Concept explainers
Given Program:
The program given in the textbook is given here with comments for better understanding.
File Name: TaxReturn.java
// Class definition
public class TaxReturn
{
// Declare and initialize the required variables
public static final int SINGLE = 1;
public static final int MARRIED = 2;
private static final double RATE1 = 0.10;
private static final double RATE2 = 0.25;
private static final double RATE1_SINGLE_LIMIT = 32000;
private static final double RATE1_MARRIED_LIMIT = 64000;
private double income;
private int status;
/*Constructs a TaxReturn object for a given income and marital status.
@param anIncome the taxpayer income
@param aStatus either SINGLE or MARRIED */
// Method definition
public TaxReturn(double anIncome, int aStatus)
{
income = anIncome;
status = aStatus;
}
// Method definition
public double getTax()
{
// Declare and initialize the required variables
double tax1 = 0;
double tax2 = 0;
/* If the entered status is "Single", compute income tax based on their income */
if (status == SINGLE)
{
/* Check whether the income is less than or equal to $32000 */
if (income <= RATE1_SINGLE_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $32000, compute the tax */
else
{
tax1 = RATE1 * RATE1_SINGLE_LIMIT;
tax2 = RATE2 * (income - RATE1_SINGLE_LIMIT);
}
}
/* If the entered status is "Married", compute income tax based on their income */
else
{
/* Check whether the income is less than or equal to $64000 */
if (income <= RATE1_MARRIED_LIMIT)
{
// If it is, compute the tax
tax1 = RATE1 * income;
}
/* If the income is greater than or equal to $64000, compute the tax */
else
{
tax1 = RATE1 * RATE1_MARRIED_LIMIT;
tax2 = RATE2 * (income - RATE1_MARRIED_LIMIT);
}
}
// Return the tax to the main function
return tax1 + tax2;
}
}
File Name: TaxCalculator.java
// Import the required package
import java.util.Scanner;
/**
This program calculates a simple tax return.
*/
// Class definition
public class TaxCalculator
{
// Main class declaration
public static void main(String[] args)
{
// Create an object for scanner class
Scanner in = new Scanner(System.in);
// Prompt the user to enter the income
System.out.print("Please enter your income: ");
// Store the entered income in the variable
double income = in.nextDouble();
// Prompt the user to enter marital status
System.out.print("Are you married? (Y/N) ");
// Store the entered value in a variable
String input = in.next();
// Declare the variable
int status;
/* Check whether the user input value for marital status is "Y" */
if (input.equals("Y"))
{
/* If it is "Y", store the taxreturn value for a married person in the variable */
status = TaxReturn.MARRIED;
}
/* Check whether the user input value for marital status is "N" */
else
{
/* If it is "N", store the taxreturn value for a single person in the variable */
status = TaxReturn.SINGLE;
}
// Create an object for TaxReturn class
TaxReturn aTaxReturn = new TaxReturn(income, status);
// Display the tax return value based on the user input
System.out.println("Tax: "
+ aTaxReturn.getTax());
}
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
BIG JAVA: LATE OBJECTS
- you can select multipy optionsarrow_forwardFor each of the following, decide whether the claim is True or False and select the True ones: Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would mean that all problems in NP can also be solved in cubic time. If a problem can be solved using Dynamic Programming, then it is not NP-complete. Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time reduction from X to Y and also one from Y to X.arrow_forwardMaximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forward
- Please help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forwardWhat does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forwardPlease assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forward
- need help with a html code and css code that will match this image.arrow_forwardneed help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forward
- Why all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forwardWhy is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forwardWrite the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forward
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageOperations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrA Guide to SQLComputer ScienceISBN:9781111527273Author:Philip J. PrattPublisher:Course Technology Ptr
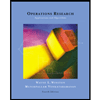
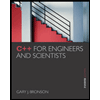
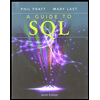
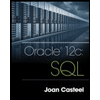