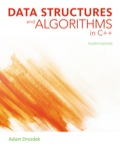
Interpreter:
- Interpreter executes each statement of a program at a time.
- It calls recursive functions for “statement”, “expression”, “factor”, “term” and “Identifier” to execute a statement.
- It allows arithmetic operations addition, subtraction, multiplication, division and exponentiation.

/***********************************************************
* This program extends the interpreter with exponentiation *
* process. *
***********************************************************/
Explanation of Solution
//interpreter.h
//Include header files
#ifndef INTERPRETER
#define INTERPRETER
#include <iostream>
#include <list>
#include <algorithm> // find
using namespace std;
//Definition of class IdNode
class IdNode
{
//Declare public methods
public:
//Declare Parameterized constructor
IdNode(char *s = "", double e = 0)
{
//Initialize variables
id = strdup(s);
value = e;
}
//Function to overload operator ==
bool operator== (const IdNode& node) const
{
/*Return true if both string are same, otherwise return false*/
return strcmp(id,node.id)==0;
}
//Declare private variables and overloading function
private:
//Declare variables
char *id;
double value;
//Declare class object
friend class Statement;
//Prototype for overloading function
friend ostream& operator<<(ostream&, const IdNode&);
};
//Definition of class Statement
class Statement
{
//Declare public functions
public :
//Constructor
Statement(){}
//Declare function getStatement()
void getStatement();
//Declare private variables
private:
//Declare variables
list<IdNode>idList;
char ch;
//Function prototypes
double factor();
double term();
double expression();
double exponent();
void readId(char*);
//Declare function to print error message
void issueError(char* s)
{
cerr << s << endl; exit(1);
}
//Function prototypes
double findValue(char*);
void processNode(char*, double);
friend ostream& operator<< (ostream&, const Statement&);
};
#endif
//interpreter.cpp
//Include header files
#include <cctype>
#include <stdio.h>
#include <string.h>
#include <string>
#include <list>
#include "interpreter.h"
//Function to find value of identifier
double Statement::findValue(char *id)
{
//Create object of class IdNode for id
IdNode tmp(id);
/*If identifier is present in IdNode list, return position */
list<IdNode>::iterator i = find(idList.begin(),idList.end(),tmp);
//If position is not end of list
if (i != idList.end())
//Return value of identifier
return i->value;
//Otherwise
else
//Print error message
issueError("Unknown variable");
// This statement will never be reached;
return 0;
}
//Function to process node
void Statement::processNode(char* id,double e)
{
/*Create object of class for IdNode for statement*/
IdNode tmp(id,e);
//If identifier is present in IdNode list find position of identifier
list<IdNode>::iterator i = find(idList.begin(),idList.end(),tmp);
//If position of identifier is not end of IdNode list
if (i != idList.end())
//Set value of identifier as value of expression
i->value = e;
//Otherwise
else
//Insert New value into list
idList.push_front(tmp);
}
/* readId() reads strings of letters and digits that start with a letter, and stores them in array passed to it as an actual parameter.*/
/* Examples of identifiers are: var1, x, pqr123xyz, aName, etc.*/
//Function to read identifier
void Statement::readId(char *id)
{
//Initialize variable i as 0
int i = 0;
//If ch is space
if (isspace(ch))
//Skip balks and read next character
cin >> ch;
//If character is alphabet
if (isalpha(ch))
{
//Read character till read a non-alphanumeric
while (isalnum(ch))
{
//Copy each character to identifier
id[i++] = ch;
//Read next character
cin.get(ch);
}
//Set last character as end of string
id[i] = '\0';
}
//Otherwise
else
//Print error message
issueError("Identifier expected");
}
//Function to find exponent
double Statement::exponent()
{
// <exponent> ::= <factor> ^ <exponent>,
double f = factor();
//If ch is operatotr ^
if (ch == '^')
//Compute power and Return it
return pow(f,exponent());
//Otherwise
else
//Return f
return f;
}
//Function factor()
double Statement::factor()
{
//Declare variables
double var, minus = 1.0;
static char id[200];
//Read character
cin >> ch;
//If ch is operator + or -
while (ch == '+' || ch == '-')
{
// take all '+'s and '-'s.
//If ch is -
if (ch == '-')
/*Set value as negative by multiplying with -1*/
minus *= -1.0;
//Read next character
cin >> ch;
}
//If ch is digit or operator dot
if (isdigit(ch) || ch == '.')
