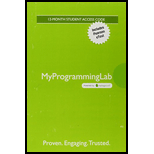
Concept explainers
MyMap using Open Addressing with Linear Probing
Program Plan:
- Define a class named “Sample” to implement MyMap using open addressing with linear probing.
- Define function main.
- Define an integer HashMap named “map”.
- Put ‘2, 2” into the map.
- Remove “2” from the map.
- Define a static class named “MyHashMap” and implement “MyMap”.
- Define required variables.
- Define a function named “MyHashMap”.
- Construct a map with the default capacity and load factor.
- Define a function named “MyHashMap” with one parameter.
- Construct a map with the specific initial capacity and default load factor.
- Define a function named “MyHashMap” with two parameters.
- Construct a map with the default capacity and load factor.
- Define a function named “clear”.
- Remove all of the entries from this map.
- Define a function named “containsKey”.
- Return true if the specified key is in the map.
- Define a function named “containsValue”.
- Return true if this map contains the specific value.
- Define a function named “entrySet”.
- Return a set of entries in the map.
- Define a function named “get”.
- Return the first value that matches the specific key.
- Define a function named “getAll”.
- Return all values for the specified key in this map.
- Define a function named “isEmpty”.
- Return true if this map contains no entries.
- Define a function named “keyset”.
- Return a set consisting of the keys in this map.
- Define a function named “put”.
- Add an entry (key, value) into the map and return the value.
- Define a function named “remove”.
- Remove the element for the specific key.
- Define a function named “size”.
- Return the number of mappings in this map.
- Define a function named “values”.
- Return a set consisting of the values in this map.
- Define a function named “hash”.
- Return “hashCode % capacity” value.
- Define a function named “supplmentHash”.
- Ensure the hashing is evenly distributed and return the result.
- Define a function named “removeEntries”.
- Remove all entries from each bucket.
- Define a function named “rehash”.
- Rehash this map.
- Define a function named “toString”.
- Return a string representing for this map.
- Define an interface name “MyMap”.
- Declare function prototypes for the functions which are created at the class “MyHashMap”.
- Define function main.

The below program creates a new concrete class that implements MyMap using open addressing with linear probing, whose hash table size is 4 and the table size get doubled when the load factor exceeds the threshold 0.5.
Explanation of Solution
Program:
public class Sample {
/** Main method */
public static void main(String[] args) {
MyHashMap<Integer, Integer> map = new MyHashMap<>();
map.put(2, 2);
System.out.println("Is key 2 in the map? " + map.containsKey(2));
map.remove(2);
System.out.println("Is key 2 in the map? " + map.containsKey(2));
}
static class MyHashMap<K, V> implements MyMap<K, V> {
// Define the default hash table size.
private static int INITIAL_CAPACITY = 4;
// Define the maximum hash table size. 1 << 30 is same as 2^30
private static int MAX_CAPACITY = 1 << 30;
// Current hash table capacity.
private int capacity;
// Define default load factor
private static float MAX_LOAD_FACTOR = 0.5f;
// Specify a load factor used in the hash table
private float loadFactorThreshold;
// The number of entries in the map
private int size = 0;
// Hash table is an array with each cell that is a linked list
MyMap.Entry<K, V>[] table;
/** Construct a map with the default capacity and load factor */
public MyHashMap() {
this(INITIAL_CAPACITY, MAX_LOAD_FACTOR);
}
/**
* Construct a map with the specified initial capacity and default load
* factor
*/
public MyHashMap(int initialCapacity) {
this(initialCapacity, MAX_LOAD_FACTOR);
}
/**
* Construct a map with the specified initial capacity and load factor
*/
public MyHashMap(int initialCapacity, float loadFactorThreshold) {
this.capacity = initialCapacity;
this.loadFactorThreshold = loadFactorThreshold;
table = new MyMap.Entry[capacity];
}
/** Remove all of the entries from this map */
public void clear() {
size = 0;
removeEntries();
}
/** Return true if the specified key is in the map */
public boolean containsKey(K key) {
if (get(key) != null)
return true;
else
return false;
}
/** Return true if this map contains the specified value */
public boolean containsValue(V value) {
for (int i = 0; i < table.length; i++)
if (table[i] != null && table[i].value.equals(value))
return true;
return false;
}
/** Return a set of entries in the map */
public java.util.Set<MyMap.Entry<K, V>> entrySet() {
java.util.Set<MyMap.Entry<K, V>> set = new java.util.HashSet<MyMap.Entry<K, V>>();
for (int i = 0; i < capacity; i++)
if (table[i] != null)
set.add(table[i]);
return set;
}
/** Return the first value that matches the specified key */
public V get(K key) {
// Perform linear probing
int i = hash(key.hashCode());
while (table[i] != null) {
if (table[i].key != null && table[i].key.equals(key))
return table[i].value;
i = (i + 1) % table.length;
}
return null;
}
/** Return all values for the specified key in this map */
public java.util.Set<V> getAll(K key) {
java.util.Set<V> set = new java.util.HashSet<V>();
for (int i = 0; i < capacity; i++)
if (table[i] != null && table[i].key.equals(key))
set.add(table[i].value);
return set;
}
