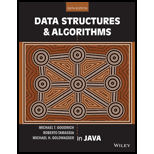
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 6, Problem 1R
Program Plan Intro
Stack:
- Stack is a non-primitive linear data structure.
- It is a collection of data of any type where insertion and deletion of data take place at one end called as top of the stack.
- As all the insertion and deletion happens from top of the stack or at one point, stack is also known as Last In First Out (LIFO) type of data structure.
Expert Solution & Answer

Explanation of Solution
Stack Operations:
Operations performed on the stack are as follows:
- PUSH
- Adds item to the collection and increases size of the stack.
- POP
- Returns the last item from collection and decreases the size of the stack.
- TOP
- Returns last item from the collection without removing it.
Current size of the Stack, S:
If 3 POP operations returned null, then only 7 POP operations out of 10 decrease the size of the stack. Therefore, the current size of stack is 18 elements since PUSH adds elements, TOP doesn't add or remove elements, and POP removes elements.
Want to see more full solutions like this?
Subscribe now to access step-by-step solutions to millions of textbook problems written by subject matter experts!
schedule02:20
Students have asked these similar questions
Suppose an initially empty stack S has performed a total of 50 push operations, 31 peek operations, and 32 pop operations, 7 of which return to indicate an empty stack. What is the current size of S?
You are allowed to operate on a stack WORK and a temporary stack TEMP (ifneeded) supporting their ADT operations of PUSH (S,X), POP (S, X) andEMPTYSTACK (S) only, where X represents an element/variable to be pushed in orpopped out of the stack and S represents a stack. You are also permitted to use onevariable if needed to carry out the operations.i) Given n distinct random numbers that are to be pushed into WORK, how canyou find the minimum element that was pushed into it? You are permitted to use alone variable.ii) Given n distinct random numbers that are to be pushed into WORK, how canyou find the maximum element that was pushed into it, all the while ensuring thatthe elements stored in WORK are in their descending order with the maximumelement beginning at the bottom of stack? You are permitted to use a lone variableand a temporary stack TEMP.iii) Given an array A[1: n] of distinct random numbers how can you obtain thesorted list in the array, making use of stacks alone?
You are allowed to operate on a stack WORK and a temporary stack TEMP (ifneeded) supporting their ADT operations of PUSH (S,X), POP (S, X) andEMPTYSTACK (S) only, where X represents an element/variable to be pushed in orpopped out of the stack and S represents a stack. You are also permitted to use one variable if needed to carry out the operations.
i) Given n distinct random numbers that are to be pushed into WORK, how canyou find the maximum element that was pushed into it, all the while ensuring thatthe elements stored in WORK are in their descending order with the maximumelement beginning at the bottom of stack? You are permitted to use a lone variableand a temporary stack TEMP.ii) Given an array A[1: n] of distinct random numbers how can you obtain thesorted list in the array, making use of stacks alone?
Chapter 6 Solutions
Data Structures and Algorithms in Java
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- You may only operate on the stacks WORK and TEMP, supporting their ADT operations of PUSH (S, X), POP (S, X), and EMPTYSTACK (S), where X is an element or variable to be pushed into or popped out of the stack and S denotes the stack itself. If necessary to complete the procedures, you may also utilise one variable. i) How can you determine the least element that was pushed into WORK given n different random integers that are going be placed into it? You are allowed to employ a single variable. ii) How can you determine the maximum element that was put into Work, given n different random integers, while making sure that the items placed in Work are arranged in ascending order, with the maximum element starting at the bottom of the stack? You are allowed to utilise the temporary stack TEMP as well as a single variable. iii) How can you create the sorted list in the array using just stacks given an array A[1: n] of unique random numbers?arrow_forwardCould you please clarify the difference between the limited version of the stack and the unbounded version of