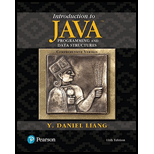
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 3.14, Problem 3.14.1CP
Suppose when you run the following program, you enter the input 2 3 6 from the console. What is the output?
public class Test {
public static void main(String[] args) {
java.util.Scanner input = new java.util.Scanner(System.in);
double x = input.nextDouble();
double y = input.nextDouble();
double z = input.nextDouble();
System.out.println((x < y && y < z) ? “sorted” : “not sorted”);
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Fix the Java code below,
You must have a Boolean variable or return value somewhere in the program.
Sample Output is in the picture
Java - How do I output this statement as a horizontal statement with a newline? Everytime I use System.out.println, it makes the entire output vertical instead of horizontal. Program below.
import java.util.Scanner;
public class LabProgram { public static void main(String[] args) { int x; int y; Scanner scan = new Scanner(System.in); x = scan.nextInt(); y = scan.nextInt(); if(y >= x){ for(int i=x; i<=y; i = i + 5) { System.out.print(i+ " "); (The problem line) } } else{ System.out.print("Second integer can't be less than the first."); } }}
Examine this code and determine what happens when it is run:
1 public class Test {
int x;
2
3
public Test(String t){
System.out.println("Test");
}
public static void main(String[] args) {
Test test = new Test ("boo");
System.out.println(test.x);
}
4
5
6
7
8
10 }
The program has a compile error because Test does not have a default
constructor.
The program has a compile error because System.out.println method cannot be
invoked from the constructor.
) The program runs successfully and prints out:
Test
O The program has a compile error because you cannot create an object from the
class that defines the object.
Chapter 3 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 3.2 - List six relational operators.Ch. 3.2 - Assuming x is 1, show the result of the following...Ch. 3.2 - Can the following conversions involving casting be...Ch. 3.3 - Write an if statement that assigns 1 to x if y is...Ch. 3.3 - Write an if statement that increases pay by 3% if...Ch. 3.3 - Prob. 3.3.3CPCh. 3.4 - Write an if statement that increases pay by 3% if...Ch. 3.4 - What is the output of the code in (a) and (b) if...Ch. 3.5 - Prob. 3.5.1CPCh. 3.5 - Suppose x = 2andy = 3. Show the output, if any, of...
Ch. 3.5 - What is wrong in the following code? if (score =...Ch. 3.6 - Which of the following statements are equivalent?...Ch. 3.6 - Prob. 3.6.2CPCh. 3.6 - Are the following statements correct? Which one is...Ch. 3.6 - Prob. 3.6.4CPCh. 3.7 - Prob. 3.7.1CPCh. 3.7 - a. How do you generate a random integer i such...Ch. 3.9 - Are the following two statements equivalent?Ch. 3.10 - Assuming that x is 1, show the result of the...Ch. 3.10 - (a) Write a Boolean expression that evaluates to...Ch. 3.10 - (a) Write a Boolean expression for |x 5| 4.5....Ch. 3.10 - Assume x and y are int type. Which of the...Ch. 3.10 - Are the following two expressions the same? (a) x...Ch. 3.10 - What is the value of the expression x = 50 x =...Ch. 3.10 - Suppose, when you run the following program, you...Ch. 3.10 - Write a Boolean expression that evaluates to true...Ch. 3.10 - Write a Boolean expression that evaluates to true...Ch. 3.10 - Write a Boolean expression that evaluates to true...Ch. 3.10 - Write a Boolean expression that evaluates to true...Ch. 3.11 - Prob. 3.11.1CPCh. 3.12 - What happens if you enter an integer as 05?Ch. 3.13 - What data types are required for a switch...Ch. 3.13 - What is y after the following switch statement is...Ch. 3.13 - What is x after the following if-else statement is...Ch. 3.13 - Write a switch statement that displays Sunday,...Ch. 3.13 - Prob. 3.13.5CPCh. 3.14 - Suppose when you run the following program, you...Ch. 3.14 - Rewrite the following if statements using the...Ch. 3.14 - Rewrite the following codes using if-else...Ch. 3.14 - Write an expression using a conditional operator...Ch. 3.15 - List the precedence order of the Boolean...Ch. 3.15 - True or false? All the binary operators except =...Ch. 3.15 - Evaluate the following expressions: 2 2 3 2 4 ...Ch. 3.15 - Is (x 0 x 10) the same as ((x 0) (x 10))? Is...Ch. 3 - (Algebra: solve quadratic equations) The two roots...Ch. 3 - (Game: add three numbers) The program in