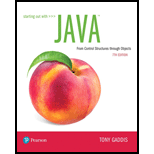
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 4, Problem 19AW
Modify the code you wrote in Question 18 so it adds all of the numbers read from the file and displays their total.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
All in one file
Assignment 5A: Multiple Frequencies. In the last assignment, we calculated the frequency of
a coin flip. This required us to have two separate variables, which we used to record the number
of heads and tails. Now that we know about arrays, we can track the frequency of all numbers in
a randomly generated sequence.
For this program, you will ask the user to provide a range of values (from 1 to that number,
inclusive) and how long of a number sequence you want to generate using that number range.
You will then generate and save the sequence in an array. After that, you will count the number
of times each number occurs in the sequence, and print the frequency of each number.
Hints: You can use multiple arrays for this assignment. One array should hold the
number sequence, and another could keep track of the frequencies of each number.
Sample Output #1:
What's the highest number you want to generate?: 5
How Long of a number sequence do you want to generate?: 10
Okay, we'll generate 10…
Finish this program from the code posted below! Note: There should be two files Main.py and Contact.py
You will implement the edit_contact function. In the function, do the following:
Ask the user to enter the name of the contact they want to edit.
If the contact exists, in a loop, give them the following choices
Remove one of the phone numbers from that Contact.
Add a phone number to that Contact.
Change that Contact's email address.
Change that Contact's name (if they do this, you will have to remove the key/value pair from the dictionary and re-add it, since the key is the contact’s name. Use the dictionary's pop method for this!)
Stop editing the Contact
Once the user is finished making changes to the Contact, the function should return.
Code:from Contact import Contactimport pickledef load_contacts():""" Unpickle the data on mydata.dat and save it to a dictionaryReturn an empty dictionary if the file doesn't exist """try:with open("mydata.dat", 'rb') as file:return…
Chapter 4 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 4.1 - What will the following program segments display?...Ch. 4.2 - How many times will Hello World be printed in the...Ch. 4.2 - How many times will I love Java programming! be...Ch. 4.3 - Write an input validation loop that asks the user...Ch. 4.3 - Write an input validation loop that asks the user...Ch. 4.3 - Write an input validation loop that asks the user...Ch. 4.5 - Name the three expressions that appear inside the...Ch. 4.5 - You want to write a for loop that displays I love...Ch. 4.5 - What will the following program segments display?...Ch. 4.5 - Write a for loop that displays your name 10 times.
Ch. 4.5 - Write a for loop that displays all of the odd...Ch. 4.5 - Write a for loop that displays every fifth number,...Ch. 4.6 - Write a for loop that repeats seven times, asking...Ch. 4.6 - In the following program segment, which variable...Ch. 4.6 - Prob. 4.15CPCh. 4.10 - What is the difference between an input file and...Ch. 4.10 - What import statement will you need in a program...Ch. 4.10 - What class do you use to write data to a file?Ch. 4.10 - Write code that does the following: opens a file...Ch. 4.10 - What classes do you use to read data from a file?Ch. 4.10 - Write code that does the following: opens a file...Ch. 4.10 - You are opening an existing file for output. How...Ch. 4.10 - What clause must you write in the header of a...Ch. 4.11 - Assume x is an int variable, and rand references a...Ch. 4.11 - Assume x is an int variable, and rand references a...Ch. 4.11 - Assume x is an int variable, and rand references a...Ch. 4.11 - Assume x is a double variable, and rand references...Ch. 4 - What will the println statement in the following...Ch. 4 - Prob. 2MCCh. 4 - Prob. 3MCCh. 4 - What is each repetition of a loop known as? a....Ch. 4 - This is a variable that controls the number of...Ch. 4 - The while loop is this type of loop. a. pretest b....Ch. 4 - The do-while loop is this type of loop. a. pretest...Ch. 4 - The for loop is this type of loop. a. pretest b....Ch. 4 - This type of loop has no way of ending and repeats...Ch. 4 - This type of loop always executes at least once....Ch. 4 - This expression is executed by the for loop only...Ch. 4 - Prob. 12MCCh. 4 - This is a special value that signals when there...Ch. 4 - To open a file for writing, you use the following...Ch. 4 - To open a file for reading, you use the following...Ch. 4 - Prob. 16MCCh. 4 - This class allows you to use the print and println...Ch. 4 - This class allows you to read a line from a file....Ch. 4 - True or False: The while loop is a pretest loop.Ch. 4 - True or False: The do-while loop is a pretest...Ch. 4 - True or False: The for loop is a posttest loop.Ch. 4 - True or False: It is not necessary to initialize...Ch. 4 - True or False: One limitation of the for loop is...Ch. 4 - True or False: A variable may be defined in the...Ch. 4 - True or False: In a nested loop, the inner loop...Ch. 4 - True or False: To calculate the total number of...Ch. 4 - // This code contains ERRORS! // It adds two...Ch. 4 - Prob. 2FTECh. 4 - // This code contains ERRORS! int choice, num1,...Ch. 4 - Prob. 4FTECh. 4 - Write a while loop that lets the user enter a...Ch. 4 - Write a do-whi1e loop that asks the user to enter...Ch. 4 - Write a for loop that displays the following set...Ch. 4 - Write a loop that asks the user to enter a number....Ch. 4 - Write a for loop that calculates the total of the...Ch. 4 - Write a nested loop that displays 10 rows of #...Ch. 4 - Convert the while loop in the following code to a...Ch. 4 - Convert the do-while loop in the following code to...Ch. 4 - Convert the following while loop to a for loop:...Ch. 4 - Convert the following for loop to a while loop:...Ch. 4 - Write an input validation loop that asks the user...Ch. 4 - Write an input validation loop that asks the user...Ch. 4 - Write nested loops to draw this pattern:Ch. 4 - Write nested loops to draw this pattern: ## # # #...Ch. 4 - Complete the following program so it displays a...Ch. 4 - Complete the following program so it performs the...Ch. 4 - Prob. 17AWCh. 4 - Prob. 18AWCh. 4 - Modify the code you wrote in Question 18 so it...Ch. 4 - Write code that opens a file named NumberList.txt...Ch. 4 - Prob. 1SACh. 4 - Why should you indent the statements in the body...Ch. 4 - Describe the difference between pretest loops and...Ch. 4 - Why are the statements in the body of a loop...Ch. 4 - Describe the difference between the while loop and...Ch. 4 - Which loop should you use in situations where you...Ch. 4 - Which loop should you use in situations where you...Ch. 4 - Which loop should you use when you know the number...Ch. 4 - Why is it critical that accumulator variables are...Ch. 4 - What is an infinite loop? Write the code for an...Ch. 4 - Describe a programming problem that would require...Ch. 4 - What does it mean to let the user control a loop?Ch. 4 - What is the advantage of using a sentinel?Ch. 4 - Prob. 14SACh. 4 - Describe a programming problem requiring the use...Ch. 4 - How does a file buffer increase a programs...Ch. 4 - Why should a program close a file when its...Ch. 4 - What is a files read position? Where is the read...Ch. 4 - When writing data to a file, what is the...Ch. 4 - What does the Scanner classs hasNext method return...Ch. 4 - What is a potential error that can occur when a...Ch. 4 - Prob. 22SACh. 4 - How do you open a file so that new data will be...Ch. 4 - Sum of Numbers Write a program that asks the user...Ch. 4 - Distance Traveled The distance a vehicle travels...Ch. 4 - Distance File Modify the program you wrote for...Ch. 4 - Pennies for Pay Write a program that calculates...Ch. 4 - Prob. 5PCCh. 4 - File Letter Counter Write a program that asks the...Ch. 4 - Hotel Occupancy A hotels occupancy rate is...Ch. 4 - Average Rainfall Write a program that uses nested...Ch. 4 - Population Write a program that will predict the...Ch. 4 - Largest and Smallest Write a program with a loop...Ch. 4 - Celsius to Fahrenheit Table Write a program that...Ch. 4 - Bar Chart Write a program that asks the user to...Ch. 4 - File Head Display Write a program that asks the...Ch. 4 - Line Numbers Write a program that asks the user...Ch. 4 - Uppercase File Converter Write a program that asks...Ch. 4 - Budget Analysis Write a program that asks the user...Ch. 4 - Random Number Guessing Game Write a program that...Ch. 4 - Random Number Guessing Game Enhancement Enhance...Ch. 4 - ESP Game Write a program that tests your ESP...Ch. 4 - Square Display Write a program that asks the user...Ch. 4 - Dice Game Write a program that plays a simple dice...Ch. 4 - Prob. 22PCCh. 4 - Personal Web Page Generator Write a program that...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
In the following exercises, write a program to carry out the task. The program should use variables for each of...
Introduction To Programming Using Visual Basic (11th Edition)
What are the two modes that a random access file may be opened in? Explain the difference between them.
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
What is the disadvantage of having too many features in a language?
