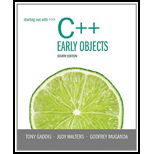
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 7PC
Program Plan Intro
Queue Exceptions
“main.cpp”:
- This program features queues that can throw exceptions and print an appropriate error message and should terminate the program.
- Include the required header files.
- Prompt the user to enqueue 5 items.
- Use of try – catch exceptional handling that returns error message and terminates the program if the queue overflows.
- Prompt the user and allow user option to overflow the queue and dequeue the queue and print the values present in the queue.
“IntQueue.h”:
- Include all the required header files.
- Declare all the variables present in the queue along with their data types.
- Declare all the function definition.
- Declare the class underflow and overflow to check for exceptional handling in queue.
“IntQueue.cpp”:
- Include all the required header files.
- Call a constructor to create a queue and initialize its elements.
- Initialize front and rear each to -1 and the number of elements initially present to 0.
- A destructor is called to delete the queue array.
- Define a queue function enqueue that inserts the value in variable num at the rear of the queue.
- Define a queue function dequeue that deletes the value at the front of the queue, and copies it into variable.
- Define a queue function isEmpty returns true if the queue is empty, and false if elements are present in the queue.
- Define a Queue function is Full return true if queue is full and false if queue is not full.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.
11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources.
Use Solver to find this information as follows:
a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell.
b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells.
c. Determine and enter the constraints based on the information provided in Table 3.
d. Use Simplex LP as the solving method to find a global optimal solution.
e. Save the Solver model below the Maximum weekly profit model label.
f. Solve the model, keeping the Solver solution.
Table 3: Solver Constraints
Constraint Cell or Range
Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5
Each Tuesday and…
Go to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows:
Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell.
Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells.
Determine and enter the constraints based on the information provided in Table 3.
Use Simplex LP as the solving method to find a global optimal solution.
Save the Solver model below the Maximum weekly profit model label.
Solve the model, keeping the Solver solution.
Table 3: Solver Constraints
Constraint
Cell or Range
Each webinar is scheduled at least once on Tuesday and once on Thursday
B4:F5
Each Tuesday and Thursday…
Chapter 18 Solutions
Starting Out with C++: Early Objects
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PC
Knowledge Booster
Similar questions
- I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forwardMale comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze. Question 19 options: True False In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home? Question 1 options: to earn enough money to buy a mink coat to have something to do while the kids were at school to pay the bills after her husband got laid offarrow_forward
- After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forward
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
- What is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forwardConsider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
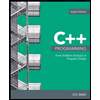
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
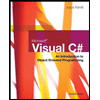
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
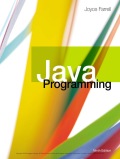
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
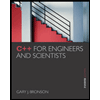
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
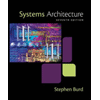
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning