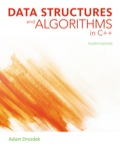
Interpreter:
- Interpreter executes each statement of a program at a time.
- It calls recursive functions for “statement”, “expression”, “factor”, “term” and “Identifier” to execute a statement.
- It allows arithmetic operations such as addition, subtraction, multiplication and division.

/**********************************************************
* This program extends the interpreter with integer *
* division. *
**********************************************************/
Explanation of Solution
//interpreter.h
//Include header files
#ifndef INTERPRETER
#define INTERPRETER
#include <iostream>
#include <list>
#include <algorithm> // find
using namespace std;
//Definition of class IdNode
class IdNode
{
//Declare public methods
public:
//Declare Parameterized constructor
IdNode(char *s = "", double e = 0)
{
//Initialize variables
id = strdup(s);
value = e;
}
//Function to overload operator ==
bool operator== (const IdNode& node) const
{
/*Return true if both string are same, otherwise return false*/
return strcmp(id,node.id)==0;
}
//Declare private variables and overloading function
private:
//Declare variables
char *id;
double value;
//Declare class object
friend class Statement;
//Prototype for overloading function
friend ostream& operator<<(ostream&, const IdNode&);
};
//Definition of class Statement
class Statement
{
//Declare public functions
public :
//Constructor
Statement(){}
//Declare function getStatement()
void getStatement();
//Declare private variables
private:
//Declare variables
list<IdNode>idList;
char ch;
//Function prototypes
double factor();
double term();
double expression();
int fl1,fl2,fl3;
void readId(char*);
//Declare function to print error message
void issueError(char* s)
{
cerr << s << endl; exit(1);
}
//Function prototypes
double findValue(char*);
void processNode(char*, double);
friend ostream& operator<< (ostream&, const Statement&);
};
#endif
//interpreter.cpp
//Include header files
#include <cctype>
#include <stdio.h>
#include <string.h>
#include <string>
#include <list>
#include "interpreter.h"
//Function to find value of identifier
double Statement::findValue(char *id)
{
//Create object of class IdNode for id
IdNode tmp(id);
/*If identifier is present in IdNode list, return position */
list<IdNode>::iterator i = find(idList.begin(),idList.end(),tmp);
//If position is not end of list
if (i != idList.end())
//Return value of identifier
return i->value;
//Otherwise
else
//Print error message
issueError("Unknown variable");
// This statement will never be reached;
return 0;
}
//Function to process node
void Statement::processNode(char* id,double e)
{
/*Create object of class for IdNode for statement*/
IdNode tmp(id,e);
/*If identifier is present in IdNode list find position of identifier*/
list<IdNode>::iterator i = find(idList.begin(),idList.end(),tmp);
//If position of identifier is not end of IdNode list
if (i != idList.end())
//Set value of identifier as value of expression
i->value = e;
//Otherwise
else
//Insert New value into list
idList.push_front(tmp);
}
/* readId() reads strings of letters and digits that start with a letter, and stores them in array passed to it as an actual parameter.*/
/* Examples of identifiers are: var1, x, pqr123xyz, aName, etc.*/
//Function to read identifier
void Statement::readId(char *id)
{
//Initialize variable i as 0
int i = 0;
//If ch is space
if (isspace(ch))
//Skip balks and read next character
cin >> ch;
//If character is alphabet
if (isalpha(ch))
{
//Read character till read a non-alphanumeric
while (isalnum(ch))
{
//Copy each character to identifier
id[i++] = ch;
//Read next character
cin.get(ch);
}
//Set last character as end of string
id[i] = '\0';
}
//Otherwise
else
//Print error message
issueError("Identifier expected");
}
//Function factor()
double Statement::factor()
{
//Declare variables
double var, minus = 1.0;
static char id[200];
fl3=0;
//Read character
cin >> ch;
//If ch is operator + or -
while (ch == '+' || ch == '-')
{
// take all '+'s and '-'s.
//If ch is -
if (ch == '-')
/*Set value as negative by multiplying with -1*/
minus *= -1.0;
//Read next character
cin >> ch;
}
//If ch is digit or operator dot
if (isdigit(ch) || ch == '.')