/** Return true if this map contains no entries */
public boolean isEmpty() {
return size == 0;
}
/** Return a set consisting of the keys in this map */
public java.util.Set<K> keySet() {
java.util.Set<K> set = new java.util.HashSet<K>();
for (int i = 0; i < capacity; i++)
if (table[i] != null)
set.add(table[i].key);
return set;
}
/** Add an entry (key, value) into the map */
public V put(K key, V value) {
if (size >= capacity * loadFactorThreshold) {
if (capacity == MAX_CAPACITY)
throw new RuntimeException("Exceeding maximum capacity");
rehash();
}
int i = hash(key.hashCode());
while (table[i] != null && table[i].key != null)
i = (i + 1) % table.length;
// Add an entry (key, value) to the table
table[i] = new MyMap.Entry<K, V>(key, value);
size++; // Increase size
return value;
}
/** Remove the element for the specified key */
public void remove(K key) {
int i = hash(key.hashCode());
while (table[i] != null
&& (table[i].key == null || !table[i].key.equals(key)))
i = (i + 1) % table.length;
if (table[i] != null && table[i].key.equals(key)) {
// A special marker Entry(null, null) is placed for the deleted
// entry
table[i] = new MyMap.Entry<K, V>(null, null);
size--;
}
}
/** Return the number of mappings in this map */
public int size() {
return size;
}
/** Return a set consisting of the values in this map */
public java.util.Set<V> values() {
java.util.Set<V> set = new java.util.HashSet<V>();
for (int i = 0; i < capacity; i++)
if (table[i] != null)
set.add(table[i].value);
return set;
}
/** Hash function */
private int hash(int hashCode) {
return hashCode % capacity;
// return supplementHash(hashCode) & (capacity - 1);
}
/** Ensure the hashing is evenly distributed */
private static int supplementHash(int h) {
h ^= (h >>> 20) ^ (h >>> 12);
return h ^ (h >>> 7) ^ (h >>> 4);
}
/** Remove all entries from each bucket */
private void removeEntries() {
for (int i = 0; i < capacity; i++)
table[i] = null;
}
/** Rehash the map */
private void rehash() {
java.util.Set<Entry<K, V>> set = entrySet(); // Get entries
capacity <<= 1; // Double capacity
table = new Entry[capacity]; // Create a new hash table
size = 0; // Clear size
for (Entry<K, V> entry : set) {
put(entry.getKey(), entry.getValue()); // Store to new table
}
}
@Override
/** Return a string representation for this map */
public String toString() {
StringBuilder builder = new StringBuilder("[");
for (int i = 0; i < capacity; i++) {
if (table[i] != null && table[i].key != null)
builder.append(table[i].toString());
}
return builder.append("]").toString();
}
}
interface MyMap<K, V> {
/** Remove all of the entries from this map */
public void clear();
/** Return true if the specified key is in the map */
public boolean containsKey(K key);
/** Return true if this map contains the specified value */
public boolean containsValue(V value);
/** Return a set of entries in the map */
public java.util.Set<Entry<K, V>> entrySet();
/** Return the first value that matches the specified key */
public V get(K key);
/** Return all values for the specified key in this map */
public java.util.Set<V> getAll(K key);
/** Return true if this map contains no entries */
public boolean isEmpty();
/** Return a set consisting of the keys in this map */
public java.util.Set<K> keySet();
/** Add an entry (key, value) into the map */
public V put(K key, V value);
/** Remove the entries for the specified key */
public void remove(K key);
/** Return the number of mappings in this map */
public int size();
/** Return a set consisting of the values in this map */
public java.util.Set<V> values();
/** Define inner class for Entry */
public static class Entry<K, V> {
K key;
V value;
public Entry(K key, V value) {
this.key = key;
this.value = value;
}
public K getKey() {
return key;
}
public V getValue() {
return value;
}
@Override
public String toString() {
return "[" + key + ", " + value + "]";
}
}
}
}
Is key 2 in the map? true
Is key 2 in the map? false
Want to see more full solutions like this?
Chapter 27 Solutions
MyLab Programming with Pearson eText -- Access Card -- for Introduction to Java Programming and Data Structures, Comprehensive Version
- you can select multipy optionsarrow_forwardFor each of the following, decide whether the claim is True or False and select the True ones: Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would mean that all problems in NP can also be solved in cubic time. If a problem can be solved using Dynamic Programming, then it is not NP-complete. Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time reduction from X to Y and also one from Y to X.arrow_forwardMaximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forward
- Please help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forwardWhat does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forwardPlease assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forward
- need help with a html code and css code that will match this image.arrow_forwardneed help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forward
- Why all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forwardWhy is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forwardWrite the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
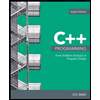
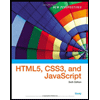
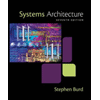
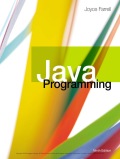