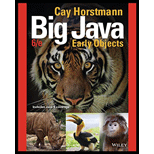
Explanation of Solution
a.
//Definition of class Test
class Test
{
//Definition of method main
public static void main(String[] args)
{
//Initialize the variables
String stars = "*****";
String stripes = "=====";
//Initialize the value of "i" to 0
int i = 0;
//Check the condition using while loop
while (i < 5)
{
//Print the output
System.out.println(stars.substring(0, i));
//Increment the value of "i"
i++;
}
}
}
Explanation:
The above code snippet initializes the variable “i” to “0”. The condition “i<=5” is checked using “while” loop. Print the output and increment the value of “i”.

Output:
*
**
***
****
Explanation of Solution
b.
//Definition of class Test
class Test
{
//Definition of method main
public static void main(String[] args)
{
//Initialize the variables
String stars = "*****";
String stripes = "=====";
//Initialize the value of "i" to 0
int i = 0;
//Check the condition using while loop
while (i < 5)
{
//Print the output
System.out.println(stars.substring(0, i));
//Print the output
System.out.println(stars.substring(i, 5));
//Increment the value of "i"
i++;
}
}
}
Explanation:
The above code snippet initializes the variable “i” to “0”. The condition “i<=5” is checked using “while” loop. Print the output by calling the two sub string function and increment the value of “i”.

Output:
*****
*
****
**
***
***
**
****
*
Explanation of Solution
c.
//Definition of class Main
class Main
{
//Definition of method main
public static void main(String[] args)
{
//Initialize the variables
String stars = "*****";
String stripes = "=====";
//Initialize the value of "i" to 0
int i = 0;
//Check the condition using while loop
while (i < 10)
{
//Check the condition for "i%2" equals to 0
if (i % 2 == 0)
{
//Print the output
System.out.println(stars);
}
else
{
//Print the output
System.out.println(stripes);
}
}
}
}
Explanation:
The above code snippet initializes the variable “i” to “0”. The condition “i<=10” is checked using “while” loop. Inside the while loop, check the condition of “i%2” using the “if” statement.

