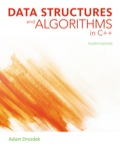
Stack:
Stack is a container in which elements inserted and removed in LIFO (Last In First Out) manner.
- “top” is address of top element of stack.
- Basic stack operations are given below:
- push(): Insert an object into stack top.
- pop(): Delete object in stack top.
- top(): Get object in stack top.

Explanation of Solution
- a.
Given stack is “S” and the additional stacks are “S1” and “S2”.
The elements in stack “S” can be reverse as shown below:
- First pop each element from “S” and push it into “S1” till “S” becomes empty.
- Pop each element from “S1” and push it into “S2” till “S1” becomes empty.
- Pop each element from “S2” and push it into “S” till “S2” becomes empty.
- Now “S” contains elements in reverse order.
Example,
Initially “S” contains 1, 2, 3 and 4.
First pop each element from “S” and push it into “S1”.
Then pop each element from “S1” and push it into “S2”.
Last pop each element from “S2” and push it into “S”.
Finally “S” contains in reverse order.
Queue:
- Queue is another data structure in which insertion and removal of elements are in FIFO(First In First Out) manner.
- Basic operations are given below:
- Enqueue: Insert element into back of queue.
- Dequeue: Remove item from queue’s front.
Explanation of Solution
- b.
Given stack is “S” and additional queue is “Q”.
The elements in stack “S” can be reverse as shown below:
- Pop each element from “S” and insert it into “Q” till “S” becomes empty.
- Delete each element from “Q” and push it into “S” till “Q” becomes empty.
- Now stack “S” contains elements in reverse order.
Example,
Initially “S” contains 1, 2, 3 and 4.
First pop each element from “S” and insert it into “Q”.
Then delete each element from “Q” and push it into “S”.
Finally, “S” contains in reverse order.
Explanation of Solution
- c.
Given stack is “S”, additional stack is “S1” and additional variables are “Num”, Num2 and “top_element”. The elements in stack “S” can be reverse as shown below.
- “Num” is number of elements initially in stack “S”.
- Decrement “Num” by one.
- Till “Num” becomes zero.
- Equate “Num” into “Num2”.
- Pop stack top of “S” and store in “top_element”.
- Pop “Num2” elements from “S” and push into “S1”.
- Then push element in “top_element” into “S”.
- Then pop each element from “S1” and push into “S” till “S1” becomes empty.
- Decrement value of “Num” by one.
- Finally “S” contains elements in reverse order.
Example,
Initially “S” contains 1, 2, 3 and 4. Stack contains four elements. So, “Num” is 4. Decrement it by one.
First iteration:
“Num” is 3, pop stack top and store in “top_element”. Then pop other three elements from “S” and push into “S1”.
- Then push element in “top_element” into “S” and pop each element from “S1” and push into “S”.
Second iteration:
“Num” is 2, pop stack top and store in “top_element”. Then pop other two elements from “S” and push into “S1”.
- Push element in “top_element” into “S” and pop each element from “S1” and push into “S”.
Third iteration:
“Num” is 1, pop stack top and store in “top_element”. Then pop one element from “S” and push into “S1”.
- Push element in “top_element” into “S” and pop each element from “S1” and push into “S”
Fourth iteration:
“Num” is 0, exit loop.
Finally, the stack “S” contains elements in reverse order.
Want to see more full solutions like this?
Chapter 4 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- I need to make a parallel version of this sequential code.arrow_forwardBenefits of using arrays as instance variables: What are the advantages of incorporating arrays as instance variables within a class? Initializing and managing arrays: How do you initialize and manage arrays within class constructors and mutators (setters)? Example of using arrays as instance variables: Share an example where you have used arrays as instance variables and discuss its application in a real-world scenario. Common mistakes with arrays as instance variables: What are some common mistakes to avoid when working with arrays as instance variables? Information hiding violations: What is the potential violation of information hiding when using arrays as instance variables? How can this be resolved?arrow_forwardDo you think that computers should replace teachers? Give three references with your answer.arrow_forward
- Is online learning or face to face learning better to teach students around the around the world? Give reasons for your answer and provide two references with your response. What are benefits of both online learning and face to face learning ? Give two references with your answer. How does online learning and face to face learning affects students around the world? Give two references with your answer.arrow_forwardExplain Five reasons if computers should replace teachers. Provide three references with your answer. List three advantages and three disadvantages face to face learning and online learning may have on children. Provide two references with your answer.arrow_forwardYou were requested to design IP addresses for the following network using the address block 10.10.10.0/24. Specify an address and net mask for each network and router interfacearrow_forward
- For the following network, propose routing tables in each of the routers R1 to R5arrow_forwardFor the following network, propose routing tables in each of the routers R1 to R5arrow_forwardUsing R language. Here is the information link. http://www.cnachtsheim-text.csom.umn.edu/Kutner/Chapter%20%206%20Data%20Sets/CH06PR18.txtarrow_forward
- Using R languagearrow_forwardHow can I type the Java OOP code by using JOptionPane with this following code below: public static void sellCruiseTicket(Cruise[] allCruises) { //Type the code here }arrow_forwardDraw a system/level-0 diagram for this scenario: You are developing a new customer relationship management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary. Example of what a level-0 diagram looks like is attached.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
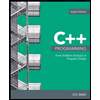
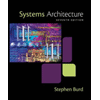
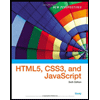
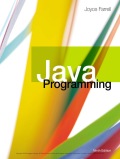
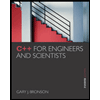