Concepts Of Programming Languages
The current source in the circuit shown generates the current pulse
Find (a) v (0); (b) the instant of time gr...
Electric Circuits. (11th Edition)
Porter’s competitive forces model: The model is used to provide a general view about the firms, the competitors...
Management Information Systems: Managing The Digital Firm (16th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A Personal Fitness Tracker is a wearable device that tracks your physical activity, calories burned, heart rate, sleeping patterns, and so on. One common physical activity that most of these devices track is the number of steps you take each day. The steps.txt file contains the number of steps a person has taken each day for a year. There are 365 lines in the file, and each line contains the number of steps taken during a day. (The first line is the number of steps taken on January 1st, the second line is the number of steps taken on January 2nd, and so forth.) Write a program that reads the file, then displays the average number of steps taken for each month. (The data is from a year that was not a leap year, so February has 28 days.) You don't need to see the file , Just write a code using the "file" like this def main(): with open("steps.txt", "r") as file:arrow_forwardThe average amount Sold function is not run as expect. Please fix it. The input file: sales.txt header in picture. It is over 5000 char. I can't copy. 13492785 2017 Jane North; 1000 78534520 2012 Tim South; 95020192756 2017 Linda East; 15000 19273458 2012 Paul West; 500078520192 2017 Mary Jane Doe; 5001 32278520 2012 Victor Smith; 799514278520 2012 Mary Johnson; 12056192785 2017 Tom Baker; 1300 88278529 2012 Diana Newman; 150089278527 2012 William Peterson; 1420098278528 2012 Jim Gaddis; 120099192785 2017 Laura King; 1000 43278524 2012 Ann McDonald; 2000 The output expect: in picture. #include "Sales.h"#include <iostream>#include <sstream>#include <iomanip>#include <fstream>#include <string>using namespace std; const int MAX_SIZE = 30; void readData(string fileName, Sales salesArr[], int n);double calcSalesAvg(Sales salesArr[], int n);void displayOverAvg(Sales salesArr[], int n, double avg);void writeReport(Sales salesArr[], int n, string…arrow_forwardThe names and student numbers of students are save in a text file called stnumbers.txt. Example of the content of the text file: Peterson 20570856 Johnson 12345678 Suku 87654321 Westley 12345678 Venter 87654321 Mokoena 79012400 Makubela 29813360 Botha 30489059 Bradley 30350069 Manana 30530679 Shabalala 28863496 Smith 87873909 Nilsson 30989698 Makwela 30256607 Govender 30048117 Ntumba 30598303 Ramsamy 29952239 Skosana 29982995 Jameson 30228484 Xulu 29092248 Wasserman 27469352 Bester 28615425 Babane 27154033 Maboya 29897890 Mahlangu 30031338 Majavu 30165970 Myene 30954177 Motaung 30907276 Ramaroka 30804507 Radebe 30007674 Sekake 30017416 Zwane 30038227 Shuro 30238072 Viljoen 28881389 Sithole 45688555 Write a function called displayData() to receive the array and number of elements as parameters and display the names and student numbers of the students with a heading and neatly spaced. Write a function, isValid(), which receives a number as parameter and determines whether the number…arrow_forward
- Chapter 7 - Programming Challenge 15 15. World Series Champions If you have downloaded this book’s source code (the companion Web site is available at www.pearsonhighered.com/gaddis), you will find a file named WorldSeriesWinners.txt. This file contains a chronological list of the winning teams in the World Series from 1903 through 2009. (The first line in the file is the name of the team that won in 1903, and the last line is the name of the team that won in 2009. Note that the World Series was not played in 1904 or 1994, so those years are skipped in the file.) Write a program that lets the user enter the name of a team, and then displays the number of times that team has won the World Series in the time period from 1903 through 2009. Tip: Read the contents of the WorldSeriesWinners.txt file into an ArrayList. When the user enters the name of a team, the program should step through the ArrayList, counting the number of times the selected team appears You only need to submit the…arrow_forwardThis program asks the user to enter 4 names for a team, storing them in an array of Strings. Then the program asks for one of those names again, and a new name to substitute instead of that one. Finally, the program changes the array, making that substitution. This modified list of names is printed on the screen. You can see sample output at the end of this file.arrow_forwardThe Apgar Medical group keeps a patient file for each doctor in the office. Each record contains the patient's first and last name, home address, and birth year. The records are sorted in ascending birth year order. Write a program so that display a count of the number of patients born each year John Hanson, 23 Elm, 1927Mary Locust, 476 Maple, 1950Susan Monroe, 512 Peachtree, 1957Carol Fortune, 2819 Locust, 1960James Fortune, 2819 Locust, 1963Lawrence Fish, 12 Elm, 1968Janice Weiss, 234 Birch, 1971Henry Garza, 199 Second, 1973Kimberly Swanson, 310 Appletree, 1980Louis Claude, 2716 Third, 1981Jill Fox, 12 Oak, 1985Opal Reynolds, 78 County Line, 1987Francis Dumas, 67 Fourth, 1992Madison Conroy, 23 Fifth, 1996Daniel Moy, 100 Sunset, 1987arrow_forward
