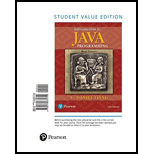
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18.7, Problem 18.7.1CP
Program Plan Intro
Towers of Hanoi:
This is a type of program which is difficult to solve. It gets easier to solve using recursion. The problem includes moving a particular number of disks of different sizes from one tower to another. It has certain rules in moving the disks they are:
- The towers are labeled “A”, “B”, and “C”. The disks are labeled as 1, 2, 3, and so on.
- The bigger disk should not be on the top of smaller disk.
- Initially all the disks are placed on tower A.
- Only one disk can be moved at a time.
The aim of this problem is to move all the disks from tower A to tower B without violating the above rules.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?
When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.
Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.
To elaborate,…
I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…
Male comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze.
Question 19 options:
True
False
In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home?
Question 1 options:
to earn enough money to buy a mink coat
to have something to do while the kids were at school
to pay the bills after her husband got laid off
Chapter 18 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 18.2 - What is a recursive method? What is an infinite...Ch. 18.2 - Prob. 18.2.2CPCh. 18.2 - Show the output of the following programs and...Ch. 18.2 - Prob. 18.2.4CPCh. 18.2 - Prob. 18.2.5CPCh. 18.2 - Write a recursive mathematical definition for...Ch. 18.3 - Prob. 18.3.1CPCh. 18.3 - What is wrong in the following methods?Ch. 18.3 - Prob. 18.3.3CPCh. 18.4 - Describe the characteristics of recursive methods.
Ch. 18.4 - Prob. 18.4.2CPCh. 18.4 - Prob. 18.4.3CPCh. 18.5 - Prob. 18.5.1CPCh. 18.5 - Prob. 18.5.2CPCh. 18.5 - What is a recursive helper method?Ch. 18.6 - Prob. 18.6.1CPCh. 18.6 - How does the program get all files and directories...Ch. 18.6 - How many times will the getSize method be invoked...Ch. 18.6 - Will the program work if the directory is empty...Ch. 18.6 - Will the program work if line 20 is replaced by...Ch. 18.6 - Will the program work if lines 20 and 21 are...Ch. 18.7 - Prob. 18.7.1CPCh. 18.8 - Prob. 18.8.1CPCh. 18.8 - Prob. 18.8.2CPCh. 18.8 - How many times is the displayTriangles method...Ch. 18.8 - Prob. 18.8.4CPCh. 18.8 - Prob. 18.8.5CPCh. 18.9 - Which of the following statements are true? a. Any...Ch. 18.9 - Prob. 18.9.2CPCh. 18.10 - Identify tail-recursive methods in this chapter.Ch. 18.10 - Rewrite the fib method in Listing 18.2 using tail...Ch. 18 - Prob. 18.1PECh. 18 - Prob. 18.2PECh. 18 - (Compute greatest common divisor using recursion)...Ch. 18 - (Sum series) Write a recursive method to compute...Ch. 18 - (Sum series) Write a recursive method to compute...Ch. 18 - (Sum series) Write a recursive method to compute...Ch. 18 - (Fibonacci series) Modify Listing 18.2,...Ch. 18 - Prob. 18.8PECh. 18 - (Print the characters in a string reversely) Write...Ch. 18 - (Occurrences of a specified character in a string)...Ch. 18 - Prob. 18.11PECh. 18 - (Print the characters in a string reversely)...Ch. 18 - (Find the largest number in an array) Write a...Ch. 18 - (Find the number of uppercase letters in a string)...Ch. 18 - Prob. 18.15PECh. 18 - (Find the number of uppercase letters in an array)...Ch. 18 - (Occurrences of a specified character in an array)...Ch. 18 - (Tower of Hanoi) Modify Listing 18.8,...Ch. 18 - Prob. 18.19PECh. 18 - (Display circles) Write a Java program that...Ch. 18 - (Decimal to binary) Write a recursive method that...Ch. 18 - (Decimal to hex) Write a recursive method that...Ch. 18 - (Binary to decimal) Write a recursive method that...Ch. 18 - (Hex to decimal) Write a recursive method that...Ch. 18 - Prob. 18.25PECh. 18 - (Create a maze) Write a program that will find a...Ch. 18 - (Koch snowflake fractal) The text presented the...Ch. 18 - (Nonrecursive directory size) Rewrite Listing...Ch. 18 - (Number of files in a directory) Write a program...Ch. 18 - (Game: Knights Tour) The Knights Tour is an...Ch. 18 - (Game: Knights Tour animation) Write a program for...Ch. 18 - (Game: Eight Queens) The Eight Queens problem is...Ch. 18 - Prob. 18.35PECh. 18 - (Sierpinski triangle) Write a program that lets...Ch. 18 - (Hilbert curve) The Hilbert curve, first described...Ch. 18 - (Recursive tree) Write a program to display a...Ch. 18 - Prob. 18.39PE
Knowledge Booster
Similar questions
- After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forward
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
- What is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forwardConsider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forward
- assume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
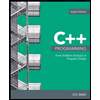
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
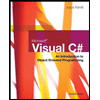
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
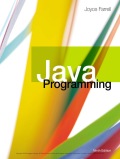
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
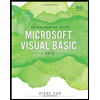
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
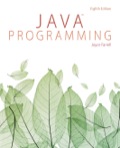
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT