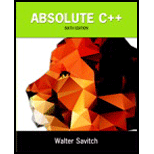
Write a

List of variables:
- hours: Store the time in hours.
- minutes: Store the time in minutes.
- answer: Store the value response ‘y’ or ‘n’.
- result: To store the values of ‘A’ or ‘P’ for AM or PM.
List of functions used:
- getTime(): Used to take values of hours and minutes using call by reference parameters.
- output(): To show the output for time.
- conversion(): To convert the time either in AM or PM.
- cin(): To take input from input streams like keyboard, files etc.
- cout(): To display the output.
Summary Introduction:
Program will use the Main () method to prompt the user to enter the time in 24-hour notation and convert it in 12-hour notation. For this, three functions are provided: one for input values, second for conversion and third for output values.
Program Description:
The purpose of the program is to convert the time from 24-hour notation to 12-hour notation.
Explanation of Solution
Program:
Following is the C++ program to convert the time from 24-hour notation to 12-hour notation.
#include <iostream> using namespace std; char conversion(int& hours); void getTime(int& hours, int& minutes); void output(int hours, int minutes, char result); int main(){ int hours, minutes; char answer; do{ getTime(hours, minutes); char cp = conversion(hours); output(hours, minutes, cp); cout<< "\nDo you want to calculate for more time? "; cin>> answer; }while(answer == 'Y' || answer == 'y'); cout<< "\nThank you! "; return 0; } void getTime(int& hours, int& minutes){ //Enter the hours and minutes as call-by-reference parameters cout<< "Enter the time in hours: "; cin>> hours; cout<< "Enter the time in minutes: "; cin>> minutes; } char conversion(int& hours){ char result; int division; if(hours <= 12){ result = 'A'; } else{ division = hours % 12; if(division == 0){ hours = 00; } else{ hours = division; } result = 'P'; } return result; } void output(int hours, int minutes, char result){ cout<< "The time is: " << hours<< ":" << minutes << " " << result << "M"; cout<<endl; }
Explanation:
In the above program, under main function, getTime() function which has two parameters. Hours and minutes is called to enter the values of hours and minutes. After that, conversion() function which has one parameter is called and output is stored in the variable cp. Finally, the output() function is used to display the result.
Sample Output:
Enter the time in hours: 15 Enter the time in minutes: 24 The time is: 3:24 PM Do you want to calculate for more time? y Enter the time in hours: 11 Enter the time in minutes: 39 The time is: 11:39 AM Do you want to calculate for more time? n Thank you!
Want to see more full solutions like this?
Chapter 4 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Starting Out With Visual Basic (8th Edition)
SURVEY OF OPERATING SYSTEMS
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with Python (4th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
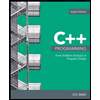
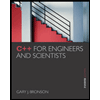
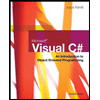
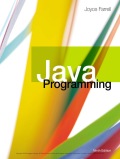