- BASH FLOW CHART: Create a flow chart to describe an algorithm that takes a text file with format ID,FirstName,Last Name,Street,City and appends a user ID field consisting of a C followed by theemployee ID. For example, the first entries in Lab 2's employees.txt are:0,Douglas L,Eberhard,Addenda Circle,Cornwall1,Elizabeth Sua,Hemauer,Wyatt Way,Peterborough2,Bailey Rae,Lopez,Turnagain Street,Sault Ste. MarieAfter processing, the entries should be:0,Douglas L,Eberhard,Addenda Circle,Cornwall,C01,Elizabeth Sua,Hemauer,Wyatt Way,Peterborough,C12,Bailey Rae,Lopez,Turnagain Street,Sault Ste. Marie,C2arrow_forwardThe file, Program11.txt, on the I: drive contains a chronological list of the World Series’ winning teams from 1903 through 2018. The first line in the file is the name of the team that won in 1903, and the last line is the name of the team that won in 2018. (Note that the World Series was not played in 1904 or 1994. There are no entries in the file indicating this.) Write a program that reads this file and creates a dictionary in which the keys are the names of the teams and each key’s associated value is the number of times the team has won the World Series. The program should also create a dictionary in which the keys are the years and each key’s associated value is the name of the team that won that year. The program should prompt the user for a year in the range of 1903 through 2018. It should thendisplay the name of the team that won the World Series that year and the number of times that team has won the World Series.Allow the user to run the program as many times as possible…arrow_forward$aprintarrow_forward
- >> classicVinyls.cpp For the following program, you will use the text file called “vinyls.txt” attached to this assignment. The file stores information about a collection of classic vinyls. The records in the file are like the ones on the following sample: Led_Zeppelin Led_Zeppelin 1969 1000.00 The_Prettiest_Star David_Bowie 1973 2000.00 Speedway Elvis_Presley 1968 5000.00 Spirit_in_the_Night Bruce_Springsteen 1973 5000.00 … Write a declaration for a structure named vinylRec that is to be used to store the records for the classic collection system. The fields in the record should include a title (string), an artist (string), the yearReleased (int), and an estimatedPrice(double). Create the following…arrow_forwardCargo Container Report. You will be producing a program which will allow the user to generate the container report on the following page in Container Number order or Ship Name order. The program will also the user to lookup the data for any given Container by number. The % cargo weight is the percentage of the cargo of the gross weight (cargo weight/gross weight), % total is the percentage of the cargo for that container of the total amount of cargo unloaded (cargo weight for the container / total cargo unloaded. Hint: you are expected to use methods for your calculations. The accompanying file has the data for the containers unloaded today. The data includes container number int ship name. String tare weight of the container (empty weight) int the gross weight (container plus the cargo). Int You will need to computer the cargo weight as the gross weight minus the tare weight. If you have issues reading the data with the String, you may remove the String. However, you will lose points.…arrow_forwardswitch_player(): we will allow the players to choose their own names, but for our purposes, it is easier to think of them as number (0 and 1, for indexing purposes). We will display their names to them on the screen, but in the background, we will keep track of them based on their number. This function should take 1 argument, an integer (either 0 or 1) representing the player and should return the opposite integer (1 if 0 is entered and 0 if 1 is entered). This is a simple function, but it will make our code cleaner later. Use your constants! Using Thonnyarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
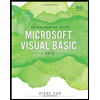
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
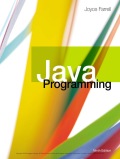
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
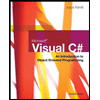
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
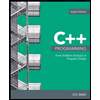
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
C - File I/O; Author: Tutorials Point (India) Ltd.;https://www.youtube.com/watch?v=cEfuwpbGi1k;License: Standard YouTube License, CC-BY
file handling functions in c | fprintf, fscanf, fread, fwrite |; Author: Education 4u;https://www.youtube.com/watch?v=aqeXS1bJihA;License: Standard Youtube License