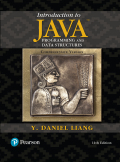
Assign grades
Program Plan:
- Import necessary packages into program.
- Define the class named “Exercise30_01”.
- Define main method.
- Define the “Scanner” object “obj” for input.
- Prompt the user and get the number of students “N” from user.
- Declare the array variable “marks[]” in type of “double”.
- Prompt and get scores using “for” loop.
- Using “DoubleStream” class assign maximum value into “best” variable.
- Declare the “grade” in type of “character”.
- Using “for” loop which is execute from “0” to “marks.length”.
- Using “if..elseif..else” condition, check the score value.
- If the value greater than “best-10”, assign “A” to “grade”.
- If the value greater than “best-20”, assign “B” to “grade”.
- If the value greater than “best-30”, assign “C” to “grade”.
- If the value greater than “best-40”, assign “D” to “grade”.
- Otherwise, assign “E” to “grade”.
- Print appropriate grade with statement on screen.
- Using “if..elseif..else” condition, check the score value.

The following JAVA code is to calculate the grade of student scores using “DoubleStream”.
Explanation of Solution
Program:
/*Include necessary packages*/
import java.util.Scanner;
//Include Stream package
import java.util.stream.DoubleStream;
//Class definition
class Exercise30_01
{
//Main method
public static void main(String[] args)
{
/*Definition of "Scanner" object*/
Scanner obj = new Scanner(System.in);
/*Prompt the user for number of students*/
System.out.print("Enter number of students: ");
//Get input from user
int N = obj.nextInt();
/*Declaration and definition of array variable*/
double[] marks = new double[N];
//Prompt the user for marks
System.out.print("Enter " + N + " scores: ");
//Loop
for (int i = 0; i < marks.length; i++)
{
/*Get scores and store into "marks[]" variable*/
marks[i] = obj.nextDouble();
}
/*Get maximum value using stream*/
double best = DoubleStream.of(marks).max().getAsDouble();
//Declaration of variable
char grade;
//Loop
for (int i = 0; i < marks.length; i++)
{
/*Condition to check marks*/
if (marks[i] >= best - 10)
//Assign grade
grade = 'A';
/*Condition to check marks*/
else if (marks[i] >= best - 20)
//Assign grade
grade = 'B';
/*Condition to check marks*/
else if (marks[i] >= best - 30)
//Assign grade
grade = 'C';
/*Condition to check marks*/
else if (marks[i] >= best - 40)
//Assign grade
grade = 'D';
//Else statement
else
//Assign grade
grade = 'F';
//Print statement
System.out.println("Student " + i + " score is " +marks[i] + " and grade is " + grade);
}
}
}
Enter number of students: 4
Enter 4 scores: 40 55 70 58
Student 0 score is 40.0 and grade is C
Student 1 score is 55.0 and grade is B
Student 2 score is 70.0 and grade is A
Student 3 score is 58.0 and grade is B
Want to see more full solutions like this?