{
// Factor can be a number
//Read digits of number
cin.putback(ch);
//Append digits to variable to form number
cin >> var >> ch;
}
//If ch is paranthesis (
else if (ch == '(')
{
//Get next expression after (
var = expression();
//If character is )
if (ch == ')')
//Read next character
cin >> ch;
//Otherwise
else
//Print error message
issueError("Right paren left out");
}
//Otherwise
else
{
//Read identifier
readId(id);
//If chracter is space
if (isspace(ch))
//Read next character
cin >> ch;
//Get value of identifier
var = findValue(id);
}
//If value of var is an integer
if (var-(int)var == 0)
{
//Set flag fl3 as 1
fl3=1;
}
//Return value
return minus * var;
}
//Function for term()
double Statement::term()
{
//Call function factor()
double f= factor();
//Do calculation for operators * and /
while (true)
{
//Switch statement
switch (ch)
{
//If ch is *, multiply recursively
case '*' : f *= factor(); break;
//If ch is /
case '/' :
//If fl3 equals 1
if (fl3 == 1)
{
//Set fl1 as 1
fl1=1;
}
//If fl3 equals 0
else
//Set fl1 as 0
fl1=0;
//Divide recursively
f /= factor();
//If fl3 equals 1
if (fl3 == 1)
{
//Set fl2 as 1
fl2=1;
}
//If fl3 equals 0
else
//Set fl2 as 0
fl2=0;
//If both fl1 and fl2 are 1
if(fl1==1 && fl2==1)
{
//Take integer part as result
f=(double)((int)f);
}
//Otherwise
else
{
/*Take double value itself as result*/
f=f;
}
break;
//Default
default:
//Return result
return f;
}
}
}
//Function for expression()
double Statement::expression()
{
//Call function term()
double t = term();
//Do calculation for operators + and -
while (true)
{
//Switch statement
switch (ch)
{
//If ch is +, adds numbers recursively
case '+' : t += term(); break;
//If ch is -, subtract numbers recursively
case '-' : t -= term(); break;
// Return f
default : return t;
}
}
}
//Function to read statement
void Statement::getStatement()
{
//Declare variables
char id[20], command[20];
double e;
//Prompt and read statement
cout << "Enter a statement: ";
cin >> ch;
//Read identifier
readId(id);
//Copy identifier to string command
strupr(strcpy(command,id));
//If command is STATUS
if (strcmp(command,"STATUS") == 0)
//Print current values of all variables
cout << *this;
//If command is PRINT
else if (strcmp(command,"PRINT") == 0)
{
//Read identifier from user
readId(id);
//Get value of identifier and print it
cout << id << " = " << findValue(id) << endl;
}
//If command is END
else if (strcmp(command,"END") == 0)
//Retun from program
exit(0);
//Otherwise
else
{
//If ch is space
if (isspace(ch))
//Read next character
cin >> ch;
//If ch is =
if (ch == '=')
{
//Read expression
e = expression();
//If ch is not ;
if (ch != ';')
//print error message
issueError("There are some extras in the statement");
//Otherwise process statement
else processNode(id,e);
}
//Otherwise print error message
else issueError("'=' is missing");
}
}
/*Function to overload operator << to print values all identifiers in list*/
ostream& operator<< (ostream& out, const Statement& s)
{
//Initialize iterator
list<IdNode>::const_iterator i = s.idList.begin();
//For each identifier
for ( ; i != s.idList.end(); i++)
//Print value of identifier
out << *i;
//Print new line
out << endl;
return out;
}
/*Function to overload operator << to print value of identifier*/
ostream& operator<< (ostream& out, const IdNode& r)
{
//Print value of identifier
out << r.id << " = " << r.value << endl;
return out;
}
//useInterpreter.cpp
//Include header files
#include "interpreter.h"
using namespace std;
//Program begins with main()
int main()
{
//Declare object of class Statement
Statement statement;
//Prompt message
cout << "The program processes statements of the following format:\n"<< "\t<id> = <expr>;\n\tprint <id>\n\tstatus\n\tend\n\n";
// This infinite loop is broken by exit(1)
while (true)
// in getStatement() or upon finding an
statement.getStatement();
//Return 0
return 0;
}
Explanation:
- Declare integer type flag variables “fla1”, “fla2” and “fla3” in class “Statement”.
- In function “factor()”,
- Initialize “fla3” as “0”,
- If number is integer type set “fla3” as “1”
- In Function “term()”
- If “ch” is “/” operator and “fla3” is “1”, set “fla1” as “1”.
- If “fla3” is “1” after recursive call of function “factor()” (That is after division), set “fla2” as “1”.
- If both flags “fla1” and “fla2” are “1” (That is both are integers for division), remove floating part of result.
Output:
The program processes statements of the following format:
<id> = <expr>;
print <id>
status
end
Enter a statement: var1=2+(11/2);
Enter a statement: var2=var1+(15.5/3.7);
Enter a statement: var3=var2*(8.2/2);
Enter a statement: status
var3 = 45.8757
var2 = 11.1892
var1 = 7
Enter a statement: var4=var3+(8/1.1);
Enter a statement: print var4
var4 = 53.1484
Enter a statement: end
Want to see more full solutions like this?
Chapter 5 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- Consider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forwardConsider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forward
- What is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forward
- No aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forwardWhat are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward
- 2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer the questions below, 1. Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key 2. Is there a direct relationship that exists between Store and Customer entities? AnswerYes/No?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
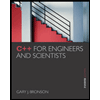
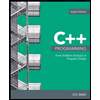
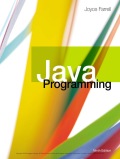
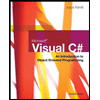
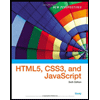