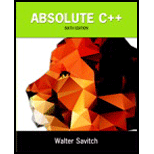
Write a function called deleteRepeats that has a partially filled array of characters as a formal parameter and that deletes all repeated letters from the array. Since a partially filled array requires two arguments, the function will actually have two formal parameters: an array parameter and a formal parameter of type int that gives the number of array positions used. When a letter is deleted, the remaining letters are moved forward to fill in the gap. This will create empty positions at the end of the array so that less of the array is used. Since the formal parameter is a partially filled array, a second formal parameter of type int will tell how many array positions are filled. This second formal parameter will be a call-by-reference parameter and will be changed to show how much of the array is used after the repeated letters are deleted. For example, consider the following code:
After this code is executed, the value of a [0] is 'a', the value of a [1 ] is 'b', the value of a [2] is 'c', and the value of size is 3. (The value of a [3] is no longer of any concern, since the partially filled array no longer uses this indexed variable.) You may assume that the partially filled array contains only lowercase letters. Embed your function in a suitable test

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Survey of Operating Systems, 5e
Starting out with Visual C# (4th Edition)
Data Mining for Business Analytics: Concepts, Techniques, and Applications with XLMiner
Experiencing MIS
Java: An Introduction to Problem Solving and Programming (7th Edition)
Artificial Intelligence: A Modern Approach
- Write a function template that gets an array of generic type T as well as the number of items within the array, calculates the standard deviation of the items within the array and returns it. The user will first give an integerItemCount value (int) and that many integer values (all ints). Then, the user will give a doubleItemCount value (int) and that many double values (all doubles). Then, the program will use the function template to calculate the standard deviations of both arrays and print them out as first the standard deviation of the int array, then the standard deviation of the double array. Standard deviation of an array with size N, values are given as , and arithmetic mean as μ can be calculated as: Requirements: You MUST define and use a function template that takes an array of generic type T and an integer which defines the size of the array Getting all the user input, calling the appropriate version of the function via the template and printing the output should be done…arrow_forwardWrite a function reportDuplicates() that takes a two di mensional integer array as a parameter and identifies the duplicate valuesin the array. The function then reports these to user. Sample input and corresponding output:How many rows? 5How many columns? 2Let’s populate the array:1 86 97 312 522 4Thank you, there are no duplicate elements!How many rows? 3How many columns? 4Let’s populate the array:3 7 5 76 9 7 38 5 12 6Thank you, 3 appears 2 times, 7 appears 3 times, 5 appears 2 times,and 6 appears 2 times.arrow_forwardWrite a function removeDuplicates -PHP( arr) that takes an associative array as a parameter and returns the same associative array except with all duplicate values removed. You may not use the function array_unique for this problem. For example:$arr = array( 'a' => "one", 'b' => "two", 'c' => "three", 'd' => "two", 'e' => "four", 'f' => "five", 'g' => "three", 'h' => "two" ); Expand (10 lines) print_r(removeDuplicates( arr)); Would print: Array ( [a] => one [e] => four [f] => five )arrow_forward
- Write a function averageCatAge() that takes an array of cat structs (cat* a_cats) and the size of the array (unsigned int numCats) as input parameters. The function then returns the mean or average age for all the cats...the average is ..... the sum of values divided by the number of valueS just in case you forgot. The average returned is a double.arrow_forwardCreate a program that accepts an integer N, and pass it to the function generatePattern. generatePattern() function which has the following description: Return type - void Parameter - integer n This function prints a right triangular pattern of letter 'T' based on the value of n. The top of the triangle starts with 1 and increments by one down on the next line until the integer n. For each row of in printing the right triangle, print "T" for n times. In the main function, call the generatePattern() function. Input 1. One line containing an integer Output Enter N: 4 T TT TTT TTTTarrow_forward1. Write an Arduino program that globally declares an array of size fifteen. The array data type is int. Write a function that fills the array with random values in the range [low, high]. The function has two parameters: low, high. The array is