Chapter 30 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
- If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? Order, Product, store, customer.arrow_forwardInheritance & Polymorphism (Ch11) There are 6 classes including Person, Student, Employee, Faculty, and Staff. 4. Problem Description: • • Design a class named Person and its two subclasses named student and Employee. • Make Faculty and Staff subclasses of Employee. • A person has a name, address, phone number, and e-mail address. • • • A person has a class status (freshman, sophomore, junior and senior). Define the status as a constant. An employee has an office, salary, and date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString() method in each class to display the class name and the person's name. 4-1. Explain on how you would code this program. (1 point) 4-2. Implement the program. (2 point) 4-3. Explain your code. (2 point)arrow_forward
- Suppose you buy an electronic device that you operate continuously. The device costs you $300 and carries a one-year warranty. The warranty states that if the device fails during its first year of use, you get a new device for no cost, and this new device carries exactly the same warranty. However, if it fails after the first year of use, the warranty is of no value. You plan to use this device for the next six years. Therefore, any time the device fails outside its warranty period, you will pay $300 for another device of the same kind. (We assume the price does not increase during the six-year period.) The time until failure for a device is gamma distributed with parameters α = 2 and β = 0.5. (This implies a mean of one year.) Use @RISK to simulate the six-year period. Include as outputs (1) your total cost, (2) the number of failures during the warranty period, and (3) the number of devices you own during the six-year period. Your expected total cost to the nearest $100 is _________,…arrow_forwardWhich one of the 4 Entities mention in the diagram can have a recursive relationship? If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)?arrow_forwardPlease answer the JAVA OOP Programming Assignment scenario below: Patriot Ships is a new cruise line company which has a fleet of 10 cruise ships, each with a capacity of 300 passengers. To manage its operations efficiently, the company is looking for a program that can help track its fleet, manage bookings, and calculate revenue for each cruise. Each cruise is tracked by a Cruise Identifier (must be 5 characters long), cruise route (e.g. Miami to Nassau), and ticket price. The program should also track how many tickets have been sold for each cruise. Create an object-oriented solution with a menu that allows a user to select one of the following options: 1. Create Cruise – This option allows a user to create a new cruise by entering all necessary details (Cruise ID, route, ticket price). If the maximum number of cruises has already been created, display an error message. 2. Search Cruise – This option allows to search a cruise by the user provided cruise ID. 3. Remove Cruise – This op…arrow_forward
- I need to know about the use and configuration of files and folders, and their attributes in Windows Server 2019.arrow_forwardSouthern Airline has 15 daily flights from Miami to New York. Each flight requires two pilots. Flights that do not have two pilots are canceled (passengers are transferred to other airlines). The average profit per flight is $6000. Because pilots get sick from time to time, the airline is considering a policy of keeping four *reserve pilots on standby to replace sick pilots. Such pilots would introduce an additional cost of $1800 per reserve pilot (whether they fly or not). The pilots on each flight are distinct and the likelihood of any pilot getting sick is independent of the likelihood of any other pilot getting sick. Southern believes that the probability of any given pilot getting sick is 0.15. A) Run a simulation of this situation with at least 1000 iterations and report the following for the present policy (no reserve pilots) and the proposed policy (four reserve pilots): The average daily utilization of the aircraft (percentage of total flights that fly) The…arrow_forwardWhy is JAVA OOP is really difficult to study?arrow_forward
- My daughter is a Girl Scout and it is time for our cookie sales. There are 15 neighbors nearby and she plans to visit every neighbor this evening. There is a 40% likelihood that someone will be home. If someone is home, there is an 85% likelihood that person will make a purchase. If a purchase is made, the revenue generated from the sale follows the Normal distribution with mean $18 and standard deviation $5. Using @RISK, simulate our door-to-door sales using at least 1000 iterations and report the expected revenue, the maximum revenue, and the average number of purchasers. What is the probability that the revenue will be greater than $120?arrow_forwardQ4 For the network of Fig. 1.41: a- Determine re b- Find Aymid =VolVi =Vo/Vi c- Calculate Zi. d- Find Ay smid e-Determine fL, JLC, and fLE f-Determine the low cutoff frequency. g- Sketch the asymptotes of the Bode plot defined by the cutoff frequencies of part (e). h-Sketch the low-frequency response for the amplifier using the results of part (f). Ans: 28.48 2, -72.91, 2.455 KS2, -54.68, 103.4 Hz. 38.05 Hz. 235.79 Hz. 235.79 Hz. 14V 15.6ΚΩ 68kQ 0.47µF Vo 0.82 ΚΩ V₁ B-120 3.3kQ 0.47µF 10kQ 1.2k0 =20µF Z₁ Fig. 1.41 Circuit forarrow_forwarda. [10 pts] Write a Boolean equation in sum-of-products canonical form for the truth table shown below: A B C Y 0 0 0 1 0 0 1 0 0 1 0 0 0 1 1 0 1 0 0 1 1 0 1 0 1 1 1 1 0 1 1 0 a. [10 pts] Minimize the Boolean equation you obtained in (a). b. [10 pts] Implement, using Logisim, the simplified logic circuit. Include an image of the circuit in your report.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
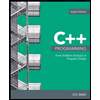
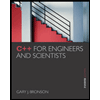
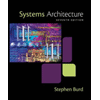