the stack?arrow_forwardIn Go Lang 4. Program stack. For the following code, answer the following questions. Assume we are putting everything for our function calls on the stack. · Show what a stack frame/activation record for main() looks like · Show what the stack frame/activation record for the 2nd call to ctTarg look like? (Yes, this means you can skip the other stack frames) · We note that targ does not change value in any recursive call. Why doesn't the compiler just store targ once in one block of memory big enough to store a string? int ctTarg(string* list, int len, string targ) { if (len <= 0) return 0; if (*list == targ) return 1 + ctTarg(list + 1, len - 1, targ); return ctTarg(list + 1, len - 1, targ); } int main() { string pets[] = {"cat", "dog", "mouse", "cat"}; cout << ctTarg(pets, 4, "cat") << endl; }arrow_forward
- You may operate on a stack WORK and a temporary stack TEMP (if necessary) to enable its ADT operations of PUSH (S,X), POP (S, X), and EMPTYSTACK (S), where X represents an element/variable to be pushed into or popped out of the stack and S represents a stack. You may also utilise one variable to carry out the procedures if necessary. I Given n different random integers to be pushed into WORK, how can you determine the maximum element pushed into it while ensuring that the items placed in WORK are in decreasing order, with the maximum element beginning at the bottom of the stack? You may utilise a single variable and the temporary stack TEMP. ii) Given an array A[1: n] of different random numbers, how can you extract the array's sorted list using only stacks?arrow_forwardWhereas the unbounded stack has no such limits, could you perhaps elaborate on how this works?arrow_forwardDiscover the stack's performance when let to be itself.arrow_forward
- Stack A has the entries a, b, c (with a on top). Stack B is empty. An entry popped out of stack A can be printed immediately or pushed to stack B. An entry popped out of the stack B can only be printed. In this arrangement, which of the following permutations of a, b, c are not possible?arrow_forwardDiscover the stack's performance when given the freedom to be who it is.arrow_forwardC4arrow_forward
- Solve the code much needed. Given a stack, a function is_consecutive takes a stack as a parameter and thatreturns whether or not the stack contains a sequence of consecutive integersstarting from the bottom of the stack (returning true if it does, returningfalse if it does not). For example:bottom [3, 4, 5, 6, 7] topThen the call of is_consecutive(s) should return true.bottom [3, 4, 6, 7] topThen the call of is_consecutive(s) should return false.bottom [3, 2, 1] topThe function should return false due to reverse order. Note: There are 2 solutions:first_is_consecutive: it uses a single stack as auxiliary storagesecond_is_consecutive: it uses a single queue as auxiliary storage"""import collections def first_is_consecutive(stack): storage_stack = [] for i in range(len(stack)): first_value = stack.pop() if len(stack) == 0: # Case odd number of values in stack return True second_value = stack.pop() if first_value - second_value != 1: # Not…arrow_forwardCould you help explain the difference between the restricted version of the stack and the unbounded version of the stack? Thank you for your time.arrow_forwardThe following function is supposed to print the last Kelements of a linked list in reverse order using stack. The function code is incomplete. You are expected to fill in (1), (2), and (3) with a single line of code for each. 1- void function(Node* head, int k) { Node *cur = head; if (cur 2 3 NULL) 4 return; 5 6 Stack st; 7 8 - while (cur != NULL) { 9 //fill in here (1) 10 //fill in here (2) 11 } 12 13 int counter 0; %3D 14 while (counter < k) { cout <« st.top() < //fill in here (3) counter++; } 15 - 16 17 18 19 20 } Your answerarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
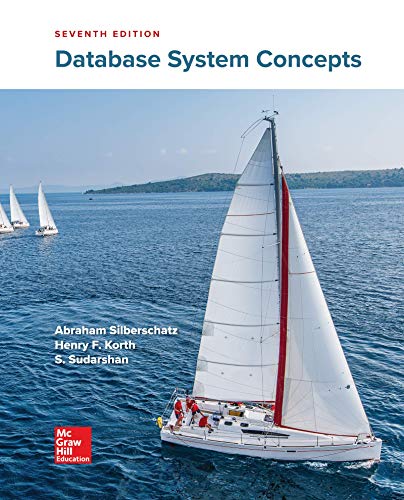
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
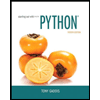
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
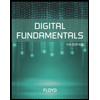
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
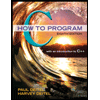
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
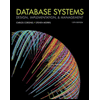
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
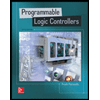
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education