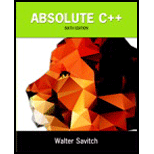
Write a

List of variables:
- hours: Store the time in hours.
- minutes: Store the time in minutes.
- answer: Store the value response ‘y’ or ‘n’.
- result: To store the values of ‘A’ or ‘P’ for AM or PM.
List of functions used:
- getTime(): Used to take values of hours and minutes using call by reference parameters.
- output(): To show the output for time.
- conversion(): To convert the time either in AM or PM.
- cin(): To take input from input streams like keyboard, files etc.
- cout(): To display the output.
Summary Introduction:
Program will use the Main () method to prompt the user to enter the time in 24-hour notation and convert it in 12-hour notation. For this, three functions are provided: one for input values, second for conversion and third for output values.
Program Description:
The purpose of the program is to convert the time from 24-hour notation to 12-hour notation.
Explanation of Solution
Program:
Following is the C++ program to convert the time from 24-hour notation to 12-hour notation.
#include <iostream> using namespace std; char conversion(int& hours); void getTime(int& hours, int& minutes); void output(int hours, int minutes, char result); int main(){ int hours, minutes; char answer; do{ getTime(hours, minutes); char cp = conversion(hours); output(hours, minutes, cp); cout<< "\nDo you want to calculate for more time? "; cin>> answer; }while(answer == 'Y' || answer == 'y'); cout<< "\nThank you! "; return 0; } void getTime(int& hours, int& minutes){ //Enter the hours and minutes as call-by-reference parameters cout<< "Enter the time in hours: "; cin>> hours; cout<< "Enter the time in minutes: "; cin>> minutes; } char conversion(int& hours){ char result; int division; if(hours <= 12){ result = 'A'; } else{ division = hours % 12; if(division == 0){ hours = 00; } else{ hours = division; } result = 'P'; } return result; } void output(int hours, int minutes, char result){ cout<< "The time is: " << hours<< ":" << minutes << " " << result << "M"; cout<<endl; }
Explanation:
In the above program, under main function, getTime() function which has two parameters. Hours and minutes is called to enter the values of hours and minutes. After that, conversion() function which has one parameter is called and output is stored in the variable cp. Finally, the output() function is used to display the result.
Sample Output:
Enter the time in hours: 15 Enter the time in minutes: 24 The time is: 3:24 PM Do you want to calculate for more time? y Enter the time in hours: 11 Enter the time in minutes: 39 The time is: 11:39 AM Do you want to calculate for more time? n Thank you!
Want to see more full solutions like this?
Chapter 4 Solutions
Absolute C++
Additional Engineering Textbook Solutions
C++ How to Program (10th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
C Programming Language
Software Engineering (10th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Write a program whose input is two integers and whose output is the two integers swapped. Ex: If the input is: 3 8 the output is: 8 3 Your program must define and call the following function. swap_values() returns the two values in swapped order.def swap_values(user_val1, user_val2) I get all tests passed except one.: the one in the bold. It puts parentheses around the answer instead of what they want for output. 0 / 2 Output differs. See highlights below. Input 4 5 Your output (5, 4) Expected output 5 4 2: Unit testkeyboard_arrow_up 2 / 2 swap_values(-1, 10) Your output swap_values(-1, 10) correctly returned 10 -1 3: Unit testkeyboard_arrow_up 3 / 3 swap_values(9, 0) Your output swap_values(9, 0) correctly returned 0 9 4: Unit testkeyboard_arrow_up 3 / 3 swap_values(11, 11) Your output swap_values(11, 11) correctly returned 11 11arrow_forwardWrite a program that reads a list of names and total points for students until -1 is entered. After gettingall of names and points, it will output them as names and grades. Write your program using a functionthat gets the points as parameter and calculates the grade and return it. No input validation is required.Also write a function that unit test your grade calculator function.Bonus (5 points): validate your input in any way that you think works better for this situation.Show an error messageBring the points to a valid rangeReturn a value that shows an error has occurredA[94,100] A-[90,94) B+[87,90) B[84,87) B-[80,84) C+[77,80) C(74,77) C-[70,74) D+[67,70) D[61,67) F[0,61)Sample input:Lee 39Lua 86Mary 91Stu 72-1Sample output:Lee FLua BMary AStu C-arrow_forwardWrite a program that inputs a line of text and a search string from the keyboard. Using function strstr, locate the first occurrence of the search string in the line of text, and assign the location to variable searchPtr of type char *. If the search string is found, print the remainder of the line of text beginning with the search string. Then, use strstr again to locate the next occurrence of the search string in the line of text. If a second occurrence is found, print the remainder of the line of text beginning with the second occurrence. input Input a line of text and a search string. Maximum number of char is 200. Output Print the remainder of the line of text beginning with the search string.arrow_forward
