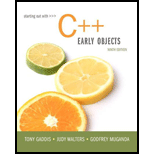
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 9RQE
Program Plan Intro
Queue:
- A queue contains sequence of items.
- The item which is inserted first, will be retrieved first.
- Queue performs “First In First Out”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
When an element is added to a queue, where is it added?
You can access any element on a queue.
True
False
It is a to the next node in the linked list, which is the property after it in the Node class.
Chapter 18 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- You need to implement a class named "Queue" that simulates a basic queue data structure. The class should have the following methods: Enqueue(int value) - adds a new element to the end of the queue Dequeue() - removes the element from the front of the queue and returns it Peek() - returns the element from the front of the queue without removing it Count() - returns the number of elements in the queue The class should also have a property named "IsEmpty" that returns a boolean indicating whether the queue is empty or not. Constraints: The queue should be implemented using an array and the array should automatically resize when needed. All methods and properties should have a time complexity of O(1) You can write the program in C# and use the test cases to check if your implementation is correct. An example of how the class should be used: Queue myQueue = new Queue(); myQueue.Enqueue(1); myQueue.Enqueue(2); myQueue.Enqueue(3); Console.WriteLine(myQueue.Peek()); // 1…arrow_forwardListQueue Node Node Node front= next next= next = nul1 rear = data "Thome" data "Abreu" data - "Jones" size - 3 The above is a queue of a waiting list. The ListQueue has a node (front) to record the address of the front element of a queue. It also has another node (rear) to record the address of the tail element of a queue. 4. How do you push a node with data, "Chu" to the above queue? front.next = new Node("Chu", front); a. b. front = new Node ("Chu", front) rear = new Node("Chu", rear) с. d. = new Node ("Chu", rear.next) rear Describe the reason of your choice. Your answer is (a, b, c, or d) Will the push action take time in 0(1) or 0(n)? Next Page Type here to searcharrow_forwardThe data structure that allows for elements to be added and removed at both ends but not in the middle is called: 1.Double-ended Queue. 2.Priority Queue. 3.Duck. 4.Torque.arrow_forward
- After removing the next element from queue, what is the index of the first element?arrow_forwardA queue and a deque data structure are related concepts. Deque is an acronym meaning "double-ended queue." With a deque, you may insert, remove, or view from either end of the queue, which distinguishes it from the other two. Use arrays to implement a dequearrow_forwardWhen removing a node from a linked list, what are the two steps?arrow_forward
- A data structure called a deque is closely related to a queue. The name deque stands for “double-ended queue.” The difference between the two is that with a deque, you can insert, remove, or view from either end of the queue. Implement a deque using arraysarrow_forwardThe definition of linked list is given as follows: struct Node { ElementType Element ; struct Node *Next ; } ; typedef struct Node *PtrToNode, *List, *Position; If L is head pointer of a linked list, then the data type of L should be ??arrow_forwardListQueue Node Node Node front- next = next next - nul1 data - "Jones" rear data "Thome" data - "Abreu" size = 3 The above is a queue of a waiting list. The ListQueue has a node (front) to record the address of the front element of a queue. It also has another node (rear) to record the address of the tail element of a queue. 3. How do you pop a node from the above queue? a. front.next = front; b. front = front.next с. rear = rear.next d. rear.next = rear Describe the reason of your choice. Your answer is (a, b, c or d) will the pop action take time in 0(1) or 0(n)? Next Page P Type here to searcharrow_forward
- What is the index of the first element in the queue after the next element has been removed from the queue?arrow_forwardc++ Write a client function that returns the back of a queue while leaving the queue unchanged. This function can call any of the methods of the ADT queue. It can also create new queues. The return type is ITemType, and it accepts a queue as a parameter.arrow_forwardModify the given code to make it work using the keywords (undo,redo,display and exit) instead of the case statment. It will insert by default but when it reads one of the keywords it will go the function we call. Example: Enter the element to be inserted in the queue: hello Enter the element to be inserted in the queue: world Enter the element to be inserted in the queue:display hello world Enter the element to be inserted in the queue:undo Enter the element to be inserted in the queue:display hello Enter the element to be inserted in the queue:redo Enter the element to be inserted in the queue:display hello world ***************************** The Code #include <stdio.h>#include <stdlib.h>struct node{char data[100];struct node *link;}*front, *rear; struct node *list=NULL;void insert(){struct node *temp;temp = (struct node*)malloc(sizeof(struct node));printf("Enter the element to be inserted in the queue: ");scanf("%s",temp->data);temp->link = NULL;if (rear ==…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
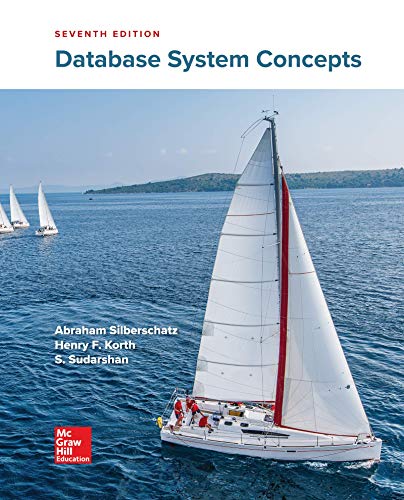
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
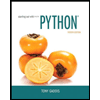
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
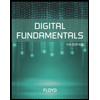
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
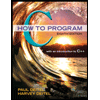
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
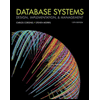
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
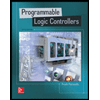
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education