not a function parameter because it has been declared globally. Write a function that serially tranmits the array data. The function has one parameter that controls the number of array elements printed on the same line. For example, assume the function is called printArray. If we want 3 array elements to be printed on one line, then the function call is printArray(3). We would see something like this for output: Array -3 14 -3 2 11 -9 7 10 -4 -2 8 See how there are 3 values printed per line? 3 is the value passed to the function parameter that controls the number of array elements printed on one line. There should be at least one space between array elements printed. In the loop function, call the function that fills the array with random values…arrow_forward
- Nothing too advanced pleaseC++arrow_forwardDefine a function named “getExamListInfo” that accepts an array of Exam objects and its size. It will return the following information to the caller: - The number of exams with perfect score - The number of exams with “Pass” status - The index of the Exam object in the array that has the largest score. For example, if you get the exam info for this array of Exam object Exam examList[] = { {"Midterm1 Exam", 90}, {"Midterm2 Exam", 80}, {"Final Exam", 50}, {"Extra Credit", 100}, {"Initial Test", 0}, {"Homework1", 69} } ; You will get Perfect Count: 1 Pass Count: 3 Index of the largest: 3 C++arrow_forwardWrite a modular program that analyzes a year’s worth of rainfall data. In addition to main, the program should have a getData function that accepts the total rainfall for each of 12 months from the user and stores it in an array holding double numbers. It should also have four value-returning functions that compute and return to main the totalRainfall, averageRainfall, driestMonth, and wettestMonth. These last two functions return the number of the month with the lowest and highest rainfall amounts, not the amount of rain that fell those months. Notice that this month number can be used to obtain the amount of rain that fell those months. This information should be used either by main or by a displayReport function called by main to print a summary rainfall report similar to the following: 2019 Rain Report for Springdale County Total rainfall: 23.19 inches Average monthly rainfall: 1.93 inches The least rain fell in January with 0.24 inches. The most rain fell in April with 4.29…arrow_forward
- Write a function called GradientCount that accepts as parameter a two-dimensional array called Matrix of size 6 by 6. The function returns how many numbers in the Matrix are gradient numbers. A number in the Matrix is considered a gradient number if it matches the following two rules: 1. The numbers to the left and above the number are smaller. 2. The numbers to the right and below the number are larger.arrow_forwardSuppose you are given a list of students registered in a course and you are required to implement an array-based student list. Each student in the list has name, regNo, department and cGpa. Implement different functions for entering the data (like constructor (for setting to default values), setName, setReg, etc.). You also need to implement the following function related to the array. insertStudent( ) : It has 2 options • Asking the user for index and then insert the student at that index AND • Asking the user for name of the student where the new student is to be added (New student should be added at the index of the student whose name is entered) o If the list is full, it should create a larger list (new size should be entered by the user), copy all the data in it and make insertion as instructed o (Insertion will only be made if the reg of the new student doesn’t exist in the list) deleteStudent( ): Same as insertStudent( ) (either by asking the index or student name) sort( ): Ask…arrow_forwardWrite a function called modifyDistinct that takes an array of positive integers in random order and modifies all distinct integers in the array. The function should take three arguments: (1) an array of integers; (2) an integer that tells the size of the array. (3) an integer to use for replacing distinct values. The function should not return a value but should change the values of distinct integers to be the value passed in the third argument. Here is an example. Suppose the array passed to the function is as shown below, and the integer passed as array size is 11 and the integer used for replacing distinct values is -1. 1 2 3 4 5 6 7 8 9 10 Array Before: 58 | 26 | 91 | 26 | 70 | 70 | 91 | 58 | 21 | 58 | 66 Then the function should alter the array so that it looks like this: 0 1 2 3 4 5 6 7 8 9 10 Array After: 58 | 26 | 91 | 26 | 70 | 70| 91| 58 | -1 | 58 | -1 The -1 in the cells indicate that the cell contained a distinct integer that is replaced with -1 (the value of the third…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage