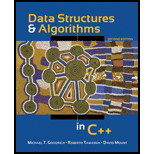
Explanation of Solution
Program code:
//include the required header files
#include <iostream>
#include<time.h>
//use the std namespace
using namespace std;
//Declare n as type of constant
const int n = 10;
//Implementation of prefixAverages1 array
double *prefixAverages1(int X[])
{
//Declare a as integer type
int a;
//Decalre A as double pointer type array
double *A = new double[n];
//Iterate the loop
for (int i = 0; i <= n - 1; i++)
{
//assign 0 to a
a = 0;
//Iterate the loop
for (int j = 0; j <= i; j++)
{
//calculate a = a+X[j] value
a = a + X[j];
}
//assign a / (i + 1)
A[i] = a / (i + 1);
}
//return value of the array A
return A;
}
//Implementation of prefixAverages1 array
double *prefixAverages2(int X[])
{
//Declare s and assign 0
int s = 0;
//Decalre A as double pointer type array
double *A = new double[n];
//Iterate the loop
for (int i = 0; i <= n - 1; i++)
{
//calculate s = s+X[i] value
s = s + X[i];
//assign s / (i + 1)
A[i] = s / (i + 1);
}
//return A
return A;
}
int main()
{
//Decalre X as type of an array
int X[n];
//Declare differenceValue1, differenceValue2 as type of double
double differenceValue1, differenceValue2;
//Declare timeInseconds1, timeInseconds2 as type of double
double timeInseconds1, timeInseconds2;
//Declare i as type of integer
int i = 0;
//Iterate the loop
while (i <= n)
{
//assign i to X[i]
X[i] = i;
//increment i
i++;
}
//Declare resultimeEntrie1,resultimeEntrie2 as type of double
double *resultimeEntrie1 =0, *resultimeEntrie2 = 0;
// declare clock_t objects as timeEntrie1,timeEntrie2
clock_t timeEntrie1, timeEntrie2;
timeEntrie1 = clock();
//store the return value of prefixAverages1(x)
resultimeEntrie1 = prefixAverages1(X);
//Declare k as integer type and assign 0
int k = 0;
// initialize timeEntrie2
timeEntrie2 = clock();
// get the differenceValueerence of two object values
differenceValue1 = (double)timeEntrie2 - (double)timeEntrie1;
// calculate timeInseconds
timeInseconds1 = differenceValue1 / CLOCKS_PER_SEC;
// display statement for timeInseconds
cout << " prefixAverages1(X) " << endl;
//Display statement
cout<<"For n = " << n << " elements then it take " << timeInseconds1 << " seconds" << endl;
// declare clock_t objects as timeEntrie3,timeEntrie4
clock_t timeEntrie3, timeEntrie4;
timeEntrie3 = clock();
//store the return value of prefixAverages2(x)
resultimeEntrie2 = prefixAverages2(X);
timeEntrie4 = clock();
// calculate the differenceValue of two object values
differenceValue2 = (double)timeEntrie4 - (double)timeEntrie3;
// calculate timeInseconds
timeInseconds2 = differenceValue2 / CLOCKS_PER_SEC;
// display statement for timeInseconds
cout << " prefixAverages2(X)" << endl;
//Display statement
cout<<"\n For n = " << n << " elements then it take " << timeInseconds2 << " seconds" << endl;
return 0;
}
Explanation:
The above program snippet is used to implement “prefixAverages1()” and “prefixAverages2()”. In the code,
- Include the required header files.
- Use the “std” namespace.
- Declare the constant “n”.
- Define the method “prefixAverages1()”.
- Declare the required variables.
- Declare an array “A”.
- Iterate a “for” loop.
- Calculate the value of “a”.
- Iterate a “for” loop.
- Calculate the value of “a”.
- Assign a value “a/(i+1)”.
- Return the value of “A”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Data Structures and Algorithms in C++
- Do you think that computers should replace teachers? Give three references with your answer.arrow_forwardIs online learning or face to face learning better to teach students around the around the world? Give reasons for your answer and provide two references with your response. What are benefits of both online learning and face to face learning ? Give two references with your answer. How does online learning and face to face learning affects students around the world? Give two references with your answer.arrow_forwardExplain Five reasons if computers should replace teachers. Provide three references with your answer. List three advantages and three disadvantages face to face learning and online learning may have on children. Provide two references with your answer.arrow_forward
- You were requested to design IP addresses for the following network using the address block 10.10.10.0/24. Specify an address and net mask for each network and router interfacearrow_forwardFor the following network, propose routing tables in each of the routers R1 to R5arrow_forwardFor the following network, propose routing tables in each of the routers R1 to R5arrow_forward
- Using R language. Here is the information link. http://www.cnachtsheim-text.csom.umn.edu/Kutner/Chapter%20%206%20Data%20Sets/CH06PR18.txtarrow_forwardUsing R languagearrow_forwardHow can I type the Java OOP code by using JOptionPane with this following code below: public static void sellCruiseTicket(Cruise[] allCruises) { //Type the code here }arrow_forward
- Draw a system/level-0 diagram for this scenario: You are developing a new customer relationship management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary. Example of what a level-0 diagram looks like is attached.arrow_forwardWhat is the value of performing exploratory data analysis in designing data visualizations? What are some examples?arrow_forwardDraw a level-0 diagram for this scenario: You are developing a new customer relationship management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
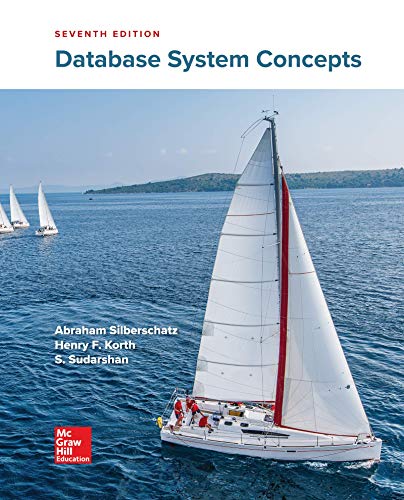
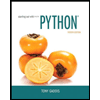
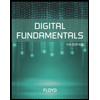
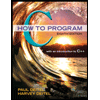
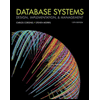
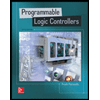