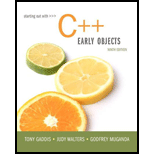
Concept explainers
Explanation of Solution
Output of the Given
//Header files
#include<iostream>
using namespace std;
//main function
int main()
{
//Declare and initialize the variables
const int UPPER = 8, LOWER = 2;
int num1, num2, num3 = 12, num4 = 3;
/*Check whether the value of 'num3' is less than 'num4' if it is true then 'UPPER' value is stored in the variable 'num1' otherwise 'LOWER' value is stored in the variable 'num1'*/
num1 = num3 < num4 ? UPPER : LOWER;
/*Check whether the value of 'num4' is greater than 'UPPER' if it is true then 'num3' value is stored in the variable 'num2' otherwise 'LOWER' value is stored in the variable 'num2'*/
num2 = num4 > UPPER ? num3 : LOWER;
//Print the values
cout << num1 << " " << num2 << endl;
//return statement
return 0;
...
Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Starting Out with C++: Early Objects (9th Edition)
- in c language typedef _people { int age; char name[ 32 ] ; } People_T ; People_T data [ 3 ]; Using string lib function, Assign 30 and Cathy to the first cell, Assign 40 and John to the second cell and Assign 50 and Tom to the third cellarrow_forwardmy_func is a function as follows. What is the value of a at the end of the code beneath? function a = my_func (a) a = a + 1; end a = 0; for i = 1:3 my func (a); end a = my_func (a);arrow_forwardC# program in Visual Studio Code. I need to be able to enter five integer values and then have the Unique values entered display. Private member variable to store 5 unique values (hint: use an Array or a List) Public function to get 5 unique values from the user and store them in the member variable loop to get the numbers, if a number is already stored, ignore it and keep looping until you have 5 unique numbers if a number is out of range, don't store the value, throw an exception and handle it in such a way that you don't break the loop but do message the user that the value was out of rangearrow_forward
- In Python, grades_dict = {'Wally': [87,96,70], 'Eva': [100,87,90], 'Sam': [94,77,90], 'Katie': [100,81,82], 'Bob': [83,65,85]} Write your own describe function that produces the same 8 statistical results, for each one of the columns, that the built-in describe() function does. Note 1: Use the sample standard deviation formula (that is, the denominator is: N-1) Note 2: Your algorithm should work for any number of columns not just for 5 Note 3: You can use the np.percentile() for the 25% and 75% percentile as well as the sort() built-in functionsarrow_forwardC++ programming Chapter(s) Covered: Chapter 1-8 Concepts tested by the program: Working with one dimensional parallel arrays Use of functions Use of loops and conditional statements Project Description The Lo Shu Magic Square is a grid with 3 rows and 3 columnsshown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly. Each number 1 – 9must not be used more than once. So, if you were to add up thenumbers used, The sum of each row, each column and each diagonal all add upto the same number, Write a program that simulates a magic square using 3 onedimensional parallel arrays of integer type. Each one the arrays corresponds to a row of the magicsquare. The program asks the user to enter the values of the magicsquare row by row and informs the user if the grid is a magicsquare or not. See the sample outputs for more clarification. Project Specifications Input for this project: Values of the grid (row by row) Output for this…arrow_forwardInstructions Write a function create_password() expects two parameters: pet_name (a string) and fav_number (an integer). The function returns a new password generated using the following pattern: fav_number followed by the pet_name followed by the star * and fav_number again (see the example below). Create a program that gets a pet name and a favorite number as input from the user, calls the above function, and then prints the output as shown below. Example Input: Angel 3 Output: Your new password is "3Angel*3". Note that the double quotes are part of the output. The assert in the provided template is checking that this function call is returning the correct value (i.e., a correctly-formed string). assert create_password("Angel", 3) == "3Angel*3" Hints Remember that you cannot directly concatenate an integer and a string - you need to convert an integer into a string using either str() or by using f-strings. Troubleshooting If you are getting AssertionError make sure that your…arrow_forward
- Arduino codearrow_forwardInstructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and a character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main(), there are two Persons already created: one Male Person and one Female Person. Your task is to first ask the user for the age of the Male Person and the age of the Female Person. Then, define and declare a function called createKidPerson() which has the following definition: Return type - Person Name - createKidPerson Parameters Person father - the father of the kid to be created Person mother - the mother of the kid to be created Description - creates a new Person and returns this. The age of this Person will be set to 1 while its gender will be set based on the rules mentioned above. Finally, create a new Person and call this createKidPerson() in the main and then pass this newly…arrow_forwardIn Python, grades_dict = {'Wally': [87,96,70], 'Eva': [100,87,90], 'Sam': [94,77,90], 'Katie': [100,81,82], 'Bob': [83, 65, 85]} write your own describe function that produces thesame 8 statistical results, for each one of the columns, that the built-in describe() function does.Note 1: Use the sample standard deviation formula (that is, the denominator is: N-1)Note 2: Your algorithm should work for any number of columns not just for 5Note 3: You can use the np.percentile() for the 25% and 75% percentile as well as the sort()built-in functionsarrow_forward
- Program Name: <LastNameFirstInit>_PetShelter You work at a Pet Shelter that take in homeless pets. You have been tasked with creating a report that shows a list of all the dog breeds currently staying at the shelter. This list should include the breed name along with the count for each breedcurrently being housed at the shelter. In addition, at the end, the report should display the name of breed that we have the most (max) of currently at the shelter. In addition, your program should utilize methods for building the report and searching for the max breed count. Two parallel arrays have been provided. You can use the following code to get you started. //START CUTTING CODE HERE public class MichakR_PetShelter { public static void main(String[] args) { String[] breedNames = {"Bulldog", "German Shepherd", "Golden Retriever", "Beagle", "Poodle", "Boxer", "Mixed Dog", "Husky"}; int[] breedCounts = {2,5,3,1,8,1,11, 3}; //generate report //get Max Breed //print report } public…arrow_forwardDeclare an enumerated data type named Direction with enumerators for North, South, East, and West.arrow_forward#include <iostream>using namespace std;struct item{ int id; float price;} s[50];int size = 0;void addData() { cout << "Enter an item data " << endl; cout << "Enter id: "; cin >> s[size].id; cout << "Enter price: "; cin >> s[size].price; cout << "successfully added" << endl << endl; size++; for (int i = 0; i < size; i++) { for (int j = i + 1; j < size; j++) { if (s[i].price > s[j].price) { struct item t = s[i]; s[i] = s[j]; s[j] = t; } } }}void retrivePrice() { int id; cout << "enter the element id:"; cin >> id; cout << endl; int i; for (i = 0; i < size; ++i) { if (id == s[i].id) { cout << "price for this item: " << s[i].price << endl << endl; break; } } if (i == size) cout…arrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
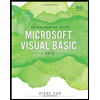
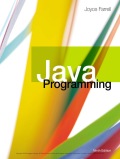