Concept explainers
Trace the following
A) (Assume the user enters 39000. Use a calculator.)
double salary, monthly;
cout << "What is your annual salary?";
cin >> salary;
monthly= static_cast<int>(salary) / 12;
cout << "Your monthly wages are"<< monthly<< endl;
B) unsigned int x, y, z;
X = y = Z = 4;
X += 2;
y −= 1;
z *= 3;
cout << x <<" "<< y <<" "<< z << endl;

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Database Concepts (7th Edition)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Problem Solving with C++ (9th Edition)
Starting out with Visual C# (4th Edition)
Modern Database Management (12th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- #include<stdio.h>#include<stdlib.h> int cent50=0;int cent20=0;int cent10=0;int cent05=0; void calculatechange(int* change){if(*change>0){if(*change>=50){*change-=50;cent50++;}else if(*change>=20){*change-=20;cent20++;}else if(*change>=10){*change-=10;cent10++;}else if(*change>=05){*change-=05;cent05++;}calculatechange(change);}}void printchange(){if(cent50)printf("\n50cents:%d coins",cent50);if(cent20)printf("\n20cents:%d coins",cent20);if(cent10)printf("\n10cents:%d coins",cent10);if(cent05)printf("\n05cents:%d coins",cent05);cent50=0;cent20=0;cent10=0;cent05=0;}void takechange(int* change){scanf("%d",change);getchar();}int main(){int change=0;int firstinput=0;while(1){if(!firstinput){printf("\nEnter the amount:");firstinput++;}else{printf("\n\nEnter the amount to continue or Enter -1 to…arrow_forwardConvert totalMeters to hectometers, decameters, and meters, finding the maximum number of hectometers, then decameters, then meters. Ex: If the input is 815, then the output is: Hectometers: 8 Decameters: 1 Meters: 5 Note: A hectometer is 100 meters. A decameter is 10 meters. 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 15 16 17 18 int totalMeters; int numHectometers; int numDecameters; int numMeters; cin >>totalMeters; cout << "Hectometers: cout << "Decameters: cout << "Meters: " << numMeters << endl; " << numHectometers << endl; << numDecameters << endl; 2 3arrow_forwardComputer Fundamentals and Programming 2 Write a program that determines a student’s grade. The program will accept 3 scores and computes the average score. Determine the grade based on the following rules: - If the average score is equal or greater than 90, the grade is A. - If the average score is greater than or equal to 70 and less than 90, the grade is B. - If the average score is greater than or equal to 50 and less than 70, the grade is C. - If the average score is less than 50, the grade is F. Source Codes and Print Screen of the Outputarrow_forward
- be recor #include #include minutes #include limit on int func(int, int, int, int); main(){ srand(time(NULL)); int a, b, c, fNum; printf("Choose three different numbers between 0-39:"); scanf ("%d%d%d", &a, &b, &c); fNum = func (a, b, c, 25); printf("\nThe result: %d", fNum); } int func (int ul, int u2, int u3, int iter){ srand (time (NULL)); int n1=0, i=0, count=0; for (;iarrow_forwardTranscribed Image Text Write a program that asks for the principal, the annual interest rate, and the number of times the interest is compounded. It should display a report similar to the following: Interest Rate: 4.25% Times Compounded: 12 Principal: $ 1000.00 Interest: 43.33 Final balance: $ 1043.33arrow_forwardJAVA:arrow_forwardProgramming Assignment: Car Repair For this programming assignment, create a car repair program that prompts the user to enter information about a customer, the customer's vehicle, and the work performed on the customer's vehicle. Your program should then display the final car repair bill on the computer screen. Your program should accomplish the following: 1. Prompt the user to enter the following information: a. Information about the customer (name, street address, city, state, zip code, phone number). b. Information about the customer's vehicle (make, model, year). c. Information about the work performed (brief description, labor hours needed, cost of parts). 2. Use a labor cost amount of $100.00 per hour and a sales tax rate of 7.0%. 3. Calculate the following: Variable subtotal sales tax total repair bill amount Formula subtotal = labor cost per hour * number of labor hours sales tax = sales tax rate * subtotal total repair bill amount = subtotal + sales tax 4. A screen shot of a…arrow_forwardStatic Variable: a variable whose lifetime is the lifetime of the program (static int x;) Dynamic Variable: It is a pointer to a variable (int *x;) Is this comparison true?arrow_forwardchange normal body temperature to 36.5–37.5 °C #include<iostream>using namespace std;int main(){ float temp;cout<<"Enter the body temperature in Celsius\n";cin>>temp;if (temp>=37){cout<<"The quarantine of the patient is required\n";cout<<"Enter the name of the patient\n";string patient;cin>>patient;cout<<"Where the passenger is from\n";string place;cin>>place;cout<<patient<<" from "<<place<<"is to be quarantined at Hospital"; }cout<<"Normal temperature\n";}arrow_forwardAssignment Content Write a program that lets the user enter the coordinates of the center and radius of two circles, circle 1 with center coordinates at (* Y1 and circle 2 with center coordinates at (X y,). The program then determines whether circle 2 is inside circle 1 or overlaps with circle 1. rl rl • (x1, y1) (x1, y1) (x2, y2), (x2, y2) Circle 2 is found to be inside circle 1 if distance between the centers is <=Tradius circle1 - radius cirede and circle 2 is said to overlap circle one if the distance between the two centers is <=radius circle1 + radius circle2- Test Cases: Enter center coordinates (x, y) and radius of circle 1:0.5 5.1 13 Enter center coordinates (x, y) and radius of circle 2:11.7 4.5 Circle 2 is inside of circle 1 Enter center coordinates (x, y) and radius of circle 1:3 75.5 Enter center coordinates (x, y) and radius of circle 2: 6.7 3.5 3 Circle 2 overlaps circle 1 Enter center coordinates (x, y) and radius of circle 1:3.55.5 1 Enter center coordinates (x, y) and…arrow_forwardC++ Visual 2019 A particular talent competition has five judges, each of whom awards a score between 0 and 10 to each performer. Fractional scores, such as 8.3, are allowed. A performer's final score is determined by dropping the highest and lowest score received, then averaging the three remaining scores. Write a program that uses this method to calculate a contestant's score. It should include the following functions: void getJudgeData() should ask the user for a judge's score, store it in a reference parameter variable, and validate it. This function should be called by main once for each of the five judges. void calcScore() should calculate and display the average of the three scores that remain after dropping the highest and lowest scores the performer received. This function should be called just once by main and should be passed the five scores. The last two functions, described below, should be called by calcScore, which uses the returned information to determine which of the…arrow_forwardPersonal BestWrite a program that asks for the name of a pole vaulter and the dates and vaultheights (in meters) of the athlete’s three best vaults. It should then report, in order ofheight (best first), the date on which each vault was made and its height.Input Validation: Only accept values between 2.0 and 5.0 for the heights.arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
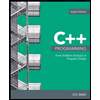