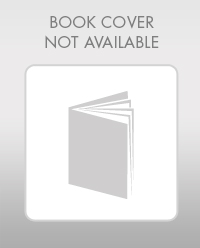
Explanation of Solution
a.
Given code:
'if the condition is true
If intX > 100
'Set the result
lblResult.Text = "Invalid Data"
'End the statement
End If
Error in the statement:
The “Then” keyword is missing in the “If” statement...
Explanation of Solution
b.
Given code:
'Create a variable "str" and set the value
Dim str As String = "Hello"
'Create an integer variable "intLength"
Dim intLength As Integer
'set the value of "intLength" as the length of "str"
intLength = Length(str)
Error in the statement:
The last statement should read “intLength = str.Length”...
Explanation of Solution
c.
Given code:
'if the condition is true
If intZ < 10 Then
'set the value of result
lblResult.Text = "Invalid Data"
Error in the statement:
The “End If” statement is missing in the above snippet.
Corrected statement:
'if the condition is true
If intZ < 10 Then
Explanation of Solution
d.
Given code:
'Create a variable "str" and set the value
Dim str As String = "123"
'if the condition is true
If str.IsNumeric Then
'set the result
lblResult.Text = "It is a number."
'end if condition
End If
Error in the statement:
The If statement should read: “If IsNumeric(str) Then”
Corrected statement:
'Create a variable "str" and set the value
Dim str As String = "123"
'if the condition is true
If IsNumeric(str) Then
'set the result
lblResult...
Explanation of Solution
Given code:
'start select case
Select Case intX
'case 1
Case < 0
'set the result
lblResult. Text = “Value too low”
'case 2
Case > 100
'set the result
lblResult. Text = “Value too high”
'else case
Case Else
'set the result
lblResult. Text =”Value just right”
'end select
End Select
Explanation:
The above code snippet is used to execute select statements. In the code,
- Start a select case
- Case 1 “<0”
- Set the result as “Value too low”
- Case 2 “>100”
- Set the result as “Value too high”
- Else Case
- Set the result as “Value just right”
- Case 1 “<0”
- End select statement.
Error in the statement:
The “Is” keyword is missing in the “Select Case” statements...

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Starting Out With Visual Basic (8th Edition)
- Q2: - Design In Visual Basic a form and write a code to find the largest value of N numbers using Do While Loop. Use InputBox function to read numbers.arrow_forwardNumber 3: Write a pseudocode and draw a flowchart to read age of a user. You should display the suitable message by using the following scheme: 0-5 - Baby6-14 - Child15-35 - Young36-55 - Middle aged56 & above - oldarrow_forwardPlease Debug this 2arrow_forward
- Please note only looking for the solution to problem 2 but problem 1 is needed to help you understand what is being asked *must be created using Visual Studio and C# Problem1 Word Counter Create an application with a method that accepts a string as an argument and returns the number of words it contains. For instance, if the argument is "Four score and seven years ago," the method should return the number 6. The application should let the user enter a string, and then it should pass the string to the method. The number of words in the string should be displayed. Average Number of Letters Problem2 Modify the program you wrote for Problem 1 (Word Counter) so it also displays the average number of letters in each word.arrow_forwardTrue or False 3. Variable names can have spaces in themarrow_forwardvisual Basicarrow_forward
- __________ are spaces that appear at the end of a string. a. Blank spaces b. Secondary spaces c. Ending spaces d. Trailing spacesarrow_forwardThe C++ keyword used to declare a character is _____. Group of answer choices chars char character string ------------------ Which of the following is an Assignment? a.) int x;b.) x = 5;c.) y = x + 2; Group of answer choices a b c a and b b and c All of the above None of the abovearrow_forwardc# Danielle, Edward, and Francis are three salespeople at Holiday Homes. Write an application named HomeSales that prompts the user for a salesperson initial (D, E, or F) input as a string. Either uppercase or lowercase initials are valid. While the user does not type Z, continue by prompting for the amount of a sale. Issue the error message "Sorry - invalid salesperson" for any invalid initials entered. Keep a running total of the amounts sold by each salesperson. After the user types Z or z for an initial, display each salesperson’s total, a grand total for all sales, and the name of the salesperson with the highest total unless there is a tie. If there is a tie, indicate this in the program's output with the message: "There was a tie". An example of the program is shown below: Enter a salesperson initial >> D Enter amount of sale >> 10 Enter next salesperson intital or Z to quit >> d Enter amount of sale >> 2 Enter next salesperson intital or Z to quit…arrow_forward
- Function for button "Show the Grades": • Name this function by your first name (for example, function fadil). • The user should fill all the textboxes: "Exam-1", "Exam-2", "Project" and "Exam-3" and then press the button. In case one of the textboxes is empty, a message will appear in alert box with the text "You should fill all the textboxes", This page says CSCI375 Grades You should fill all the textboxes Exam-1 78 Exam-2 Project 67 Exam-3 66 Show the Grades Your Grades: Publishing Date Published on: Change Background Color • The user should enter all the grades of the activities greater than or equal to 60, otherwise a message will appear in alert box with the text "You failed one of the activities", This page says CSCI375 Grades You failed one of the activities Exam-1 59 Exam-2 88 Project 76 Exam-3 90 Show the Grades Your Grades: Publishing Date Published on: Change Background Color After filling all the textboxes, entering the correct grades and pressing the button "Show the…arrow_forwardQ: Textboxes are often used to receive input from the user. a) true b)Falsearrow_forwardselect pre increment operators .A variable++ .B ++variable .C variable-- .D --variablearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
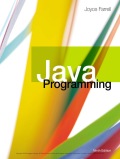
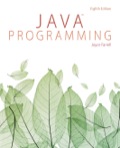
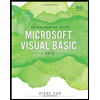