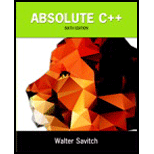
Write a
There are a variety of ways to deal with the month names. One straightforward method is to code the months as integers and then do a conversion before doing the output. A large switch statement is acceptable in an output function. The month input can be handled in any manner you wish, as long as it is relatively easy and pleasant for the user.
After you have completed the previous program, produce an enhanced version that also outputs a graph showing the average rainfall and the actual rainfall for each of the previous 12 months. The graph should be similar to the one shown in Display 5.4, except that there should be two bar graphs for each month, and they should be labeled as the average rainfall and the rainfall for the most recent month. Your program should ask the user whether he or she wants to see the table or the bar graph, and then should display whichever format is requested. Include a loop that allows the user to see either format as often as the user wishes until the user requests that the program end.

Program plan:
- Necessary header files are included.
- Four functions table (),barGraph(),barAsterisks(),displayMonth () are declared for various functions and defined inside the main() function
- double actualRainfall[12],double avgRainfall[12], double statusRainfall[12],int currentMonth, char userChoice, int count variables are declared of different data types to store multiple values required in the program.
- For loops are used for various conditions.
- A do-while loop is used to exit from the program.
- A switch statement is used for printing the different month names according the choice of month.
Program Description:
The main purpose of the program is to compare the rainfall of 12 months in a city. Comparison of average monthly rainfall and actual monthly rainfall is shown either in tabular form or with the help of Bar Graph.
Explanation of Solution
Sample Program
/*Header files section*/ #include<iostream> #include<stdlib.h> #include<string> #include <iomanip> using namespace std; //Function declaration void table(double actualRainfall[], double avgRainfall[], double statusRainfall[]); void barGraph(double avgRainfall[], double actualRainfall[], double statusRainfall[]); void barAsterisks(int stars); void displayMonth(int month); //main method int main() { //Declare variables double actualRainfall[12]; double avgRainfall[12]; double statusRainfall[12]; int currentMonth; char userChoice; int count=0; //Prompt and read each month's rainfall //from the user cout << "Enter average monthly " << "rainfalls for each month..." << endl; for (int i = 0; i < 12; i++) { displayMonth(i); cout << ": "; cin >> avgRainfall[i]; } //Prompt and read the current month // and then asks for the rainfall figures //for the previous 12 months cout << "Enter the current month " "(For example: Enter 1 for January) : " << endl; cin >> currentMonth; cout << "Enter the actual rainfall for " " each month in the previous year..." << endl; for (int month = currentMonth - 1; count < 12; month = (month + 1) % 12, count++) { displayMonth(month); cout << ": "; cin >> actualRainfall[month]; } for (int i = 0, j = 0; i < 12; i++, j++) { if (avgRainfall[i] > actualRainfall[i]) statusRainfall[j] = actualRainfall[i] - avgRainfall[i]; else statusRainfall[j] = actualRainfall[i] - avgRainfall[i]; } cout << endl; //do-while loop to see either table or bar do { cout << "Enter your choice(T for table or " " B for bar-graph or E for exit program): "; cin >> userChoice; cout << endl; if (userChoice == 'T' || userChoice == 't') table(actualRainfall, avgRainfall, statusRainfall); else if (userChoice == 'B' || userChoice == 'b') barGraph(avgRainfall, actualRainfall, statusRainfall); else if (userChoice == 'E' || userChoice == 'e') { cout << "Exit the program...Thank you"<<endl; exit(0); } cout << endl; } while (true); return 0; } //Method definition of table void table(double rainfall[], double avgRainfall[], double statusRainfall[]) { cout << endl; cout << "___________________________________________" "______________________________________" << endl; cout << "Month" << setw(20) << "Actual_Rainfall" << setw(20) << "Average_Rainfall "<< setw(25) << " Above_or_Below_Average_Rainfall" << setw(20) << endl; cout << "___________________________________________" "______________________________________" << endl; for (int i = 0; i < 12; i++) { cout.setf(ios_base::left); displayMonth(i); cout << "\t" << setw(20) << rainfall[i] << setw(20) << avgRainfall[i] << setw(20) << statusRainfall[i]; cout << endl; } cout << "_____________________________________________" "____________________________________" << endl; } //Method definition of barGraph void barGraph(double avgRainfall[], double rainfall[], double statusRainfall[]) { cout << "Graph showing the average rainfall " "and the actual rainfall for each of the " "previous 12 months..." << endl; cout << endl; for (int i = 0; i < 12; i++) { displayMonth(i); cout << ":" << "Average_Rainfall" << " "; int n1 = (int)avgRainfall[i]; barAsterisks(n1); cout << endl; displayMonth(i); cout << ":" << "Actual_Rainfall" << " "; int n = (int)rainfall[i]; barAsterisks(n); cout << endl; cout << endl; } } //Method definition of barAsterisks void barAsterisks(int stars) { for (int count = 1; count <= stars; count++) cout << "*"; } //Method definition of displayMonth void displayMonth(int month) { cout.width(8); switch (month) { case 0: cout << "January"; break; case 1: cout << "February"; break; case 2: cout << "March"; break; case 3: cout << "April"; break; case 4: cout << "May"; break; case 5: cout << "June"; break; case 6: cout << "July"; break; case 7: cout << "August"; break; case 8: cout << "September"; break; case 9: cout << "October"; break; case 10: cout << "November"; break; case 11: cout << "December"; break; } }
Explanation:
In the above program, user inputs the average and actual monthly rainfall for the 12 months. After that a comparison is made between the average and actual rainfall of the particular month. Different options are provided to the user to display the comparison of rainfalls of the months either in Tabular form or as Bar Graph. In that option only, user can choose to exit the program by choosing the provided option. In the last according to the choice of user, data is displayed either in Tabular form or as Bar Graph.
Sample Output:
Want to see more full solutions like this?
Chapter 5 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Problem Solving with C++ (10th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Starting Out with Python (4th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with C++: Early Objects (9th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- Which one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forwardInheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forwardSuppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forward
- Which one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardPlease answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forwardI need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forward
- Southern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forwardWhy is JAVA OOP is really difficult to study?arrow_forwardMy daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forward
- Q4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forwarda. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forwardUsing XML, design a simple user interface for a fictional app. Your UI should include at least three different UI components (e.g., TextView, Button, EditText). Explain the purpose of each component in your design-you need to add screenshots of your work with your name as part of the code to appear on the interface-. Screenshot is needed.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
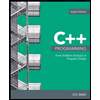
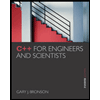
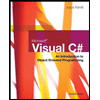