- The ceiling of a floating-point number x is the smallest integer that is still larger than or equal to x. Alternatively, the ceiling of a floating-point number x is what you get when you round x up to the nearest integer. For example, the ceiling of 2.1 is 3, the ceiling of 0.9 is 1, the ceiling of -4.5 is -4, etc. Write a function called ceiling() to compute the ceiling of a float input parameter that returns one integer value. You may not use python’s ceil() or floor() functions. Your function may use int()/float() functions, and the floor division operator (i.e., '//').arrow_forwardprogram with a function. program with a function.arrow_forwardThis is python programming Create a function based on the following information: You are given two strings with words separated by commas. Try to find what is common between these strings. The words in the same string don't repeat. Your function should find all of the words that appear in both strings. The result must be represented as a string of words separated by commas in alphabetic order. Input: Two arguments as strings. Output: The common words as a string. Return: Nothing is returnedarrow_forward
- Write a simple calculator program with four operations: add, subtract, multiply, and divide. Create a separate function for each that returns the result of the operation (for example, an “add” function that returns the sum of two values). In the main() function, the program should ask the user to enter two numbers (floating point type) separated by a '+', '-', '*', or '/' character. The program should then call the appropriate function and display the result of the operation.arrow_forwardWrite a program that prompts the user to input a sequence of characters and outputs the number of vowels. (Use the function isVowel written in part 1). Write the function prototype for the isVowel function Write the function call for the isVowel function Attach the input and output of a successful execution of your program below (Hint: Use the snipping tool to take screenshots):arrow_forwardTask 4 Take a string as a input from the user with all small letters. Then print the next alphabet in sequence for each alphabet found in the input. Hint: You need to use functions ord() and chr(). The ASCII value of 'a' is 97 and 'z' is 122. Example 1: Input: abcd Output: bcde Example 2: Input: the cow Output: uifldpx Example 3: [Must fulfil this criteria] Input: xyzabc Output: yzabcdarrow_forward
- Write a program which will take marks of three quizzes as input, then call a function void ScaleUp(float q1, float q2, float q3) to check if the marks of any of the quiz ‘q’ is less than the average of rest of two quizzes ‘a’. If marks of any quiz is less than the average of the rest of two marks then add the difference between the average of the two quizzes and the quiz itself to q i.e., q = a - q, to scale up the quiz marks.For example, if q1 = 5, q2 = 8 and q3 = 9 then your function will change the marks of quiz 1 using the steps below:A = (q3 + q2 )/2 => (8+9)/2=> 8.5 Diff = A - q1 => 8.5 - 5 => 3.5 Q1 = Q1 + Diff => 5 + 3.5 => 8.5Note that the marks are input from the user, so there might be three cases I. if q1 < q2 + q3 orIi. if q2 < q1 + q3 orIii. if q3 < q1 + q2arrow_forwardWrite a function that finds and returns the smallest value of n for which: 1 + 2 + 3 + ... + n is greater than or equal to max (where max is a number input from the user). You can use the following prototype: int SmallestValue(int); In the main program be sure to declare all variables and show the call. Be user friendly! Sample run: What is the max number: 23 The smallest value of n where the sum of 1 to n is greater or equal to 23 is: 7 Again? Y What is the max number: 12 The smallest value of n where the sum of 1 to n is greater or equal to 12 is: 5 Again? N in c++ please use basic coding I'm not too advancedarrow_forwardWrite a python program to solve this problem. You have been hired by the Abahani football club to write a function that will calculate the total bonus on the yearly earnings of each player for the total goals they have scored. Since the number of players will vary, you decide to use the "*args" technique that you learned in your CSE110 class. (No need to take any input from the user. Call the functions and print the values inside the function.) For each player: pass the name, yearly earning, the total goal scored this season, bonus percent per goal. Additionally, If the goal scored is above 30, add an extra bonus of 10000 taka. If it is between 20 and 30 inclusive, add an extra 5000 taka. Function call:function_name("Neymar", 1200000, 30, 10, "Jamal", 700000, 19, 5)Output:Neymar earned a bonus of 3605000 Taka for 30 goals.Jamal earned a bonus of 665000 Taka for 19 goals.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
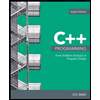