Listing...Ch. 3 - (Algebra: solve 2 2 linear equations) A linear...Ch. 3 - (Random month) Write a program that randomly...Ch. 3 - (Find future dates) Write a program that prompts...Ch. 3 - (Health application: BMI) Revise Listing 3.4,...Ch. 3 - (Financial application: monetary units) Modify...Ch. 3 - Prob. 3.8PECh. 3 - (Business: check ISBN-10) An ISBN-10...Ch. 3 - (Game: addition quiz) Listing 3.3,...Ch. 3 - (Find the number of days in a month) Write a...Ch. 3 - (Palindrome integer) Write a program that prompts...Ch. 3 - (Financial application: compute taxes) Listing...Ch. 3 - (Game: heads or tails) Write a program that lets...Ch. 3 - (Game: lottery) Revise Listing 3.8, Lottery.java....Ch. 3 - Prob. 3.16PECh. 3 - (Game: scissor, rock, paper) Write a program that...Ch. 3 - (Cost of shipping) A shipping company uses the...Ch. 3 - (Compute the perimeter of a triangle) Write a...Ch. 3 - (Science: wind-chill temperature) Programming...Ch. 3 - Prob. 3.21PECh. 3 - (Geometry: point in a circle?) Write a program...Ch. 3 - (Geometry: point in a rectangle?) Write a program...Ch. 3 - (Game: pick a card) Write a program that simulates...Ch. 3 - (Geometry: intersecting point) Two points on line...Ch. 3 - (Use the , ||, and ^ operators) Write a program...Ch. 3 - (Geometry: points in triangle?) Suppose a right...Ch. 3 - (Geometry: two rectangles) Write a program that...Ch. 3 - (Geometry: two circles) Write a program that...Ch. 3 - (Current time) Revise Programming Exercise 2.8 to...Ch. 3 - (Financials: currency exchange) Write a program...Ch. 3 - Prob. 3.32PECh. 3 - (Financial: compare costs) Suppose you shop for...Ch. 3 - Prob. 3.34PE
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
(set and get Methods) Explain why a class might provide a set method and a get method for an instance variable.
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Would the program in Display 12.8 behave any differently if you replaced the using directive using namespace dt...
Problem Solving with C++ (10th Edition)
What data structure does Python use in place of arrays?
Concepts Of Programming Languages
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
Words that have predefined meaning in a programming language are called _____ .
Starting Out With Visual Basic (8th Edition)
What is the difference between exothermic cutting and endothermic cutting, and how do the cut edges differ?
Degarmo's Materials And Processes In Manufacturing
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- I have my code here and I am getting errors (I have also attached my code): " import java.util.Compiler; public class Test Score { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Ënter the first exam Score: "); Double Score1 = scan.nextDouble(); System.out.println("Enter the second exam Score: "); Double Score2 = scan.nextDouble(); System.out.println("Enter the third exam Score: "); Double Score3 = scan.nextDouble(); System.out.println("Enter the fourth exam Score: "); Double Score4 = scan.nextDouble(); System.out.println("Enter the fifth exam Score: "); Double Score5 = scan.nextDouble(); Double total = Score1 + Score2 + Score3 + Score4 + Score5 Double average = total/5; if (average >= 90) { System.out.printf("your average was a: %f and recieved an A in this Class", average ); } else if (average >= 80) {…arrow_forwardprogram that takes a first name as the input, and outputs a welcome message to that name. Ex: If the input is: Chrystal the output is: Hey Chrystal! Welcome to zyBooks!arrow_forwardimport java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forward
- Write an expression that will cause the following code to print "I am an adult" if the value of userAge is greater than 19. 1 import java.util.Scanner; 3 public class AgeChecker { public static void main (String ] args) { 4 int userAge; 7 Scanner scnr = new Scanner(System.in); userAge = scnr.nextInt(); // Program will be tested with values: 18, 19, 20, 21. 8 9 10 if (//your code goes here) { System.out.println("I am a teenager"); } else { System.out.println("I am an adult"); } } 11 12 13 14 15 16 17 18 }arrow_forwardThe following program has 10 blanks, all of which can be deduced from other given parts of the program. blank java.util.Scanner; public class Perimeter { public static Blank main(String [] args) { double length, width, Blank ; Scanner Blank= new Scanner(); System.Blank .print("Enter the length of the rectangle: "); length = scan.nextDouble(); System.out.print("Enter the width of the rectangle: "); width = scan.next Blank(); perimeter = Blank*2 + width*2; System.out. ("%s%f%s, "The perimeter of the rectangle is " + Blank + " inches."); }//end blank method }//end classarrow_forwardWrite code that outputs variable numBaths as follows. End with a newline. Ex: If the input is: 3 the output is: Baths: 3 1 import java.util.Scanner; 2 3 public class OutputTest { public static void main (String [] args) { int numBaths; 4 5 // Our tests will run your program with input 3, then run again with input 6. // Your program should work for any input, though. 7 8 new Scanner(System.in); scnr.nextInt(); 9. Scanner scnr = 10 numBaths %3D 11 12 System.out.println(numBaths); 13arrow_forward
- // ArtShow.java - This program determines if an art show attendee gets a 5% discount // for preregistering. // Input: Interactive. // Output: A statement telling the user if they get a discount or no discount. import java.util.Scanner; public class ArtShow { public static void main(String args[]) { Scanner s = new Scanner(System.in); String registerString; System.out.println("Did you preregister? Enter Y or N: "); registerString = s.nextLine(); // Test input here. If Y, call discount(), else call noDiscount(). System.exit(0); } // End of main() method. // Write discount method here. // Write noDiscount method here. } // End of ArtShow class.arrow_forwardWrite code that outputs variable numBirds as follows. End with a newline. Ex: If the input is: 3 the output is: Birds: 3 1 import java.util.Scanner; 2 3 public class OutputTest { public static void main (String [] args) { int numBirds; 4 // Our tests will run your program with input 3, then run again with input 6. // Your program should work for any input, though. Scanner scnr = new Scanner(System.in); 7 8 10 numBirds scnr.nextInt(); 11 12 /* Your code goes here */ 12arrow_forwardclass Output{public static void main(String args[]){Integer i = new Integer(557);float x = i.floatValue();System.out.print(x);}} What will the given code prints on the output screen.arrow_forward
- 1. What is the output of the following program? import java.util.Scanner; public class Factorial { public static void main(String args[]){ Scanner scanner = new Scanner(System.in); System.out.printIn("Enter the number:"); scanner.nextInt(); int factorial = fact(num); System.out.println("Factorial of int num = entered number is: "+factorial); } static int fact(int n) { int output; if(n==1){ return 1; } output = fact(n-1)* n; return output; } }arrow_forwardIs this the correct Java programming code when repeat the images below using the text method of input and output? import java.util.Scanner; public class Main { public static void main(String[] args) { //scanner object Scanner scnr = new Scanner(System.in); String name,street,city,postalcode; //input System.out.print("What is your name? "); name = scnr.nextLine(); System.out.print("stret name "); street = scnr.nextLine(); System.out.print("City name "); city = scnr.nextLine(); System.out.print("Postal code "); postalcode = scnr.nextLine(); } }arrow_forwardWrite code that outputs variable numDays as follows. End with a newline. Ex: If the input is: the output is: Days: 3 1 import java.util.Scanner; 2 3 public class OutputTest { public static void main (String [] args) { int numDays; 4 6. // Our tests will run your program with input 3, then run again with input 6. // Your program should work for any input, though. Scanner scnr = new Scanner(System.in); numDays 7 8 9. 10 scnr.nextInt(); 11 12 /* Your code goes here */ 13 } 15 } 14arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
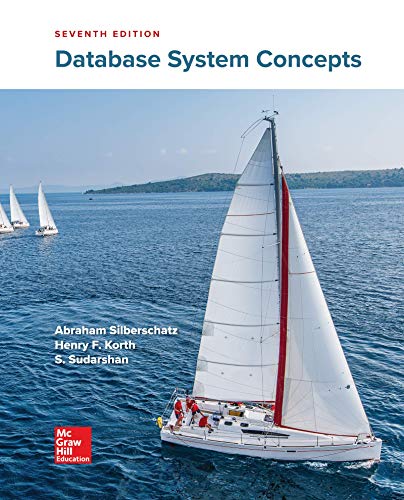
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
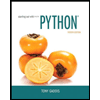
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
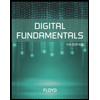
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
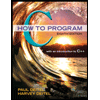
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
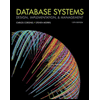
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
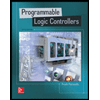
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Java random numbers; Author: Bro code;https://www.youtube.com/watch?v=VMZLPl16P5c;License: Standard YouTube License, CC-BY