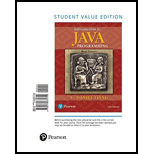
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
11th Edition
ISBN: 9780134671710
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 17.6, Problem 17.6.2CP
Program Plan Intro
Serializable objects:
To serialize an object it needs to convert its state to a byte stream. After converting it into the byte stream it can be reverted back into a copy of the object. To serialize a java object, its class or any superclass should implement “java.io.serializable”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's
cssProperty value, and return the new "px" value.
Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px =
"200px".
SHOW EXPECTED
HTML JavaScript
1 function addPixels (element, cssProperty, pixelAmount) {
2
3
/* Your solution goes here *1
4 }
5
6 const helloElem = document.querySelector("# helloMessage");
7 const newVal = addPixels (helloElem, "width", 50);
8 helloElem.style.setProperty("width", newVal);
[
Solve in MATLAB
Hello please look at the attached picture. I need an detailed explanation of the architecture
Chapter 17 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Ch. 17.2 - What is a text file and what is a binary file? Can...Ch. 17.2 - How do you read or write text data in Java? What...Ch. 17.3 - Prob. 17.3.1CPCh. 17.3 - How is a Java character represented in the memory,...Ch. 17.3 - If you write the string ABC to an ASCII text file,...Ch. 17.3 - If you write the string 100 to an ASCII text file,...Ch. 17.3 - What is the encoding scheme for representing a...Ch. 17.4 - Prob. 17.4.1CPCh. 17.4 - Why should you always close streams? How do you...Ch. 17.4 - Prob. 17.4.3CP
Ch. 17.4 - Does FileInputStream/Fi1eOutputStream introduce...Ch. 17.4 - What will happen if you attempt to create an input...Ch. 17.4 - How do you append data to an existing text file...Ch. 17.4 - Prob. 17.4.7CPCh. 17.4 - What is written to a file using writeByte(91) on a...Ch. 17.4 - How do you check the end of a file in an input...Ch. 17.4 - What is wrong in the following code? Import...Ch. 17.4 - Suppose you run the following program on Windows...Ch. 17.4 - After the following program is finished, how many...Ch. 17.4 - For each of the following statements on a...Ch. 17.4 - What are the advantages of using buffered streams?...Ch. 17.5 - How does the program check if a file already...Ch. 17.5 - How does the program detect the end of the file...Ch. 17.5 - How does the program count the number of bytes...Ch. 17.6 - Prob. 17.6.1CPCh. 17.6 - Prob. 17.6.2CPCh. 17.6 - Is it true that any instance of...Ch. 17.6 - Prob. 17.6.4CPCh. 17.6 - Prob. 17.6.5CPCh. 17.6 - What will happen when you attempt to run the...Ch. 17.7 - Can RandomAccessFi1e streams read and write a data...Ch. 17.7 - Create a RandomAccessFi1e stream for the file...Ch. 17.7 - What happens if the file test.dat does not exist...Ch. 17 - (Create a text file) Write a program to create a...Ch. 17 - (Create a binary data file) Write a program to...Ch. 17 - (Sum all the integers in a binary data file)...Ch. 17 - (Convert a text file into UTF) Write a program...Ch. 17 - Prob. 17.5PECh. 17 - Prob. 17.6PECh. 17 - Prob. 17.7PECh. 17 - (Update count) Suppose that you wish to track how...Ch. 17 - (Address book) Write a program that stores,...Ch. 17 - (Split files) Suppose you want to back up a huge...Ch. 17 - (Split files GUI) Rewrite Exercise 17.10 with a...Ch. 17 - Prob. 17.12PECh. 17 - (Combine files GUI) Rewrite Exercise 17.12 with a...Ch. 17 - (Encrypt files) Encode the file by adding 5 to...Ch. 17 - (Decrypt files) Suppose a file is encrypted using...Ch. 17 - (Frequency of characters) Write a program that...Ch. 17 - (BitOutputStream) Implement a class named...Ch. 17 - (View bits) Write the following method that...Ch. 17 - (View hex) Write a program that prompts the user...Ch. 17 - (Hex editor) Write a GUI application that lets the...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
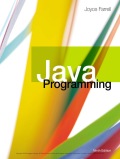
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
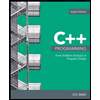
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
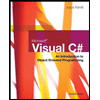
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,