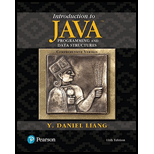
(Algebra: solve quadratic equations) The two roots of a quadratic equation ax2 + bx + c = 0 can be obtained using the following formula:
b2 − 4ac is called the discriminant of the quadratic equation. If it is positive, the equation has two real roots. If it is zero, the equation has one root. If it is negative, the equation has no real roots.
Write a
Note you can use Math. pow(x, 0.5) to compute

Algebra: solve quadratic equation
Program Plan:
- Import the required packages.
- Create a class Exercise
- Define the main method.
- Define the scanner input and prompt user to enter the value of a,b,c.
- Get the input.
- Calculate the discriminant value.
- Condition to validate the discriminant value.
- After validation the value gets of the root gets calculated based on the condition.
- Display the result of roots.
The below program is used to solve the quadratic equation:
Explanation of Solution
Program:
//import the required packages
import java.util.Scanner;
//define the class exercise
public class Exercise
{
public static void main(String[] args)
{
//scanner input
Scanner input = new Scanner(System.in);
/*prompt user to enter the value of the a,b and c*/
System.out.print("Enter a, b, c: ");
//get value of a
double a = input.nextDouble();
//get value of b
double b = input.nextDouble();
//get value of c
double c = input.nextDouble();
//equation to calculate the discriminant
double discriminant = b * b - 4 * a * c;
//condition to validate the discriminant value
if (discriminant < 0)
{
//display result
System.out.println("The equation has no real roots");
}
//condition to validate the discriminant value
else if (discriminant == 0)
{
//equation to calculate the root value
double r1 = -b / (2 * a);
//display result
System.out.println("The equation has one root " + r1);
}
//condition to validate the discriminant value
else
{
// (discriminant > 0)
//equation to calculate the root value
double r1 = (-b + Math.pow(discriminant, 0.5)) / (2 * a);
//equation to calculate the root value
double r2 = (-b - Math.pow(discriminant, 0.5)) / (2 * a);
//display result
System.out.println("The equation has two roots " + r1 + " and " + r2);
}
}
}
Enter a, b, c: 1
2.0
1
The equation has one root -1.0
Additional Output 1:
Enter a, b, c: 1.0
3
1
The equation has two roots -0.3819660112501051 and -2.618033988749895
Additional Output 2:
Enter a, b, c: 1
2
3
The equation has no real roots
Want to see more full solutions like this?
Chapter 3 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Software Engineering (10th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Modern Database Management (12th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- (Algebra: solve linear equations) Write a function that solves the following 2 x 2 system of linear equation: aoox + a01y = bo boa11 - bjao1 X = bja00 - boa10 a10x + any = bị y = agoa11 dooa11 - d01a10 The function header is const int SIZE = 2; bool linearEquation(const double a[][SIZE], const double b[], double result[]); The function returns false if apo1 - aoja10 is 0; otherwise, returns true. Write a test program that prompts the user to enter ao0. d01, a10, a11, bo, bị, and display the result. If aooa11 – a0ia10 is 0, report that "The equation has no solution". A sample run is similar to Programming Exercise 3.3.arrow_forward(Perfect Numbers) An integer number is said to be a perfect number if its factors, including1 (but not the number itself), sum to the number. For example, 6 is a perfect number because 6 =1 + 2 + 3. Write a function isPerfect that determines whether parameter number is a perfect number. Use this function in a program that determines and prints all the perfect numbers between 1and 1000. Print the factors of each perfect number to confirm that the number is indeed perfect.Challenge the power of your computer by testing numbers much larger than 1000.arrow_forward[THIS IS NOT GRADED] The question mentions the bouncy program; this is the bouncy program I have written that computes the total distance traveled by the ball given initial height, bounciness index, and number of bounces: height = float(input("Enter the height from which the ball is dropped: ")) bounciness = float(input("Enter the bounciness index of the ball: ")) distance = 0 bounces = int(input("Enter the number of times the ball is allowed to continue bouncing: ")) for eachPass in range(bounces): distance += height height *= bounciness distance += height print('\nTotal distance traveled is:', distance, 'units.') I will attatch the pre-existing code that the question provided. Thank you for your help.arrow_forward
- (Algebra: solve 2 x 2 linear equations) You can use Cramer's rule to solve the following 2 X 2 system of linear equation: ed – bf ax + by = e cx + dy = f af - eс y ad – bc %3D ad – bc Write a program that prompts the user to enter a, b, c, d, e, and f, and displays the result. If ad – bc is 0, report that "The equation has no solution."arrow_forward(Factorials) Factorials are used frequently in probability problems. The factorial of a positive integer n (written n! and pronounced “n factorial”) is equal to the product of the positive integers from 1 to n. Write an application that calculates the factorials of 1 through 20. Use type long . Display the results in tabular format. What difficulty might prevent you from calculating the factorial of 100?arrow_forward(GREATEST COMMON DIVISOR) The greatest common divisor of integers x and y is the largest integer that evenly divides into both x and y. Write and test a recursive function gcd that returns the greatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equal to 0, then gcd (x, y) is x; otherwise, gcd (x, y) is gcd (y, x % y), where % is the remainder operator.arrow_forward
- Exercise 1: (Design of algorithm to find greatest common divisor) In mathematics, the greatest common divisor (gcd) of two or more integers is the largest positive integer that divides each of the integers. For example, the gcd of 8 and 12 is 4. Why? Divisors of 8 are 1, 2, 4, 8. Divisors of 12 are 1, 2, 4, 6, 12 Thus, the common divisors of 8 and 12 are 1, 2, 4. Out of these common divisors, the greatest one is 4. Therefore, the greatest common divisor (gcd) of 8 and 12 is 4. Write a programming code for a function FindGCD(m,n) that find the greatest common divisor. You can use any language of Java/C++/Python/Octave. Find GCD Algorithm: Step 1 Make an array to store common divisors of two integers m, n. Step 2 Check all the integers from 1 to minimun(m,n) whether they divide both m, n. If yes, add it to the array. Step 3 Return the maximum number in the array.arrow_forward(Recursive Greatest Common Divisor) The greatest common divisor of integers x and y isthe largest integer that evenly divides both x and y. Write a recursive function gcd that returns thegreatest common divisor of x and y. The gcd of x and y is defined recursively as follows: If y is equalto 0, then gcd(x, y) is x; otherwise gcd(x, y) is gcd(y, x % y), where % is the remainder operator.arrow_forward(Algebra: solve quadratic equations) The two roots of a quadratic equation ax? + bx + x = 0 can be obtained using the following formula: -b + VB - 4ac -b - Vb - 4ac and r2 2a 2a Write a function with the following header void solveQuadraticEquation(double a, double b, double c, double& discriminant, double& rl, double& r2) b² - 4ac is called the discriminant of the quadratic equation. If the discriminant is less than 0, the equation has no roots. In this case, ignore the value in rl and r2. Write a test program that prompts the user to enter values for a, b, and c and dis- plays the result based on the discriminant. If the discriminant is greater than or equal to 0, display the two roots. If the discriminant is equal to 0, display the one root. Otherwise, display "the equation has no roots". See Programming Exercise 3.1 for sample runs.arrow_forward
- (Algebra: multiply two matrices) Write a function to multiply two matrices a and b and save the result in c. Eミ9-EE9-G9 bu b12 b13 a23 X b21 b2 b23 b31 b32 b3, a12 a13 C12 C13 a21 a22 C21 C22 C23 a31 a32 a33 C31 C32 C3, The header of the function is const int N = 3; void multiplyMatrix(const double a[] [N], const double b[] [N], double c[][N]); Each element cij is a;1 × bij + an X b; + az X bzj. Write a test program that prompts the user to enter two 3 X 3 matrices and dis- plays their product. Here is a sample run:arrow_forward(Single Digit) Complete the definition of the following function:singleDigit :: Int -> Int singleDigit takes a positive integer, num, as input and returns a digit between 0 and 9 as the output. The output is computed as follows: sum all the digits in num to obtain a result; if this result is less than 10 then result is the answer; otherwise take the result and apply the same procedure (i.e. sum its digits and compute a result, and so on). Here is a sample run:*Main> singleDigit 37425 3 *Main> singleDigit 9876543 6 Here is how the above answers are computed by hand:singleDigit 37425 => 3+7+4+2+5 = 21 => 2+1 = 3 singleDigit 9876543 => 9+8+7+6+5+4+3 = 42 => 4+2 = 6arrow_forward(ABET 2) Construct a regular expression corresponding to the following set: {binary strings such that every odd position is a 1}. You may assume that the even positions can be a 0 or 1.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
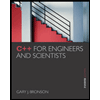
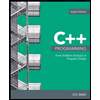