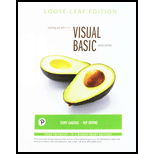
Joe's Pizza Palace needs an application to calculate the number of slices a pizza of any size can be divided into. The application should do the following:
- a. Allow the user to enter the diameter of the pizza, in inches.
- b. Calculate the number of slices that can be cut from a pizza that size.
- c. Display a message that indicates the number of slices.
To calculate the number of slices that can be cut from the pizza, you must know the following facts:
- a. Each slice should have an area of 14.125 inches.
- b. To calculate the number of slices, divide the area of the pizza by 14.125.
The area of the pizza is calculated with the following formula:
Area = πr2
Note
π is the Greek letter pi. 3.14159 can be used as its value. The variable r is the radius of the pizza. Divide the diameter by 2 to get the radius.
If the user fails to enter numeric values, display an appropriate error message and do not attempt to perform calculations. Use the following test data to determine if the application is calculating properly:

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Starting Out With Visual Basic, Student Value Edition (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects
Starting Out with Python (3rd Edition)
Experiencing MIS
Artificial Intelligence: A Modern Approach
Computer Systems: A Programmer's Perspective (3rd Edition)
- Account numbers sometimes contain a check digit that is the result of a mathematical calculation. The inclusion of the digit in an account number helps ascertain whether the number is a valid one. Write an application named CheckDigit that asks a user to enter a four-digit account number and determines whether it is a valid number. The number is valid if the fourth digit is the remainder when the number represented by the first three digits of the four-digit number is divided by 7. For example, 7770 is valid, because 0 is the remainder when 777 is divided by 7. The next problems rely on the generation of a random number. You can create a random number that is at least mi n but less than max using the following statements: Random ranNumberCenerator = new Random(); int randomNumber; randomNumber = ranNumberGenerator .Next(min, max);arrow_forwardPercentage of vehicles: Write an interactive program that asks the users to input the number of cars, trucks and suv that entered a parking lot. Your progam should then calculate the percetages of each vehicle type and display the result.arrow_forwardWrite the pseudocode for an application that will request three numbers from a user. The application should then determine and display the largest of the three numbers.arrow_forward
- Python programming language You will be creating an application to calculate the maximum amount of contribution a person can make to a Roth IRA based on their age and income. Over 50 years old and your contribution limit goes up from $6000 to $7000 dollars. However, if the person is married and the combined household income is over $206,000 a year, or a single person with income over $139,000, you are not allowed to contribute. Write an application that asks the user their age and their income. Using this information use a nested if statement to calculate the maximum allowable contribution.arrow_forwardA retail company must file a monthly sales tax report listing the total sales for the month and the amount of state and county sales tax collected. The state sales tax rate is 4 percent and the county sales tax rate is 2 percent. Write a program that asks the user to enter the total sales for the month. The application should calculate and display the following: The amount of county sales tax The amount of state sales tax The total sales tax (county plus state) Step 3: Complete the pseudocode by writing the missing lines. (Reference: Defining and Calling a Module, page 78-81). Also, when writing your modules and making calls, be sure to pass necessary variables as arguments and accept them as reference parameters if they need to be modified in the module. (Reference: Passing Arguments by Value and by Reference, page 97 – 103). Module main () //Declare local variables Declare Real totalSales…arrow_forwardA retail company must file a monthly sales tax report listing the total sales for the month and the amount of state and county sales tax collected. The state sales tax rate is 4 percent and the county sales tax rate is 2 percent. Write a program that asks the user to enter the total sales for the month. The application should calculate and display the following: The amount of county sales tax The amount of state sales tax The total sales tax (county plus state) Step 2: Given the major task involved in this program, what modules might you consider including? Describe the purpose of each module. (Reference: Defining and Calling a Module, page 78). Module Name Purpose Module inputData () Allows the user to enter required user inputarrow_forward
- Random Number Guessing Game Create an application that generates a random number in the range of 1 through 100 and asks the user to guess what the number is. If the user’s guess is higher than the random number, the program should display “Too high, try again.” If the user’s guess is lower than the random number, the program should display “Too low, try again.” If the user guesses the number, the application should congratulate the user and then generate a new random number so the game can start over. Optional Enhancement: Enhance the game so it keeps count of the number of guesses that the user makes. When the user correctly guesses the random number, the program should display the number of guesses.arrow_forwardGeometry Calculator Write a program that displays the following menu: Geometry Calculator 1. Calculate the Area of a Circle 2. Calculate the Area of a Rectangle 3. Calculate the Area of a Triangle 4. Quit Enter your choice (1-4): If the user enters 1, the program should ask for the radius of the circle and then display its area. Use the following formula: area = Pi*r^2 Use 3.14159 for and the radius of the circle for r. If the user enters 2, the program should ask for the length and width of the rectangle and then display the rectangle’s area. Use the following formula: area = length * width If the user enters 3 the program should ask for the length of the triangle’s base and its height, and then display its area. Use the following formula: area = base * height * .5 If the user enters 4, the program should end. Input Validation: Display an error message if the user enters a number outside the range of 1 through 4 when selecting an item from the menu. Do not accept negative values for…arrow_forward7. SOFTWARE SALES A software company sells a package that retails for $99. Quantity discounts are given according to the following table: Quantity 10-19 20-49 50-99 100 or more Discount 20 % 30 % 40 % 50% Create an application that lets the user enter the number of packages purchased. The program should then display the amount of the discount (if any) and the total amount of the purchase after the discount.arrow_forward
- # CODE _ 09.arrow_forwardAn online book club awards points to its customers based on the number of bookspurchased each month . Points are awarded as follows:Books Purchased01234 or morePoints Earned051530soWrite a program that asks the user to enter the number of books purchased this monthand then displays the number of points awarded .arrow_forwardTHIS SHOULD BE WRITTEN IN VISUAL C# FORMS: Create an application that lets the user know how many Pounds he/she will have when they get to England based on the number of dollars they have now. Also do the reverse. Calculate how many Dollars they will have when they return based on the number of Pounds they have when in England. Do the same for travelers going to Europe. Convert Dollars to Euro and Euro to Dollars. Program should allow you to enter the customer name, destination, money converted from and money converted to. After each customer transaction the name, destination, and both money amounts should be stored on a history file. Because the exchange rate changes daily you want a system that will easily adapt to the change. Your program needs to read text file (notepad) that will contain the conversion rates. That way each morning when you get to work you can put the new rates in the text file. Your program will read the text file as soon as you turn it on (Form_Load) and…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
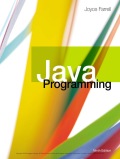
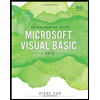
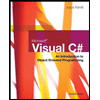
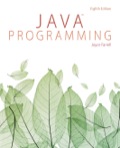