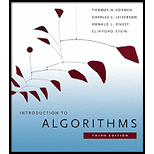
Introduction to Algorithms
3rd Edition
ISBN: 9780262033848
Author: Thomas H. Cormen, Ronald L. Rivest, Charles E. Leiserson, Clifford Stein
Publisher: MIT Press
expand_more
expand_more
format_list_bulleted
Question
Chapter 22.3, Problem 1E
Program Plan Intro
To make chart of
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Hands-On Assignments Part II
Assignment 1-5: Querying the DoGood Donor Database
Review the DoGood Donor data by writing and running SQL statements to perform the following
tasks:
1. List each donor who has made a pledge and indicated a single lump sum payment. Include
first name, last name, pledge date, and pledge amount.
2. List each donor who has made a pledge and indicated monthly payments over one year.
Include first name, last name, pledge date, and pledge amount. Also, display the monthly
payment amount. (Equal monthly payments are made for all pledges paid in monthly
payments.)
3. Display an unduplicated list of projects (ID and name) that have pledges committed. Don't
display all projects defined; list only those that have pledges assigned.
4. Display the number of pledges made by each donor. Include the donor ID, first name, last
name, and number of pledges.
5. Display all pledges made before March 8, 2012. Include all column data from the
DD PLEDGE table.
Write a FancyCar class to support basic operations such as drive, add gas, honk horn, and start engine. FancyCar.java is provided with method stubs. Follow each step to gradually complete all methods.
Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main() method includes basic method calls. Add statements in main() as methods are completed to support development mode testing.
Step 0. Declare private fields for miles driven as shown on the odometer (int), gallons of gas in tank (double), miles per gallon or MPG (double), driving capacity (double), and car model (String). Note the provided final variable indicates the gas tank capacity of 14.0 gallons.
Step 1 (2 pts). 1) Complete the default constructor by initializing the odometer to five miles, tank is full of gas, miles per gallon is 24.0, and the model is "Old Clunker". 2)…
Find the error:
daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0]
days_of_week = ['Sunday', 'Monday', 'Tuesday',
'Wednesday', 'Thursday', 'Friday',
'Saturday']
for i in range(7):
daily_sales[i] = float(input('Enter the sales for ' \
+ day_of_week[i] + ': ')
Chapter 22 Solutions
Introduction to Algorithms
Ch. 22.1 - Prob. 1ECh. 22.1 - Prob. 2ECh. 22.1 - Prob. 3ECh. 22.1 - Prob. 4ECh. 22.1 - Prob. 5ECh. 22.1 - Prob. 6ECh. 22.1 - Prob. 7ECh. 22.1 - Prob. 8ECh. 22.2 - Prob. 1ECh. 22.2 - Prob. 2E
Ch. 22.2 - Prob. 3ECh. 22.2 - Prob. 4ECh. 22.2 - Prob. 5ECh. 22.2 - Prob. 6ECh. 22.2 - Prob. 7ECh. 22.2 - Prob. 8ECh. 22.2 - Prob. 9ECh. 22.3 - Prob. 1ECh. 22.3 - Prob. 2ECh. 22.3 - Prob. 3ECh. 22.3 - Prob. 4ECh. 22.3 - Prob. 5ECh. 22.3 - Prob. 6ECh. 22.3 - Prob. 7ECh. 22.3 - Prob. 8ECh. 22.3 - Prob. 9ECh. 22.3 - Prob. 10ECh. 22.3 - Prob. 11ECh. 22.3 - Prob. 12ECh. 22.3 - Prob. 13ECh. 22.4 - Prob. 1ECh. 22.4 - Prob. 2ECh. 22.4 - Prob. 3ECh. 22.4 - Prob. 4ECh. 22.4 - Prob. 5ECh. 22.5 - Prob. 1ECh. 22.5 - Prob. 2ECh. 22.5 - Prob. 3ECh. 22.5 - Prob. 4ECh. 22.5 - Prob. 5ECh. 22.5 - Prob. 6ECh. 22.5 - Prob. 7ECh. 22 - Prob. 1PCh. 22 - Prob. 2PCh. 22 - Prob. 3PCh. 22 - Prob. 4P
Knowledge Booster
Similar questions
- Find the error: daily_sales = [0.0, 0,0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(7): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': ')arrow_forwardFind the error: daily_sales = [0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0] days_of_week = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'] for i in range(6): daily_sales[i] = float(input('Enter the sales for ' \ + days_of_week[i] + ': '))arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it and normalize it? Give two references with your answer.arrow_forward
- What are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Consider that the database offline is not allowed since people are connected to it and personal data might be bridged and not secured. Provide three refernces with you answer.arrow_forwardShould software manufacturers should be tolerant of the practice of software piracy in third-world countries to allow these countries an opportunity to move more quickly into the information age? Why or why not?arrow_forwardI would like to know about the features of Advanced Threat Protection (ATP), AMD-V, and domain name space (DNS).arrow_forward
- I need help to resolve the following activityarrow_forwardModern life has been impacted immensely by computers. Computers have penetrated every aspect of oursociety, either for better or for worse. From supermarket scanners calculating our shopping transactionswhile keeping store inventory; robots that handle highly specialized tasks or even simple human tasks,computers do much more than just computing. But where did all this technology come from and whereis it heading? Does the future look promising or should we worry about computers taking over theworld? Or are they just a necessary evil? Provide three references with your answer.arrow_forwardWhat are the steps you will follow in order to check the database and fix any problems with it? Have in mind that you SHOULD normalize it as well. Describe in full, consider the following:• Taking the database offline is not allowed since people are connected to it.• Personal data might be bridged and not secured. Provide three refernces with you answerarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningPrinciples of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage Learning
- Operations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeFundamentals of Information SystemsComputer ScienceISBN:9781305082168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
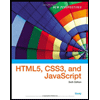
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
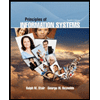
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
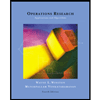
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole
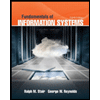
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
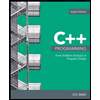
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning