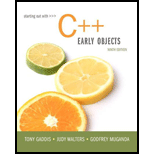
Starting Out with C++: Early Objects (9th Edition)
9th Edition
ISBN: 9780134400242
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 1PC
Program Plan Intro
Static Stack Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “stck” for stack.
- Declare a variable named “popElem”.
- Push 5 elements inside the stack using the function “push_Elem ()”.
- Pop 5 elements from the stack using the function “pop_Elem ()”.
Stack.h:
- Include required header files.
- Create a template.
- Declare a class named “Stack”. Inside the class
- Inside the “private” access specifier,
- Create an object for the template
- Declare the variables “stackSize” and “top_Elem”.
- Inside the “public” access specifier,
- Give a declaration for an overloaded constructor.
- Give function declaration for “push_Elem ()”, “pop_Elem ()”, “is_Full ()”, and “is_Empty ()”.
- Inside the “private” access specifier,
- Give function definition for the overloaded constructor.
- Create stack size
- Assign the value to the “stackSize”.
- Assign -1 to the variable “top_Elem”
- Give function definition for “push_Elem ()”.
- Check if the stack is full using the function “is_Full ()”
- If the condition is true then print “The stack is full”.
- If the condition is not true then,
- Increment the variable “top_Elem”.
- Assign the element to the top position.
- Check if the stack is full using the function “is_Full ()”
- Give function definition for “pop_Elem ()”.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign the element to the variable “num”.
- Decrement the variable “top_Elem”.
- Check if the stack is empty using the function “is_Empty ()”
- Give function definition for “is_Full ()”.
- Assign Boolean value to the variable
- Check if the top and the stack size is same
- Assign true to “status”.
- Return the status
- Give function definition for “is_Empty ()”.
- Assign Boolean value to the variable
- Check if the top is equal to -1.
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ Question
You need to write a class called LinkedList that implements the following List operations:
public void add(int index, Object item);
// adds an item to the list at the given index, that index may be at start, end or after or before the
// specific element
2.public void remove(int index);
// removes the item from the list that has the given index
3.public void remove(Object item);
// finds the item from list and removes that item from the list
4.public List duplicate();
// creates a duplicate of the list
// postcondition: returns a copy of the linked list
5.public List duplicateReversed();
// creates a duplicate of the list with the nodes in reverse order
// postcondition: returns a copy of the linked list with the nodes in
6.public List ReverseDisplay();
//print list in reverse order
7.public Delete_Smallest();
// Delete smallest element from linked list
8.public List Delete_duplicate();
// Delete duplicate elements from a given linked list.Retain the…
C++ Question
You need to write a class called LinkedList that implements the following List operations:
public void add(int index, Object item);
// adds an item to the list at the given index, that index may be at start, end or after or before the
// specific element
2.public void remove(int index);
// removes the item from the list that has the given index
3.public void remove(Object item);
// finds the item from list and removes that item from the list
4.public List duplicate();
// creates a duplicate of the list
// postcondition: returns a copy of the linked list
5.public List duplicateReversed();
// creates a duplicate of the list with the nodes in reverse order
// postcondition: returns a copy of the linked list with the nodes in
6.public List ReverseDisplay();
//print list in reverse order
7.public Delete_Smallest();
// Delete smallest element from linked list
8.public List Delete_duplicate();
// Delete duplicate elements from a given linked list.Retain the…
Briefly describe the stack parameter.
Chapter 18 Solutions
Starting Out with C++: Early Objects (9th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 14PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- C++ ProgrammingActivity: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "queue.h" #include "linkedlist.h" class SLLQueue : public Queue { LinkedList* list; public: SLLQueue() { list = new LinkedList(); } void enqueue(int e) { list->addTail(e); return; } int dequeue() { int elem; elem = list->removeHead(); return elem; } int first() { int elem; elem = list->get(1); return elem;; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } int collect(int max) { int sum = 0; while(first() != 0) { if(sum + first() <= max) { sum += first(); dequeue(); } else {…arrow_forwardC++ ProgrammingActivity: Deque Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "deque.h" #include "linkedlist.h" #include <iostream> using namespace std; class DLLDeque : public Deque { DoublyLinkedList* list; public: DLLDeque() { list = new DoublyLinkedList(); } void addFirst(int e) { list->addAt(e,1); } void addLast(int e) { list->addAt(e,size()+1); } int removeFirst() { return list->removeAt(1); } int removeLast() { return list->removeAt(size()); } int size(){ return list->size(); } bool isEmpty() { return list->isEmpty(); } // OPTIONAL: a helper method to help you debug void print() {…arrow_forwardData Structure & Algorithum java program Do the following: 1) Add a constructor to the class "LList" that creates a list from a given array of objects.2) Add a method "addAll" to the "LList" class that adds an array of items to the end of the list. The header of the method is as follows, where "T" is the generic type of the objects in the list. 3) Write a Test/Driver program that thoroughly tests all the methods in the class "LList".arrow_forward
- In c++ Also add comments explaining each linearrow_forwardC++ ProgrammingActivity: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM #include "queue.h" #include "linkedlist.h" class SLLQueue : public Queue { LinkedList* list; public: SLLQueue() { list = new LinkedList(); } void enqueue(int e) { list->addTail(e); return; } int dequeue() { int elem; elem = list->removeHead(); return elem; } int first() { int elem; elem = list->get(1); return elem;; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } int collect(int max) { int sum = 0; while(first() != 0) { if(sum + first() <= max) { sum += first();…arrow_forwardComputer Science //iterator() creates a new Iterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end". template <typename ValueType>typename DoublyLinkedList<ValueType>::Iterator DoublyLinkedList<ValueType>::iterator(){//return iterator(head);} //constIterator() creates a new ConstIterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end". template <typename ValueType>typename DoublyLinkedList<ValueType>::ConstIterator DoublyLinkedList<ValueType>::constIterator() const{//return constIterator(head);} //Initializes a newly-constructed IteratorBase to operate on//the given list. It will initially be referring to the first//value in the list, unless the list is empty, in which case//it will be…arrow_forward
- Read this: Complete the code in Visual Studio using C++ Programming Language - Give me the answer in Visual Studio so I can copy*** - Separate the files such as .cpp, .h, or .main if any! Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character string operator [] Treat like…arrow_forwardRead this: Complete the code in Visual Studio using C++ Programming Language with 1 file or clear answer!! - Give me the answer in Visual Studio so I can copy*** - Separate the files such as .cpp, .h, or .main if any! Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character…arrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. So using the class made in task 1, make a class named as Stack, having following additional functionalities: bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy…arrow_forward
- C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS int main(int argc, char** argv) { SLLStack* stack = new SLLStack(); int test; int length; string str; char top; bool flag = true; cin >> test; switch (test) { case 0: getline(cin, str); length = str.length(); for(int i = 0; i < length; i++){ if(str[i] == '{' || str[i] == '(' || str[i] == '['){ stack->push(str[i]); } else if (str[i] == '}' || str[i] == ')' || str[i] == ']'){ if(!stack->isEmpty()){ top = stack->top(); if(top == '{' && str[i] == '}' || top == '(' && str[i] == ')' ||…arrow_forwardStack:#ifndef STACKTYPE_H_INCLUDED#define STACKTYPE_H_INCLUDEDconst int MAX_ITEMS = 5;class FullStack// Exception class thrown// by Push when stack is full.{};class EmptyStack// Exception class thrown// by Pop and Top when stack is emtpy.{};template <class ItemType>class StackType{public:StackType();bool IsFull();bool IsEmpty();void Push(ItemType);void Pop();ItemType Top();private:int top;ItemType items[MAX_ITEMS];};#endif // STACKTYPE_H_INCLUDED Queue:#ifndef QUETYPE_H_INCLUDED#define QUETYPE_H_INCLUDEDtemplate<class T>class QueType{public:QueType();QueType(int);~QueType();void MakeEmpty();bool IsEmpty();bool IsFull();void Enqueue(T);void Dequeue(T&);T Front();private:int front;int rear;T *info;int maxQue;};#endif // QUETYPE_H_INCLUDEDarrow_forwarddata structure-JAVAarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
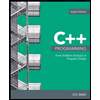
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning