSet-up and implementation code for a void function MaxYou are not required to write a complete C++ program but must write and submit just your responses to the four specific function related questions below: QC1: Write the heading for a void function called Max that has three intparameters: num1, num2 and greatest. The first two parameters receive data from the caller, and greatest is used to return a value as a reference parameter. Document the data flow of the parameters with appropriate comments*. QC2: Write the function prototype for the function in QC1. QC3: Write the function definition of the function in QC1 so that it returns the greatest of the two input parameters via greatest, a reference parameter. QC4: Add comments to the function definition* you wrote in QC3 that also states its precondition and postcondition.arrow_forwardmodule 4: Categorize Functions and provide description of what each function can do.arrow_forward
- Write a function for the function prototype int triple(int);. The function should réturn the cube of the parameter. int triple(int); // prototypearrow_forwardDefine the term void functions.arrow_forward#include using namespace std; || function declaration int max(int num1, int num2); int main () { // local variable declaration: int a = 100; int b = 200; int ret; I| calling a function to get max value. ret = max(a, b); cout num2) result = num1; else result = num2; return result; }arrow_forward
- C++ Functions provide a means to modularize applications Write a function called "Calculate" takes two double arguments returns a double result For example, the following is a function that takes a single "double" argument and returns a "double" result double squareArea(double side){ double lArea; lArea = side * side; return lArea;}arrow_forwardAn application that uses user-defined functions has to provide function prototypes.arrow_forward(3) Data type Dog: (a) Define data type Dog with a single value constructor that receives two strings and an integer as the dog's name, breed, and age respectively. (b) Define a function breed that receives a Dog and returns the string that represents the breed component.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
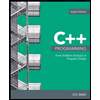