Output:
*****
*****
*****
*****
*****
*****
*****
.
.
.
.
Infinite loop
Want to see more full solutions like this?
Chapter 6 Solutions
Big Java, Binder Ready Version: Early Objects
- Question#2: Design and implement a Java program using Abstract Factory and Singleton design patterns. The program displays date and time in one of the following two formats: Format 1: Date: MM/DD/YYYY Time: HH:MM:SS Format 2: Date: DD-MM-YYYY Time: SS,MM,HH The following is how the program works. In the beginning, the program asks the user what display format that she wants. Then the program continuously asks the user to give one of the following commands, and performs the corresponding task. Note that the program gets the current date and time from the system clock (use the appropriate Java date and time operations for this). 'd' display current date 't': display current time 'q': quit the program. • In the program, there should be 2 product hierarchies: "DateObject” and “TimeObject”. Each hierarchy should have format and format2 described above. • Implement the factories as singletons. • Run your code and attach screenshots of the results. • Draw a UML class diagram for the program.arrow_forward#include <linux/module.h> #include <linux/kernel.h> // part 2 #include <linux/sched.h> // part 2 extra #include <linux/hash.h> #include <linux/gcd.h> #include <asm/param.h> #include <linux/jiffies.h> void print_init_PCB(void) { printk(KERN_INFO "init_task pid:%d\n", init_task.pid); printk(KERN_INFO "init_task state:%lu\n", init_task.state); printk(KERN_INFO "init_task flags:%d\n", init_task.flags); printk(KERN_INFO "init_task runtime priority:%d\n", init_task.rt_priority); printk(KERN_INFO "init_task process policy:%d\n", init_task.policy); printk(KERN_INFO "init_task task group id:%d\n", init_task.tgid); } /* This function is called when the module is loaded. */ int simple_init(void) { printk(KERN_INFO "Loading Module\n"); print_init_PCB(); printk(KERN_INFO "Golden Ration Prime = %lu\n", GOLDEN_RATIO_PRIME); printk(KERN_INFO "HZ = %d\n", HZ); printk(KERN_INFO "enter jiffies = %lu\n", jiffies); return 0; } /* This function is called when the…arrow_forwardList at least five Operating Systems you know. What is the difference between the kernel mode and the user mode for the Linux? What is the system-call? Give an example of API in OS that use the system-call. What is cache? Why the CPU has cache? What is the difference between the Static Linking and Dynamic Linking when compiling the code.arrow_forward
- In the GoF book, List interface is defined as follows: interface List { int count(); //return the current number of elements in the list Object get(int index); //return the object at the index in the list Object first(); //return the first object in the list Object last(); //return the last object in the list boolean include(Object obj); //return true is the object in the list void append(Object obj); //append the object to the end of the list void prepend(Object obj); //insert the object to the front of the list void delete(Object obj); //remove the object from the list void deleteLast(); //remove the last element of the list void deleteFirst(); //remove the first element of the list void deleteAll(); //remove all elements of the list (a) Write a class adapter to adapt Java ArrayList to GoF List interface. (b) Write a main program to test your adapters through List interface. (c) Same requirement as (a) and (b), but write an object adapter to adapt Java ArrayList to GoF List…arrow_forwardIn modern packet-switched networks, including the Internet, the source host segments long, application-layer messages (for example, an image or a music file) into smaller packets and sends the packets into the network. The receiver then reassembles the packets back into the original message. We refer to this process as message segmentation. Figure 1.27 (attached) illustrates the end-to-end transport of a message with and without message segmentation. Consider a message that is 106 bits long that is to be sent from source to destination in Figure 1.27. Suppose each link in the figure is 5 Mbps. Ignore propagation, queuing, and processing delays. a. Consider sending the message from source to destination without message segmentation. How long does it take to move the message from the source host to the first packet switch? Keeping in mind that each switch uses store-and-forward packet switching, what is the total time to move the message from source host to destination host? b. Now…arrow_forwardConsider a packet of length L that begins at end system A and travels over three links to a destination end system. These three links are connected by two packet switches. Let di, si, and Ri denote the length, propagation speed, and the transmission rate of link i, for i = 1, 2, 3. The packet switch delays each packet by dproc. Assuming no queuing delays, in terms of di, si, Ri, (i = 1, 2, 3), and L, what is the total end-to-end delay for the packet? Suppose now the packet is 1,500 bytes, the propagation speed on all three links is 2.5 * 10^8 m/s, the transmission rates of all three links are 2.5 Mbps, the packet switch processing delay is 3 msec, the length of the first link is 5,000 km, the length of the second link is 4,000 km, and the length of the last link is 1,000 km. For these values, what is the end-to-end delay?arrow_forward
- how to know the weight to data and data to weight also weight by infomraion gain in rapid miner , between this flow diagram retrieve then selecte attrbuite then set role and split data and decision tree and apply model and peformance ,please show how the operators should be connected:arrow_forwardusing rapid miner how to creat decison trea for all attribute and another one with delete one or more of them also how i know the weight of each attribute and what that mean in impact the resultarrow_forwardQ.1. Architecture performance [10 marks] Answer A certain microprocessor requires either 2, 4, or 6 machine cycles to perform various operations. ⚫ (40+g+f)% require 2 machine cycles, ⚫ (30-g) % require 4 machine cycles, and ⚫ (30-f)% require 6 machine cycles. (a) What is the average number of machine cycles per instruction for this microprocessor? Answer (b) What is the clock rate (machine cycles per second) required for this microprocessor to be a "1000 MIPS" processor? Answer (c) Suppose that 35% of the instructions require retrieving an operand from memory which needs an extra 8 machine cycles. What is the average number of machine cycles per instruction, including the instructions that fetch operands from memory?arrow_forward
- Q.2. Architecture performance [25 marks] Consider two different implementations, M1 and M2, of the same instruction set. M1 has a clock rate of 2 GHz and M2 has a clock rate of 3.3 GHz. There are two classes of instructions with the following CPIs: Class A CPI for M1 CPI for M2 2.f 1.g B 5 3 C 6 4 Note that the dots in 2 fand 1.g indicate decimal points and not multiplication. a) What are the peak MIPS performances for both machines? b) Which implementation is faster, if half the instructions executed in a certain program are from class A, while the rest are divided equally among classes B and C. c) What speedup factor for the execution of class-A instructions would lead to 20% overall speedup? d) What is the maximum possible speedup that can be achieved by only improving the execution of class-A instructions? Explain why. e) What is the clock rate required for microprocessor M1 to be a "1000 MIPS" (not peak MIPS) processor?arrow_forwardPLEASE SOLVE STEP BY STEP WITHOUT ARTIFICIAL INTELLIGENCE OR CHATGPT I don't understand why you use chatgpt, if I wanted to I would do it myself, I need to learn from you, not from being a d amn robot. SOLVE STEP BY STEP I WANT THE DIAGRAM PERFECTLY IN SIMULINKarrow_forwardI need to develop and run a program that prompts the user to enter a positive integer n, and then calculate the value of n factorial n! = multiplication of all integers between 1 and n, and print the value n! on the screen. This is for C*.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
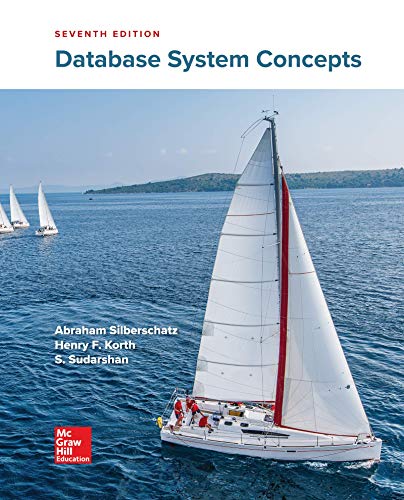
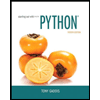
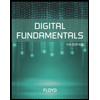
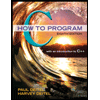
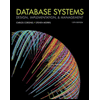
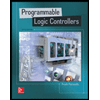