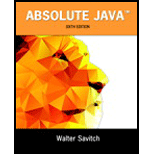
Continue with the object keyboard from Self-Test Exercise 16. Consider the
following input:
What values will the following code assign to the variables word1 and word2?

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Absolute Java (6th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Python (4th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (8th Edition)
- Write an application that determines whether a phrase entered by the user is a palindrome. A palindrome is a phrase that reads the same backward and forward without regarding capitalization, spaces or punctuation. And it will allow the user to enter as many phrases as he likes after each iteration.arrow_forwardJAVAA7-Write a class with a constructor that accepts a String object as its argument. The class should have a method that returns the number of vowels in the string, and another method that returns the number of consonants in the string. Demonstrate the class in a program by invoking the methods that return the number of vowels and consonants. Print the counts returned.arrow_forwardComputer programmin Javaarrow_forward
- In some circumstances, a class is reliant on itself. That is, one class's object interacts with another class's object. To accomplish this, a method of the class may accept an object of the same class as a parameter.The String class's concat function is an example of this situation. The method is called by one String object and receives another String object as an argument. Here's an illustration:str3 is equal to str1.concat(str2);The method's String object (str1) appends its characters to those of the String supplied as a parameter (str2). As a consequence, a new String object is returned and saved as str3. Create Java code to implement the conditions given.arrow_forwardstr is a String object.Write Java statements that finds the number of the white-space characters in str.Write only the Java statements that performs the above task, nothing else.arrow_forwardDescriptionA researcher is analyzing DNA. A DNA can be represented as a string composed of the characters A, G, C, or T.One day, researchers found a strange DNA, which is Smooth Repeated DNA. The DNA is represented by a string that has infinite length. The string has a repeating pattern, i.e. the DNA string 0 is repeated an infinite number of times. For example, if0 = "????", then = "???????????? . . . ".According to researchers, a DNA is said to be special if it contains substrings . Determine whetheris a substring of . Squad FormatA line containing the two strings 0 and . Output FormatA line that determines whether it is a substring of . Issue “YES” ifis a substring of . Output “NO” otherwise. Example Input and Output Input Example Example Output AGCT GC YES AGCT TA YES AGCT GT No AGCT TAGCTAGCT YES AGGACCTA CTAA YES Explanation ExampleIn the first to fourth test case examples, is worth "???????????? . . . ". The part in bold is one of the…arrow_forward
- Lab Task 04: Include the methods in the file KMP_Implementation.txt in a complete Java program that prompts for and reads a text string and then a text pattern to search for in the text. It then displays the result of the search. Sample program runs: Enter the text: ABABСАВАВАВСАВАВСАВАВСАВАВКККАВАВСАВАВ Enter the pattern to search for: ABABCABAB Pattern found at these text starting indexes: 0 Enter the text: A KFUPM STUDENT Enter the pattern to search for: KFE Pattern not in text. 7 12 17 29arrow_forwardIn the Raptor program Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.)• If both players make the same choice, the…arrow_forwardIn the Raptor program Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.)• If both players make the same choice, the…arrow_forward
- In the Raptor program Write a program that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is 2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Don’t display the computer’s choice yet.)2. The user enters his or her choice of “rock,” “paper,” or “scissors” at the keyboard.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses paper and the other player chooses rock, then paper wins. (Paper wraps rock.)• If both players make the same choice, the…arrow_forwardPalindromes - “A palindrome” is a string that reads the same from both directions. For example: the word "mom" is a palindrome. Also, the string "Murder for a jar of red rum" is a palindrome. - So, you need to implement a Boolean function that takes as input a string and its return is true (1) in case the string is a palindrome and false (0) otherwise. - There are many ways to detect if a phrase is a palindrome. The method that you will implement in this task is by using two stacks. This works as follows. Push the left half of the characters to one stack (from left to right) and push the second half of the characters (from right to left) to another stack. Pop from both stacks and return false if at any time the two popped characters are different. Otherwise, you return true after comparing all the elements. Phrases of odd length have to be treated by skipping the middle element like the word "mom", your halves are "m" and "m". - Hint: (without using STL)arrow_forwardPalindromes - “A palindrome” is a string that reads the same from both directions. For example: the word "mom" is a palindrome. Also, the string "Murder for a jar of red rum" is a palindrome. - So, you need to implement a Boolean function that takes as input a string and its return is true (1) in case the string is a palindrome and false (0) otherwise. - There are many ways to detect if a phrase is a palindrome. The method that you will implement in this task is by using two stacks. This works as follows. Push the left half of the characters to one stack (from left to right) and push the second half of the characters (from right to left) to another stack. Pop from both stacks and return false if at any time the two popped characters are different. Otherwise, you return true after comparing all the elements. Phrases of odd length have to be treated by skipping the middle element like the word "mom", your halves are "m" and "m". - Hint: (without using STL)arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
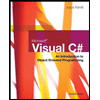
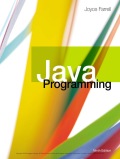