{ // Factor can be a number
//Read digits of number
cin.putback(ch);
cin >> var >> ch;
}
//If ch is paranthesis (
else if (ch == '(')
{
// or a parenthesized expression,
//Get next expression after (
var = expression();
//If character is )
if (ch == ')')
//Read next character
cin >> ch;
//Otherwise
else
//Print error message
issueError("Right paren left out");
}
//Otherwise
else
{
//Read identifier
readId(id);
//If chracter is space
if (isspace(ch))
//Read next character
cin >> ch;
//Get value of identifier
var = findValue(id);
}
//Return value
return minus * var;
}
//Function for term()
double Statement::term()
{
//Call function exponent()
double f = exponent();
//Do calculation for operators * and /
while (true)
{
//Switch statement
switch (ch)
{
//If ch is *, multiply recursively
case '*' : f *= exponent(); break;
//If ch is *, divide recursively
case '/' : f /= exponent(); break;
//Defaultly return f
default : return f;
}
}
}
//Function for expression()
double Statement::expression()
{
//Call function term()
double t = term();
//Do calculation for operators + and -
while (true)
{
//Switch statement
switch (ch)
{
//If ch is +, add recursively
case '+' : t += term(); break;
//If ch is -, subtract recursively
case '-' : t -= term(); break;
// Return f
default : return t;
}
}
}
//Function to read statement
void Statement::getStatement()
{
//Declare variables
char id[20], command[20];
double e;
//Prompt and read statement
cout << "Enter a statement: ";
cin >> ch;
//Read identifier
readId(id);
//Copy identifier to string command
strupr(strcpy(command,id));
//If command is STATUS
if (strcmp(command,"STATUS") == 0)
//Print current values of all variables
cout << *this;
//If command is PRINT
else if (strcmp(command,"PRINT") == 0)
{
//Read identifier from user
readId(id);
//Get value of identifier and print it
cout << id << " = " << findValue(id) << endl;
}
//If command is END
else if (strcmp(command,"END") == 0)
//Retun from program
exit(0);
//Otherwise
else
{
//If ch is space
if (isspace(ch))
//Read next character
cin >> ch;
//If ch is =
if (ch == '=')
{
//Read expression
e = expression();
//If ch is not ;
if (ch != ';')
//print error message
issueError("There are some extras in the statement");
//Otherwise process statement
else processNode(id,e);
}
//Otherwise print error message
else issueError("'=' is missing");
}
}
/*Function to overload operator << to print values all identifiers in list*/
ostream& operator<< (ostream& out, const Statement& s)
{
//Initialize iterator
list<IdNode>::const_iterator i = s.idList.begin();
//For each identifier
for ( ; i != s.idList.end(); i++)
//Print value of identifier
out << *i;
//Print new line
out << endl;
return out;
}
/*Function to overload operator << to print value od identifier*/
ostream& operator<< (ostream& out, const IdNode& r)
{
//Print value of identifier
out << r.id << " = " << r.value << endl;
return out;
}
//useInterpreter.cpp
//Include header files
#include "interpreter.h"
using namespace std;
//Program begins with main()
int main()
{
//Declare object of class Statement
Statement statement;
//Prompt message
cout << "The program processes statements of the following format:\n"<< "\t<id> = <expr>;\n\tprint <id>\n\tstatus\n\tend\n\n";
// This infinite loop is broken by exit(1)
while (true)
// in getStatement() or upon finding an
statement.getStatement();
//Return 0
return 0;
}
Explanation:
- Define function “exponent()” as member of class “Statement”.
- In function “exponent()”,
- It calls function “factor()”
- If next character is “^” compute exponentiation by calling function itself recursively.
- Return result.
- Function “term()” calls function “exponent()” instead of “factor()”.
Output:
The program processes statements of the following format:
<id> = <expr>;
print <id>
status
end
Enter a statement: var1=2+(2^3);
Enter a statement: var2=var1-2;
Enter a statement: var3=var2*2;
Enter a statement: status
var3 = 16
var2 = 8
var1 = 10
Enter a statement: var3=var3-4;
Enter a statement: print var3
var3 = 12
Enter a statement: end
Want to see more full solutions like this?
Chapter 5 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- 1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forward
- What are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forward
- We want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forwardA controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward
- 4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forwardWhich tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
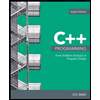
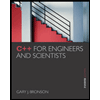
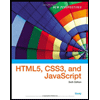
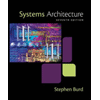