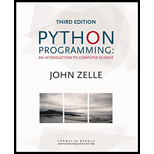
Draw a face
Program plan:
- Import the header file.
- Define the “drawFace” method
- Get the “x” and “y” positions
- Get the “p1” and “p2” positions
- Call the “Oval” method for drawing the face
- Set the color to the face
- Draw the face
- Get the “lc” and “rc” position
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Get the “m1”, “m2”, and “m3” values
- Call the “Rectangle” method
- Set the color to the face
- Call the “draw” method
- Call the “Line” method
- Get the center position
- Get the “x” and “y” position
- Call the “Point” method
- Call the “Line” method
- Call the “draw” method
- Get the “x” and “y” position for different variables
- Calculate “t” and “t1” value
- Call the “Rectangle” method
- Call the “draw” method
- Iterate “i” until it reaches 7
- Clone the teeth by calling “clone” method
- Move the face by calling “move” method
- Call the “draw” method
- Define the main method.
- Read the filename from the user
- Get the image width and height from the file
- Set the graph win size
- Set the coords for graph
- Draw the face
- Get the number of faces from the user
- Iterate “i” until it reaches “n” value
- Get the mouse action
- Call the “drawFace” method
- Call the function “main()”.

This Python program is used to get the GIF (Graphic Interchange format) file and draw the smileys when the user clicking the GIF image.
Explanation of Solution
Program:
#import the header file
from graphics import *
#definition of "drawFace" method
def drawFace(center, size, window):
#get the "x" value
x1 = center.getX()
#get the "y" value
y1 = center.getY()
#get the "p1" value
p1 = Point(x1-(.7 * size), y1 - size)
#get the "p2" value
p2 = Point(x1+(.7 * size), y1 + size)
#call the "Oval" method
head = Oval(p1, p2)
#fill the color
head.setFill("white")
#call the "draw" method
head.draw(window)
#get the "lc" value
lc = Point(x1 - .2 * size, y1 + .6 * size)
#get the "rc" value
rc = Point(x1 + .2 * size, y1 + .6 * size)
#call the "Circle" method
leftEye = Circle(lc, .13 * size)
#fill the color
leftEye.setFill("white")
#call the "draw" method
leftEye.draw(window)
#call the "Circle" method
rightEye = Circle(rc, .13 * size)
#fill the color
rightEye.setFill("white")
#call the "draw" method
rightEye.draw(window)
#get the "m1" value
m1 = Point(x1 - .3 * size, y1 - .5 * size)
#get the "m2" value
m2 = Point(x1 + .3 * size, y1 - .25 * size)
#get the "m3" value
m3 = Point(x1 + .3 * size, y1 - .5 * size)
#call the "Rectangle" method
mouth = Rectangle(m1, m2)
#fill the color
mouth.setFill("white")
#call the "draw" method
mouth.draw(window)
#call the "Line" method
leftLip = Line(m1, Point(x1- .3 * size, y1 - .25 * size))
#get the center position
mLeftCent = leftLip.getCenter()
#get the "x" position
mLCx = mLeftCent.getX()
#get the "y" position
mLCy = mLeftCent.getY()
#call the "Point" method
mRightCent = Point(x1 + .3 * size, mLCy)
#call the "Line" method
lip = Line(mLeftCent, mRightCent)
#call the "draw" method
lip.draw(window)
#get the "x" positions
m1x = m1.getX()
m3x = m3.getX()
#get the "y" positions
m1y = m1.getY()
m2y = m2.getY()
#calculate the "t" value
t = m1x - m3x
#calculate the "t1" value
t1 = Point(m1x - (1/8 * t), m2y)
#call the "Rectangle" method
teeth = Rectangle(m1, t1)
#call the "draw" method
teeth.draw(window)
#iterate "i" until it reaches 7
for i in range (7):
#clone the face
t2 = teeth.clone()
#call the "move" method
t2.move(-i * (1/8 * t), 0)
#call the "draw" method
t2.draw(window)
#definition of main method
def main():
#get the input filename from the user
fname = input("Enter filename: ")
infile = Image(Point(10, 10), fname)
#get the width of the image
wWidth = infile.getWidth()
#get the height of the image
wHeight = infile.getHeight()
#set graph win name
window = GraphWin('Smile!', wWidth,wHeight)
#set the coords
window.setCoords(0, 0, 20, 20)
#draw a smiley
infile.draw(window)
#get how many faces the user wants to draw
n = eval(input("How many faces should we block? "))
#iterate "i" until it reaches "n"
for i in range(n):
#get the mouse action
point = window.getMouse()
#draw a face by calling the method "drawFace"
drawFace(point, 3, window)
#call the "main" method
main()
Output:
Enter filename: test_img.gif
How many faces should we block? 2
>>>
Screenshot of “Smile!” window
Want to see more full solutions like this?
Chapter 6 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- Inheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forward
- Please answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forwardI need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forward
- Why is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forward
- a. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forwardUsing XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forwardQ4) A thin ring of radius 5 cm is placed on plane z = 1 cm so that its center is at (0,0,1 cm). If the ring carries 50 mA along a^, find H at (0,0,a).arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
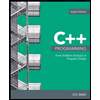
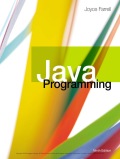
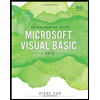
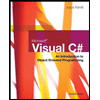
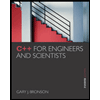